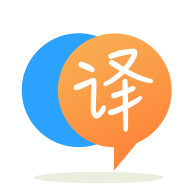
[英]Define a std::map with a templated Key type based on a function that is received as a parameter
[英]How to determine that a type received in the templated function is a list?
假设我有一个模板化函数,仅打印它在调用中刚刚收到的类型。
template<typename T>
void printType()
{
if (typeid(T) == typeid(int))
{
printf("Type is int.\r\n");
}
else if (typeid(T) == typeid(std::string))
{
printf("Type is string.\r\n");
}
else if (typeid(T) == typeid(MyList<int>))
{
printf("Type is a list.\r\n");
}
else
{
printf("Type is not supported.\r\n");
}
}
int main()
{
printType<int>();
printType<std::string>();
printType<MyList<int>>();
return 0;
}
函数必须处理的类型的支持仅限于某些类型:整数,字符串和列表。
我的问题是MyList类型。 在我的示例代码中,该函数仅处理int列表,但我希望使其处理一个列表,而与它包含的元素类型无关(例如: MyList<double>
, MyList<std::string>
, MyList<MyList<MyList<int>>>
等)。
这仅是我的问题的最小代表。
我如何检测到函数调用提供的类型是MyList,而不管其包含的type元素是什么?
不需要typeid
。 只需使用T类型来分派给不同的重载:
void printType_impl(int) {}
template<typename T>
void printType_impl(myList<T>) {}
void printType_impl(std::string) {}
template<typename T>
void printType()
{
printType_impl(T());
}
如果您担心默认构造可能不允许编译器进行优化的printType_impl
,那么请让printType_impl
取相应的指针,并像printType( static_cast<T*>(nullptr) );
一样调用它printType( static_cast<T*>(nullptr) );
代替。
您可以专门化结构(不是函数)并忽略typeid:
#include <cstdio>
#include <string>
template <typename T>
struct MyList {};
namespace PrintType {
template <typename T>
struct Implementation {
static void apply() {
printf("Type is not supported.\r\n");
}
};
template <>
struct Implementation<int> {
static void apply() {
printf("Type is int.\r\n");
}
};
// And more ...
template <typename T>
struct Implementation< MyList<T> > {
static void apply() {
printf("Type is a list.\r\n");
}
};
}
template <typename T>
void printType() {
PrintType::Implementation<T>::apply();
}
int main()
{
printType<int>();
printType<std::string>();
printType<MyList<int>>();
return 0;
}
输出:
Type is int.
Type is not supported.
Type is a list.
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.