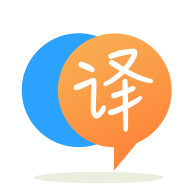
[英]JavaScript Prototypal Inheritance Chain: “that” technique for accessing upper level properties and methods?
[英]Prototypal inheritance chain with JavaScript
function Person(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
Person.prototype.talk = function () {
return this.firstName + " " + this.lastName;
}
//creating a Person object for extension
var manager = new Person('jon', 'doe');
console.log(manager.talk());
//Manager prototype..but doesn't inherit Person methods
function Manager(firstName, lastName, accessCode) {
//shared properties
this.firstName = firstName;
this.lastName = lastName;
this.accesscode = accessCode;
}
function personChecker(person) {
var returnCode = 0;
if (person instanceof Person) {
returnCode = 1;
}
else if (person instanceof Manager) {
returnCode = 2;
}
return returnCode;
}
console.log(personChecker(manager));
是否可以共享原型并拥有不同的构造函数? 我想让Manager从Person继承所有内容(然后扩展它),并在原型上进行功能切换,并根据传递给personChecker
函数的参数执行不同的personChecker
支持这种继承的典型方法:
// create a blank function to pass the prototype
var blank = function() {};
// assign the prototype to inherit to the blank constructor
blank.prototype = Person.prototype;
// pass an instance of the prototype as the Manager prototype
Manager.prototype = new blank();
var person = new Person('John', 'Doe');
var manager = new Manager('Greg', 'Smith', 'access1234');
manager.talk(); // Greg Smith
person.talk(); // John Doe
这是一个小提琴: http : //jsfiddle.net/XF28r/
请注意,经理也是人,因此您需要切换支票。
function personChecker(person) {
var returnCode = 0;
if (person instanceof Manager) {
returnCode = 2;
} else if (person instanceof Person) {
returnCode = 1;
}
return returnCode;
}
尽管注意,我会将其放在辅助方法中:
function extend(parent, child) {
var blank = function() {};
blank.prototype = parent.prototype;
child.prototype = new blank();
}
因此,您可以简单地使用它:
extend(Person, Manager);
正如Bergi在评论中提到的那样,这也可以简化为:
function extend(parent, child) {
child.prototype = Object.create(parent.prototype);
}
(适用于IE 9或更高版本)
在JavaScript中,继承通常是这样完成的:
Manager.prototype = Object.create(Person.prototype);
Object.create
创建一个新对象,并将该对象作为其原型链中的第一个参数传递。
如果您需要支持没有Object.create
旧浏览器,则可以执行“原型舞蹈”:
function F(){}
F.prototype = Person.prototype;
Manager.prototype = new F();
无论如何,避免代码调用构造函数来获取这样的对象( Manager.prototype = new Person()
),这是众所周知的反模式,一旦构造函数依赖于其任何参数,它就会中断(并且会导致其他更细微的问题)。
使用以下内容:
function Person(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
Person.prototype.talk = function () {
return this.firstName + " " + this.lastName;
}
function Manager(firstName, lastName, accessCode) {
//shared properties
this.firstName = firstName;
this.lastName = lastName;
this.accesscode = accessCode;
}
Manager.prototype = new Person('jon', 'doe');
function personChecker(person) {
var returnCode = 0;
if (person instanceof Manager) {
returnCode = 2;
}
else if (person instanceof Person) {
returnCode = 1;
}
return returnCode;
}
请注意,我更改了条件语句的顺序,因为Manager
的实例也是Person
的实例:
var per = new Person('A', 'B');
per.talk(); // 'A B'
per instanceof Person; // true
per instanceof Manager; // false
personChecker(per); // 1
var man = new Manager('A', 'B');
man.talk(); // 'A B'
man instanceof Person; // true !!!!
man instanceof Manager; // true !!!!
personChecker(man); // 2
如果你想做的好方法,而不是
Manager.prototype = new Person('jon', 'doe');
采用
Manager.prototype = Object.create(Person.prototype, {constructor: {value: Manager}});
但它不适用于旧的浏览器。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.