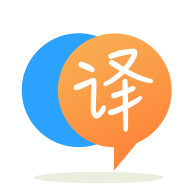
[英]How to define method in class that can only be called from __init__ method
[英]Where to define a method that is used only inside __init__ and must not be called anywhere else?
class Line(object):
def __init__(self):
self.length = None
self.run_equation()
def run_equation(self):
# it runs through a formula then assigns the end value to length
self.length = 50 # 50 for example
现在,我想用几个Line
对象填充一个列表。 事情是我不想再次调用run_equation()
,因为我已经在__init__
内分配了属性,并且它的值一定不能更改。
我应该遵循什么方法,或者我必须坚持这一思路,而不必从实例中调用该方法?
PS:在Google上找不到很多,或者我只是不知道如何搜索这个问题。
Python尝试从外部用户隐藏以2个下划线开头的方法和数据。 它并不完美,但是下划线告诉程序员您确实不希望他们摆弄它们。 添加一个属性以获取长度的只读视图,我想您将拥有所需的内容:
class Line(object):
@property
def length(self):
"""The length of the line"""
return self.__length
def __init__(self):
"""Create a line"""
self.__length = None
self.__run_equation()
def _run_equation(self):
self.__length = 50
试运行显示访问受限
>>> from line import Line
>>> l=Line()
>>> l.length
50
>>> l.length = 1
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: can't set attribute
>>> l.__length = 1
>>> l.__run_equation()
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'Line' object has no attribute '__run_equation'
>>> l.length
50
>>> help(Line)
Help on class Line in module line:
class Line(__builtin__.object)
| Methods defined here:
|
| __init__(self)
| Create a line
|
| ----------------------------------------------------------------------
| Data descriptors defined here:
|
| __dict__
| dictionary for instance variables (if defined)
|
| __weakref__
| list of weak references to the object (if defined)
|
| length
| The length of the line
如果在__init__
只有一个地方使用它,那么您就不需要单独的方法了。 只需将该方法的内容直接放入__init__
即可:
class Line (object):
def __init__ (self):
self.length = 50
可以在另一个函数中定义一个函数,并且该函数只能从包含的函数中看到:
def example():
def pointless_function():
print("Why do I exist, again?")
pointless_function()
example()
pointless_function()
完成后,您可以摆脱它:
class Line(object):
def __init__(self):
self.length = None
self.assign_value_to_length()
self.assign_value_to_length = None
def assign_value_to_length(self):
self.length = 50
不过,这不是很好的做法。 相反,我只是添加一个断言:
def assign_value_to_length(self):
assert self.length is None
self.length = 50
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.