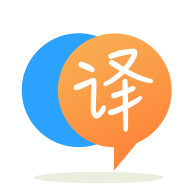
[英]Passing a structure by value, with another structure as one of its members, changes values of this member's members
[英]How to sort an array of structures according to values of one of its members, breaking ties on the basis of another member?
假设有一个结构:
struct x
{
int a,b,c;
};
结构数组包含arr [0] = {4,2,5},arr [1] = {6,3,1},arr [2] = {4,1,8}
那么如何根据成员'a'的值升序对该数组排序。 会根据成员“ b”的值来打断领带。
因此排序后的数组应为:arr [2],然后是arr [0],然后是arr [1]。
我用过qsort(arr,n,sizeof(struct x),compare);
比较函数定义为
int compare(const void* a, const void * b)
{
return (*(int*)a-*(int*)b);
}
如果我必须根据成员b断开关系,我该怎么做(当前是根据先到先得的关系断开关系)。
int compare(const void* a, const void * b){
struct x x = *(struct x*)a;
struct x y = *(struct x*)b;
return x.a < y.a ? -1 : (x.a > y.a ? 1 : (x.b < y.b ? -1 : x.b > y.b));
}
如果您使用的是C而不是C ++,则可以通过以下compare()
来完成:
int compare(const void* a, const void* b) {
struct x s_a = *((struct x*)a);
struct x s_b = *((struct x*)b);
if(s_a.a == s_b.a)
return s_a.b < s_b.b ? -1 : 1; //the order of equivalent elements is undefined in qsort() of stdlib, so it doesn't matter to return 1 directly.
return s_a.a < s_b.a ? -1 : 1;
}
如果要在成员a和b相等时根据成员c打破关系,请在compare()
添加更多if-else
语句。
与适当的比较器一起使用std::sort
。 本例中使用std::tie
实现使用逐一比较a
先b
,但是你可以手工编写自己的。 唯一的要求是,它必须满足严格的弱排序 :
bool cmp(const x& lhs, const x& rhs)
{
return std::tie(lhs.a, lhs.b) < std::tie(rhs.a, rhs.b);
}
std::sort(std::begin(arr), std::end(arr), cmp);
或使用lambda:
std::sort(std::begin(arr),
std::end(arr),
[](const x& lhs, const x& rhs)
{
return std::tie(lhs.a, lhs.b) < std::tie(rhs.a, rhs.b);
});
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.