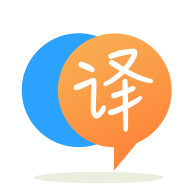
[英]EF Core, one-to-many relationship - how to insert records in both tables with a single view?
[英]Insert new Entity with one to many relationship not creating records in both tables
我正在使用MVC5和EF6 Code First创建一个可以具有许多Contact
实体的新Company
实体。 但是,只有Company
记录被写入数据库。
楷模:
public class Company
{
public virtual int CompanyId { get; set; }
[Required]
public virtual string Name { get; set; }
[Required]
public virtual Address Address { get; set; }
[Required]
public virtual string Phone { get; set; }
public virtual string Email { get; set; }
// can have many Contacts
public virtual IEnumerable<Contact> Contacts { get; set; }
}
public class Contact
{
public virtual int ContactId { get; set; }
[Required]
public virtual string Title { get; set; }
[Required]
public virtual string Forename { get; set; }
[Required]
public virtual string Surname { get; set; }
[Required]
public virtual string Phone { get; set; }
public virtual string Email { get; set; }
// belongs to one Company
public virtual Company Company { get; set; }
}
控制器:
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<ActionResult> Create_POST([Bind(Include = "CallerType,Name,Address,Phone,Email,Contacts")] CompanyViewModel viewModel)
{
if (ModelState.IsValid)
{
// tried commented code to make sure it wasn't a problem with my repository and contact records aren't saved to database...
//var context = new EfDbContext();
//var company = new Company
//{
// Name = viewModel.Name,
// Phone = viewModel.Phone,
// Address = viewModel.Address,
// Email = viewModel.Email
//};
//context.Companies.Add(company);
//var contact = new Contact
//{
// Company = company,
// Title = "mr",
// Forename= "f",
// Surname = "s",
// Phone = "132"
//};
//var contact2 = new Contact
//{
// Company = company,
// Title = "mr",
// Forename = "for",
// Surname = "sur",
// Phone = "987"
//};
//var contacts = new List<Contact> {contact, contact2};
//company.Contacts = contacts;
//context.SaveChanges();
var contacts = viewModel.Contacts.Select(c => new Contact
{
Title = c.Title,
Forename = c.Forename,
Surname = c.Surname,
Phone = c.Phone,
Email = c.Email
});
var company = new Company
{
Name = viewModel.Name,
Phone = viewModel.Phone,
Address = viewModel.Address,
Email = viewModel.Email,
Contacts = contacts
};
await _companyRepository.CreateAsync(company);
var redirectUrl = new UrlHelper(Request.RequestContext).Action("Create", "Enquiry", new { id = company.CompanyId, callerType = viewModel.CallerType });
return Json(new { Url = redirectUrl });
}
Response.StatusCode = 400;
return PartialView("~/Views/Company/_Create.cshtml", viewModel);
}
仓库:
public async Task<TEntity> CreateAsync(TEntity entity)
{
if (entity == null) throw new ArgumentNullException(nameof(entity));
DbContext.Set<TEntity>().Add(entity);
await DbContext.SaveChangesAsync();
return entity;
}
我的印象是DbContext
会“知道”,因为填充了Contacts
集合,它将创建链接到创建的Company
Contact
记录。 还是我必须创建Contact
因为这是其中包含外键的表,EF会“知道”首先创建Company
吗?
还是需要首先使用SaveChanges()
实际保存Company
实体,然后将其分配给Contact
记录并执行第二个SaveChanges()
?
如果是这种情况,那么我需要使用Database.BeginTransaction()
吗?
关于这方面的任何指导都将是很棒的,因为这是我第一次使用实体框架。
Contacts
必须是ICollection
。 EF不支持将IEnumerable
用作导航属性,因为无法向其中添加项目。
这也意味着您应该更改创建contacts
的代码:
var contacts = viewModel.Contacts.Select(c => new Contact
{
Title = c.Title,
Forename = c.Forename,
Surname = c.Surname,
Phone = c.Phone,
Email = c.Email
}).ToList(); // <= ToList() added
您的关系应通过覆盖OnModelCreating方法在DbContext中建立
我本人是Entity Framework的新手,但发现方法名称有助于学习如何建立关系
希望以下内容能对您有所帮助
protected override void OnModelCreating(DbModelBuilder modelBuilder)
{
modelBuilder.Entity<Company>()
.HasMany(c => c.Contacts)
.WithRequired(co => co.Company)
.HasForeignKey(co => co.CompanyId);
}
您还可以在您的关系中设置级联,这样,当删除一个实体时,相关实体也将被删除,如果您需要删除公司,可能需要考虑这一点。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.