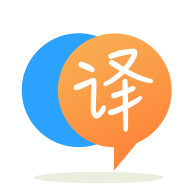
[英]How to select all related data from two tables having one-to-many relationship using Entity Framework?
[英]Insert data simultaneously in entity having one to many relationship
我有两个实体:“学生”和“注册”具有一对多关系。 实体看起来像
public class Student{
[Key]
public int Studentid {get; set;}
public string fname{get; set;}
public string lname{get; set;}
public virtual ICollection<Enrollement> Enrollement_E{ get; set; }
}
public class Enrollement{
[Key]
public int EnrolId {get; set;}
[ForeignKey("Studentid ")]
public int Student{get; set;}
public string kk{get; set;}
public virtual Student Student_E { get; set; }
}
Studentid是自动递增的主键,在注册表中用作外键。 我正在尝试将数据插入两个表中。 如果其中一个失败,则两个插入都不应提交。 因此,我有以下代码。
try{
repository.stu.insert(new student{fname = "fname", lname="lname"});
repository.enr.insert(new Enrollement{student=???, kk="test"});
}
finally{
repository.save();
}
如果尚未保存数据,我应该如何将外键传递给注册记录。 还是有另一种方法?
代替分配外键,而是分配整个对象:
try{
var student = new student(){fname = "fname", lname="lname"};
repository.stu.insert(student);
repository.enr.insert(new Enrollement(){Student_E = student});
}
finally{
repository.save();
}
这样,当student
获得新密钥时,它将自动保存到注册中。
您不需要分别保存这两个对象,只需在保存调用中即可实现
public class Student{
[Key]
public int Studentid {get; set;}
public string fname{get; set;}
public string lname{get; set;}
public virtual ICollection<Enrollement> Enrollement_E{ get; set; }
}
public class Enrollement{
[Key]
public int EnrolId {get; set;}
[ForeignKey("Studentid ")]
public int Student{get; set;}
public string kk{get; set;}
public virtual Student Student_E { get; set; }
}
在连接的OnModelCreating方法内,定义类似的关系,它将确保您具有Student对象,其相关或子对象时,即也将保存Enrollements
modelBuilder.Entity<Student>()
.HasMany(c => c. Enrollement_E)
.WithOne(e => e. Student_E);
modelBuilder.Entity<Enrollement>().Ignore(x => x. Student_E);
现在您的代码应如下所示
try{
student _student = new student{fname = "fname", lname="lname"};
_student.Enrollement_E = new List< Enrollement>();
_student.Enrollement_E.add(new Enrollement{student=???, kk="test"});
repository.stu.insert(_student);
}
finally
{
repository.save();
}
这是使用Entity Framework Core完成的最后一件事,遵循约定,您还需要更改外键字段
public int Student{get; set;} to public int StudentId{get; set;}
EF轻松处理外键以将数据插入到多个相关表中。 如果要同时在两个表中插入数据,则可以使用以下代码进行插入:
student st = new student
{
fname = "fname",
lname= "lname",
Enrollement_E=new List<Enrollement>
{
new Enrollement{kk="something"}
}
};
repository.student.Add(st);
repository.SaveChanges();
EF本身为第二张表做外键
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.