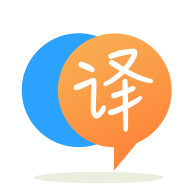
[英]How to reach the member data of a class from inside one of its member class/struct?
[英]How to sort an array of struct/class based on its member data?
如何根据成员数据对结构/类数组进行排序,因为这失败了?
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
struct O{
const string n;
int a=1;
};
bool bfunction (O a, O b) {
return a.n < b.n; }
int main () {
O m[]={ {"unta"}, {"jalan"}, {"sama"}, {"aki"} };
// using function in sort control
sort (m.begin(), m.end(), &bfunction);
}
gcc 给出:
error: request for member ‘begin’ in ‘m’, which is of non-class type ‘O [4]’
sort (m.begin(), m.end(), &bfunction);
^~~~~
error: request for member ‘end’ in ‘m’, which is of non-class type ‘O [4]’
sort (m.begin(), m.end(), &bfunction);
^~~~~
真诚有用的帮助表示赞赏
使用std::array
#include <iostream>
#include <algorithm>
#include <vector>
#include <array>
struct O {
std::string n;
int a;
O(const char* c) : a(1) { n = c; }
};
bool bfunction(O a, O b) {
return a.n < b.n;
}
int main() {
std::array<O, 4> m = { "unta", "jalan", "sama", "aki" };
// using function in sort control
std::sort(m.begin(), m.end(), &bfunction);
}
这里犯了一些错误:
sort (m.begin(), m.end(), &bfunction);
在O[]
上调用begin()
和end()
。 但是数组没有任何成员函数。
您在这里有一些选择:要么使m
成为std::array<O, 4>
或std::vector<O>
或使用适用于静态数组的std::begin(m)
和std::end(m)
.
排序函数应该通过常量引用来获取它的参数:
bool bfunction (const O &a, const O &b)
在排序函数中, an < bn
比较两个字符串数组,但在任何地方都没有定义这样的比较。 这是您需要解决的逻辑错误。 想想你真正想在这里比较什么。 比较是为std::string
定义的,例如return an[0] < bn[0];
会工作。
排序任何元素时都需要移动。 但是您的 struct O
没有移动构造函数,因为您没有提供一个,并且自动生成的构造函数会格式错误,因为O
具有const
成员。
我认为解决这个问题的最好方法是将所有成员变量设为私有并通过 getter 和 setter 控制对它们的访问。 目前,最简单的方法是删除const
。
这是我如何做的一个可复制粘贴的示例(假设您的n
应该只是一个字符串,而不是一个包含七个字符串的数组)。 如果你真的想要一个字符串数组作为n
,那么你必须为它们定义一个正确的顺序。
#include <iostream>
#include <algorithm>
#include <vector>
#include <array>
class O {
std::string n;
int a;
public:
O(const char* c, int a = 1) : n(c), a(a) {}
const std::string& get_n() const { return n; }
};
bool bfunction(const O& a, const O& b) {
return a.get_n() < b.get_n();
}
int main() {
std::array<O, 4> m = { "unta", "jalan", "sama", "aki" };
std::sort(m.begin(), m.end(), &bfunction);
for(auto& x : m) {
std::cout << x.get_n() << ',';
}
}
现场代码在这里。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.