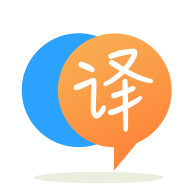
[英]Why does this not work when trying to find the sum of all multiples of 3 and 5 in 1000?
[英]find the sum of the multiples of 3 and 5 below 1000
好的,伙计们,所以我正在做欧拉计划挑战,我不敢相信我被困在第一个挑战上。 尽管我的代码看起来很实用,但我真的不明白为什么我得到了错误的答案:
import java.util.ArrayList;
public class Multithree {
public static void main(String[] args) {
// TODO Auto-generated method stub
ArrayList<Integer> x = new ArrayList<Integer>();
ArrayList<Integer> y = new ArrayList<Integer>();
int totalforthree = 0;
int totalforfive = 0;
int total =0;
for(int temp =0; temp < 1000 ; temp++){
if(temp % 3 == 0){
x.add(temp);
totalforthree += temp;
}
}
for(int temp =0; temp < 1000 ; temp++){
if(temp % 5 == 0){
y.add(temp);
totalforfive += temp;
}
}
total = totalforfive + totalforthree;
System.out.println("The multiples of 3 or 5 up to 1000 are: " +total);
}
}
我得到的答案是 266333,它说这是错误的......
您应该对两者使用相同的 for 循环以避免重复计算两者的倍数。 比如 15,30...
for(int temp =0; temp < 1000 ; temp++){
if(temp % 3 == 0){
x.add(temp);
totalforthree += temp;
}else if(temp % 5 == 0){
y.add(temp);
totalforfive += temp;
}
}
从数学的角度来看,
您没有考虑 3 和 5 之间的公因数。
因为有重复计算。
例如; 数 15 , 30 , 45 , 60 , 75 , 90 , 105 , 120 , 135 , 150 , 165 , 180 , 195 , 210 , 225 , 240 , 3 5, 3, 5, 3, 5, 3, 5, 3, 5, 3, 5, 3, 5, 3, 5, 3, 5, 255, 3, 5, 3, 0 ,390,405,420,435,450,465,480,495,510,525,540,555,570,585,600,615,630,645,660,675,690,705,720,735,750 、 765 、 780 、 795 、 810 、 825 、 840 、 855 、 870 、 885 、 900 、 915 、 930 、 945 、 960 、 975 、 90 是公因数。
公因数的总和 = 33165。
你的答案是 266333
正确答案是 233168。
你的答案 - 公因数总和
266333-33165=233168。
(这是获取公因数和公因数总和的代码)
public static void main(String[] args) {
System.out.println("The sum of the Common Factors : " + getCommonFactorSum());
}
private static int getCommonFactorSum() {
int sum = 0;
for (int i = 1; i < 1000; i++) {
if (i % 3 == 0 && i % 5 == 0) {
sum += i;
System.out.println(i);
}
}
难道你们都认为不是使用循环来计算倍数的总和,我们可以使用简单的算术级数公式轻松计算 n 项的总和来计算 n 项的总和。
我使用循环和公式评估了结果。 循环对短数据范围非常有用。 但是当数据范围增长超过 10 10程序需要几个小时来处理循环的结果。 但是当使用简单的算术级数公式时,同样以毫秒为单位评估结果。
我们真正需要做的是:
算法:
这是我的博客文章CodeForWin - Project Euler 1: Multiples of 3 and 5中的 Java 代码片段
n--; //Since we need to compute the sum less than n.
//Check if n is more than or equal to 3 then compute sum of all divisible by
//3 and add to sum.
if(n>=3) {
totalElements = n/3;
sum += (totalElements * ( 3 + totalElements*3)) / 2;
}
//Check if n is more than or equal to 5 then compute sum of all elements
//divisible by 5 and add to sum.
if(n >= 5) {
totalElements = n/5;
sum += (totalElements * (5 + totalElements * 5)) / 2;
}
//Check if n is more than or equal to 15 then compute sum of all elements
//divisible by 15 and subtract from sum.
if(n >= 15) {
totalElements = n/15;
sum -= (totalElements * (15 + totalElements * 15)) / 2;
}
System.out.println(sum);
如果您使用的是 Java 8,则可以通过以下方式进行:
Integer sum = IntStream.range(1, 1000) // create range
.filter(i -> i % 3 == 0 || i % 5 == 0) // filter out
.sum(); // output: 233168
要计算可被3
和5
整除的数字两次,您可以将上述行写两次或.map()
2 * i
值:
Integer sum = IntStream.range(1, 1000)
.filter(i -> i % 3 == 0 || i % 5 == 0)
.map(i -> i % 3 == 0 && i % 5 == 0 ? 2 * i : i)
.sum(); // output: 266333
我如何解决这个问题是我取了一个整数值(初始化为零)并继续添加 i 的增量值,如果它的模数 3 或 5 给我零。
private static int getSum() {
int sum = 0;
for (int i = 1; i < 1000; i++) {
if (i % 3 == 0 || i % 5 == 0) {
sum += i;
}
}
return sum;
}
我这样做了好几次。 完成 Java 所需代码的最快、最干净和最简单的方法是:
public class MultiplesOf3And5 {
public static void main(String[] args){
System.out.println("The sum of the multiples of 3 and 5 is: " + getSum());
}
private static int getSum() {
int sum = 0;
for (int i = 1; i < 1000; i++) {
if (i % 3 == 0 || i % 5 == 0) {
sum += i;
}
}
return sum;
}
如果有人建议减少代码行数,请告诉我您的解决方案。 我是编程新手。
int count = 0;
for (int i = 1; i <= 1000 / 3; i++)
{
count = count + (i * 3);
if (i < 1000 / 5 && !(i % 3 == 0))
{
count = count + (i * 5);
}
}
其他人已经指出了您代码中的错误,但是我想补充一点,模数运算符解决方案并不是最有效的.
更快的实现将是这样的:
int multiply3_5(int max)
{
int i, x3 = 0, x5 = 0, x15 = 0;
for(i = 3; i < max; i+=3) x3 += i; // Store all multiples of 3
for(i = 5; i < max; i+=5) x5 += i; // Store all multiples of 5
for(i = 15; i < max; i+=15) x15 += i; // Store all multiples 15;
return x3+x5-x15;
}
在这个解决方案中,我不得不取出 15 的倍数,因为 3 和 5 有 15 的倍数,所以在第二个循环中它会添加已经在第一个循环中添加的 15 的倍数;
时间复杂度为O(1)
解决方案
另一个更好的解决方案(时间复杂度为O(1)
)是如果您采用数学方法。
您正在尝试对所有数字求和 3 + 6 + 9 ... 1000 和 5 + 10 + 15 +20 + ... 1000 这与 3 * (1 + 2 + 3 + 4 + ... + 333) 和 5 * ( 1 + 2 + 3 + 4 + ... + 200),'n' 个自然数的总和是 (n * (n + 1)) (source ) 所以你可以在一个常数时间内计算,如下:
int multiply3_5(int max)
{
int x3 = (max - 1) / 3;
int x5 = (max - 1) / 5;
int x15 = (max - 1) / 15;
int sn3 = (x3 * (x3 + 1)) / 2;
int sn5 = (x5 * (x5 + 1)) / 2;
int sn15 = (x15 * (x15 + 1)) / 2;
return (3*sn3) + (5 *sn5) - (15*sn15);
}
运行示例:
public class SumMultiples2And5 {
public static int multiply3_5_complexityN(int max){
int i, x3 = 0, x5 = 0, x15 = 0;
for(i = 3; i < max; i+=3) x3 += i; // Store all multiples of 3
for(i = 5; i < max; i+=5) x5 += i; // Store all multiples of 5
for(i = 15; i < max; i+=15) x15 += i; // Store all multiples 15;
return x3 + x5 - x15;
}
public static int multiply3_5_constant(int max){
int x3 = (max - 1) / 3;
int x5 = (max - 1) / 5;
int x15 = (max - 1) / 15;
int sn3 = (x3 * (x3 + 1)) / 2;
int sn5 = (x5 * (x5 + 1)) / 2;
int sn15 = (x15 * (x15 + 1)) / 2;
return (3*sn3) + (5 *sn5) - (15*sn15);
}
public static void main(String[] args) {
System.out.println(multiply3_5_complexityN(1000));
System.out.println(multiply3_5_constant(1000));
}
}
输出:
233168
233168
简直了
public class Main
{
public static void main(String[] args) {
int sum=0;
for(int i=1;i<1000;i++){
if(i%3==0 || i%5==0){
sum+=i;
}
}
System.out.println(sum);
}
}
您正在计算一些数字两次。 您需要做的是在一个 for 循环中添加,并使用 if-else 语句,如果您找到 3 的倍数,则也不会将它们计入 5。
if(temp % 3 == 0){
x.add(temp);
totalforthree += temp;
} else if(temp % 5 == 0){
y.add(temp);
totalforfive += temp;
}
上面给出的逻辑显示错误答案,因为计算时采用 3 和 5 的倍数。 在上面的逻辑中遗漏了一些东西,即 15、30、45、60……是 3 的倍数,也是 5 的倍数。那么我们在添加时需要忽略这些。
public static void main(String[] args) {
int Sum=0, i=0, j=0;
for(i=0;i<=1000;i++)
if (i%3==0 && i<=999)
Sum=Sum+i;
for(j=0;j<=1000;j++)
if (j%5==0 && j<1000 && j*5%3!=0)
Sum=Sum+j;
System.out.println("The Sum is "+Sum);
}
好的,所以这不是最好看的代码,但它完成了工作。
public class Multiples {
public static void main(String[]args) {
int firstNumber = 3;
int secondNumber = 5;
ArrayList<Integer> numberToCheck = new ArrayList<Integer>();
ArrayList<Integer> multiples = new ArrayList<Integer>();
int sumOfMultiples = 0;
for (int i = 0; i < 1000; i++) {
numberToCheck.add(i);
if (numberToCheck.get(i) % firstNumber == 0 || numberToCheck.get(i) % secondNumber == 0) {
multiples.add(numberToCheck.get(i));
}
}
for (int i=0; i<multiples.size(); i++) {
sumOfMultiples += multiples.get(i);
}
System.out.println(multiples);
System.out.println("Sum Of Multiples: " + sumOfMultiples);
}
}
如果数字是 10,那么 3 的倍数是 3,6,9,5 的倍数是 5,10 总和是 33,程序给出相同的答案:
package com.parag;
/*
* @author Parag Satav
*/
public class MultipleAddition {
/**
* @param args
*/
public static void main( final String[] args ) {
// TODO Auto-generated method stub
ArrayList<Integer> x = new ArrayList<Integer>();
ArrayList<Integer> y = new ArrayList<Integer>();
int totalforthree = 0;
int totalforfive = 0;
int number = 8;
int total = 0;
for ( int temp = 1; temp <= number; temp++ ) {
if ( temp % 3 == 0 ) {
x.add( temp );
totalforthree += temp;
}
else if ( temp % 5 == 0 ) {
y.add( temp );
totalforfive += temp;
}
}
total = totalforfive + totalforthree;
System.out.println( "multiples of 3 : " + x );
System.out.println( "multiples of 5 : " + y );
System.out.println( "The multiples of 3 or 5 up to " + number + " are: " + total );
}
}
public class Solution {
public static void main(String[] args) {
/* Enter your code here. Read input from STDIN. Print output to STDOUT. Your class should be named Solution. */
Scanner sc = new Scanner(System.in);
int t = sc.nextInt();
while (t>0){
int sum = 0;
int count =0;
int n = sc.nextInt();
n--;
System.out.println((n/3*(6+(n/3-1)*3))/2 + (n/5*(10+(n/5-1)*5))/2 - (n/15*(30+(n/15-1)*15))/2);
t--;
}
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.