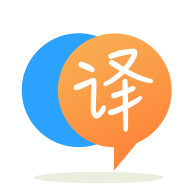
[英]How to open a remote file with GDAL in Python through a Flask application
[英]How to Open a file through python
我对编程和 python 语言很陌生。
我知道如何在 python 中打开文件,但问题是如何将文件作为函数的参数打开?
例子:
function(parameter)
这是我写出代码的方式:
def function(file):
with open('file.txt', 'r') as f:
contents = f.readlines()
lines = []
for line in f:
lines.append(line)
print(contents)
您可以轻松传递文件对象。
with open('file.txt', 'r') as f: #open the file
contents = function(f) #put the lines to a variable.
并在您的函数中,返回行列表
def function(file):
lines = []
for line in f:
lines.append(line)
return lines
另一个技巧,python文件对象实际上有一个方法来读取文件的行。 像这样:
with open('file.txt', 'r') as f: #open the file
contents = f.readlines() #put the lines to a variable (list).
使用第二种方法, readlines
就像你的函数。 您不必再次调用它。
更新以下是您应该如何编写代码:
第一种方法:
def function(file):
lines = []
for line in f:
lines.append(line)
return lines
with open('file.txt', 'r') as f: #open the file
contents = function(f) #put the lines to a variable (list).
print(contents)
第二个:
with open('file.txt', 'r') as f: #open the file
contents = f.readlines() #put the lines to a variable (list).
print(contents)
希望这可以帮助!
Python 允许将多个 open() 语句放在一个 with 中。 你用逗号分隔它们。 您的代码将是:
def filter(txt, oldfile, newfile):
'''\
Read a list of names from a file line by line into an output file.
If a line begins with a particular name, insert a string of text
after the name before appending the line to the output file.
'''
with open(newfile, 'w') as outfile, open(oldfile, 'r', encoding='utf-8') as infile:
for line in infile:
if line.startswith(txt):
line = line[0:len(txt)] + ' - Truly a great person!\n'
outfile.write(line)
# input the name you want to check against
text = input('Please enter the name of a great person: ')
letsgo = filter(text,'Spanish', 'Spanish2')
不,通过在函数末尾放置显式返回,您不会获得任何收益。 您可以使用 return 提前退出,但您在最后拥有它,并且该函数将在没有它的情况下退出。 (当然,对于返回值的函数,您可以使用 return 来指定要返回的值。)
def fun(file):
contents = None
with open(file, 'r') as fp:
contents = fp.readlines()
## if you want to eliminate all blank lines uncomment the next line
#contents = [line for line in ''.join(contents).splitlines() if line]
return contents
print fun('test_file.txt')
或者你甚至可以修改它,这样它也可以将文件对象作为函数参数
这是一种更简单的打开文件的方法,无需在 Python 3.4 中定义自己的函数:
var=open("A_blank_text_document_you_created","type_of_file")
var.write("what you want to write")
print (var.read()) #this outputs the file contents
var.close() #closing the file
以下是文件类型:
"r"
: 只是为了读取文件
"w"
: 只是写一个文件
"r+"
: 一种允许读写文件的特殊类型
有关更多信息,请参阅此备忘单。
def main():
file=open("chirag.txt","r")
for n in file:
print (n.strip("t"))
file.close()
if __name__== "__main__":
main()
the other method is
with open("chirag.txt","r") as f:
for n in f:
print(n)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.