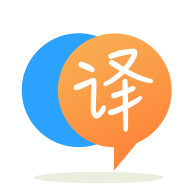
[英]How to restrict user to set date based on other date from date picker in android
[英]How to set date on date picker based on other date picker in android
我使用下面的代码来生成运行时日期选择器。
public class MultiDatePickerActivity extends Activity {
private TextView startDateDisplay;
private TextView endDateDisplay;
private Button startPickDate;
private Button endPickDate;
private Calendar startDate;
private Calendar endDate;
static final int DATE_DIALOG_ID = 0;
private TextView activeDateDisplay;
private Calendar activeDate;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.multidatepicker);
/* capture our View elements for the start date function */
startDateDisplay = (TextView) findViewById(R.id.startDateDisplay);
startPickDate = (Button) findViewById(R.id.startPickDate);
/* get the current date */
startDate = Calendar.getInstance();
/* add a click listener to the button */
startPickDate.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
showDateDialog(startDateDisplay, startDate);
}
});
/* capture our View elements for the end date function */
endDateDisplay = (TextView) findViewById(R.id.endDateDisplay);
endPickDate = (Button) findViewById(R.id.endPickDate);
/* get the current date */
endDate = Calendar.getInstance();
/* add a click listener to the button */
endPickDate.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
showDateDialog(endDateDisplay, endDate);
}
});
/* display the current date (this method is below) */
updateDisplay(startDateDisplay, startDate);
updateDisplay(endDateDisplay, endDate);
}
private void updateDisplay(TextView dateDisplay, Calendar date) {
dateDisplay.setText(
new StringBuilder()
// Month is 0 based so add 1
.append(date.get(Calendar.MONTH) + 1).append("-")
.append(date.get(Calendar.DAY_OF_MONTH)).append("-")
.append(date.get(Calendar.YEAR)).append(" "));
}
public void showDateDialog(TextView dateDisplay, Calendar date) {
activeDateDisplay = dateDisplay;
activeDate = date;
showDialog(DATE_DIALOG_ID);
}
private OnDateSetListener dateSetListener = new OnDateSetListener() {
@Override
public void onDateSet(DatePicker view, int year, int monthOfYear, int dayOfMonth) {
activeDate.set(Calendar.YEAR, year);
activeDate.set(Calendar.MONTH, monthOfYear);
activeDate.set(Calendar.DAY_OF_MONTH, dayOfMonth);
updateDisplay(activeDateDisplay, activeDate);
unregisterDateDisplay();
}
};
private void unregisterDateDisplay() {
activeDateDisplay = null;
activeDate = null;
}
@Override
protected Dialog onCreateDialog(int id) {
switch (id) {
case DATE_DIALOG_ID:
return new DatePickerDialog(this, dateSetListener, activeDate.get(Calendar.YEAR), activeDate.get(Calendar.MONTH), activeDate.get(Calendar.DAY_OF_MONTH));
}
return null;
}
@Override
protected void onPrepareDialog(int id, Dialog dialog) {
super.onPrepareDialog(id, dialog);
switch (id) {
case DATE_DIALOG_ID:
((DatePickerDialog) dialog).updateDate(activeDate.get(Calendar.YEAR), activeDate.get(Calendar.MONTH), activeDate.get(Calendar.DAY_OF_MONTH));
break;
}
}
}
我想按endDate时不应该显示当前日期,我希望它检查在startDate中选择的日期,并且应该在endDate选择器中显示startDate的下一个日期。
这意味着我想基于startDate(开始日期的下一个日期)设置endDate。 假设我今天是2013年12月5日,如果我选择2013年5月5日作为开始日期,那么它应该在endDate选择器中显示16-05-2013。 所以我怎么能做到这一点。
请帮我。 任何帮助,将不胜感激。 提前致谢。
日历具有添加方法。 从文档中:
将给定金额添加到“日历”字段。
要添加1天,您可以执行以下操作:
yourDestinationCalendar.add(Calendar.DATE, 1);
将yourDestinationCalendar
初始化为与填充小部件所用的日历相同的方式
您可以在DatePickerDialog
设置最小值和最大值。
从第一个对话框(startDate)获取日期,并在当天添加一天,如下面的代码所示。
Calendar mCalendarTo = Calendar.getInstance();
mCalendarTo.set(year_value, month_value, day_value);
mCalendarTo.add(Calendar.DATE, 1);
将最小值设置为DatePickerDialog
(endDate)
mDatePickerDialog.getDatePicker().setMinDate(mCalendarTo.getTimeInMillis());
如果我正确理解了您的问题,我认为您应该在OnDateSetListener
为开始日期选择器执行此操作。
使用onDateSet
建议的代码向传递给onDateSet
方法的日期添加一天,并在updateDisplay
方法中为endDatePicker使用此新Calendar实例。
这是一个可以适应您的代码的简单示例
Button startDateButton = (Button)findViewById(R.id.start_date_button);
Button endDateButton = (Button)findViewById(R.id.end_date_button);
Calendar cal = Calendar.getInstance();
_endDateListener = new DatePickerDialog.OnDateSetListener() {
@Override
public void onDateSet(DatePicker view, int year, int monthOfYear, int dayOfMonth) {
//Do what ever you need to do in the end date listener
}
};
_startDateListener = new DatePickerDialog.OnDateSetListener() {
@Override
public void onDateSet(DatePicker view, int year, int monthOfYear, int dayOfMonth) {
Calendar cal = Calendar.getInstance();
cal.set(year, monthOfYear, dayOfMonth);
cal.add(Calendar.DATE, 1);
_endDateDialog = new DatePickerDialog(_context, _endDateListener, cal.get(Calendar.YEAR), cal.get(Calendar.MONTH), cal.get(Calendar.DAY_OF_MONTH));
}
};
_startDateDialog = new DatePickerDialog(this, _startDateListener, cal.get(Calendar.YEAR), cal.get(Calendar.MONTH), cal.get(Calendar.DAY_OF_MONTH));
_endDateDialog = new DatePickerDialog(this, _endDateListener, cal.get(Calendar.YEAR), cal.get(Calendar.MONTH), cal.get(Calendar.DAY_OF_MONTH));
startDateButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
_startDateDialog.show();
}
});
endDateButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
_endDateDialog.show();
}
});
希望这已经为您澄清了
在这种情况下,结束日期将是用户选择的开始日期之后的1天。 希望这可以帮助.. :)
public class MainActivity extends AppCompatActivity {
Button StartDate, EndDate;
int year_x, month_x, day_x;
int year_y, month_y, day_y;
static final int DIALOG_ID_1 = 0;
static final int DIALOG_ID_2 = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
final Calendar calendar = Calendar.getInstance();
year_x = calendar.get(Calendar.YEAR);
month_x = calendar.get(Calendar.MONTH);
day_x = calendar.get(Calendar.DAY_OF_MONTH);
year_y = year_x;
month_y = month_x;
day_y = day_x + 1;
showDialogOnButtonClick();
}
public void showDialogOnButtonClick() {
StartDate = (Button) findViewById(R.id.bt_startDate);
StartDate.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
showDialog(DIALOG_ID_1);
}
});
EndDate = (Button) findViewById(R.id.bt_EndDate);
EndDate.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
showDialog(DIALOG_ID_2);
}
});
}
@Override
protected Dialog onCreateDialog(int id) {
if (id == DIALOG_ID_1) {
return new DatePickerDialog(this, dpickerlistener, year_x, month_x, day_x);
} else if (id == DIALOG_ID_2)
return new DatePickerDialog(this, dpickerlistener2, year_y, month_y, day_y);
else return null;
}
private DatePickerDialog.OnDateSetListener dpickerlistener = new DatePickerDialog.OnDateSetListener() {
@Override
public void onDateSet(DatePicker view, int year, int monthOfYear, int dayOfMonth) {
year_x = year;
month_x = monthOfYear + 1;
day_x = dayOfMonth;
Toast.makeText(MainActivity.this, "Start Date : " + day_x + " / " + month_x + " / " + year_x, Toast.LENGTH_SHORT).show();
}
};
private DatePickerDialog.OnDateSetListener dpickerlistener2 = new DatePickerDialog.OnDateSetListener() {
@Override
public void onDateSet(DatePicker view, int year, int monthOfYear, int dayOfMonth) {
year_y = year;
month_y = monthOfYear + 1;
day_y = dayOfMonth;
Toast.makeText(MainActivity.this, "End Date : " + day_y + " / " + month_y + " / " + year_y, Toast.LENGTH_SHORT).show();
}
};
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.