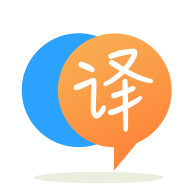
[英]how can i concatenate determined number of characters with another string?
[英]How can I find the number of unique characters in a string?
我没有发现任何特别适合这个目的。
我试图找出一个函数来计算字符串中每个字符的出现次数,以便我可以在最后从长度中取出它们以找出该字符串中使用了多少同质字符。
我试过嵌套循环,第一个应用,第二个扫描字符串,如果它没有出现在字符串的其他地方,则有条件地满足该字符:
size_t CountUniqueCharacters(char *str)
{
int i,j;
char unique[CHAR_MAX];
for(i=strlen(str); i>=0; i--)
{
for(j=strlen(str); j>=0; j--)
{
if(str[i] != unique[j])
unique[j] = str[i];
}
}
return strlen(unique);
}
这并不奏效。
如果您愿意限制某人键入诸如"aaaaaaaaaaaaa"
类的懒惰名称,这将很有用。
这是一个简单的 C++ 解决方案。 该方法的复杂度为 O(n):
int countDistinct(string s)
{
unordered_map<char, int> m;
for (int i = 0; i < s.length(); i++) {
m[s[i]]++;
}
return m.size();
}
这个方法有O(n^2)
复杂度,但是很可能(虽然有点复杂)在O(n)
做到这一点。
int CountUniqueCharacters(char* str){
int count = 0;
for (int i = 0; i < strlen(str); i++){
bool appears = false;
for (int j = 0; j < i; j++){
if (str[j] == str[i]){
appears = true;
break;
}
}
if (!appears){
count++;
}
}
return count;
}
该方法迭代字符串中的所有字符——对于每个字符,它检查该字符是否出现在任何前面的字符中。 如果不是,则该字符是唯一的,并且计数递增。
好吧,您可以为此使用 HashSet 或 unordered_set,但它的最坏情况时间复杂度为 O(N)。 因此,最好使用 256 个内存位置的数组或arr[256]
。 这在 O(256)~ O(1) 时间内给出了所需的输出
我发现以下计算不同字符的方法非常简单,并且在O(n)
。 这里的逻辑是,只需遍历字符数组,并为每个字符设置其计数1
,即使它重复,也只用1
覆盖该值。 完成遍历后,只需将所有字符出现次数相加即可。
int count_distinc_char(const char *a){
int c_arr[MAX_CHAR] = {0};
int i, count = 0;
for( i = 0; a[i] != '\0'; i++){
c_arr[a[i] - 'a'] = 1;
}
for( i = 0; i < MAX_CHAR; i++){
count += c_arr[i];
}
return count;
}
创建一个链表来存储在字符串中找到的字符及其出现的节点结构,如下所示,
struct tagCharOccurence
{
char ch;
unsigned int iCount;
};
现在一一读取字符串中的所有字符,并在读取一个字符时检查它是否存在于链表中,如果是,则增加其计数,如果在链表中找不到字符,则插入一个带有 'ch 的新节点' 设置为读取字符并将计数初始化为 1。
通过这种方式,您将仅在单次传递中获得每个字符的出现次数。 您现在可以使用链表打印遇到的字符次数。
我刚刚在 Stack Overflow 上寻找其他一些东西时遇到了这个问题。 但我仍然发布了一个可能对某些人有帮助的解决方案:
这也用于实现 huffman conding here 。 在那里你需要知道每个字符的频率,所以比你需要的多一点。
#include <climits>
const int UniqueSymbols = 1 << CHAR_BIT;
const char* SampleString = "this is an example for huffman encoding";
左移运算符将 lhs(即 1) CHAR_BIT
次向左移动,因此乘以 2^8(在大多数计算机上)为 256,因为 UTF-8 中有 256 个唯一符号
在你的main
你有
int main() {
// Build frequency table
int frequencies[UniqueSymbols] = {0};
const char* ptr = SampleString;
while (*ptr != '\0') {
++frequencies[*ptr++];
}
}
我发现它非常小而且很有帮助。 唯一的缺点是frequencies
的大小在这里是 256,唯一性只是检查哪个值是 1。
这是计算唯一字数的 C 程序的源代码。 C程序在Linux系统上编译成功并运行
int i = 0, e, j, d, k, space = 0;
char a[50], b[15][20], c[15][20];
printf("Read a string:\n");
fflush(stdin);
scanf("%[^\n]s", a);
for (i = 0;a[i] != '\0';i++) //loop to count no of words
{
if (a[i] = = ' ')
space++;
}
i = 0;
for (j = 0;j<(space + 1);i++, j++) //loop to store each word into an 2D array
{
k = 0;
while (a[i] != '\0')
{
if (a[i] == ' ')
{
break;
}
else
{
b[j][k++] = a[i];
i++;
}
}
b[j][k] = '\0';
}
i = 0;
strcpy(c[i], b[i]);
for (e = 1;e <= j;e++) //loop to check whether the string is already present in the 2D array or not
{
for (d = 0;d <= i;d++)
{
if (strcmp(c[i], b[e]) == 0)
break;
else
{
i++;
strcpy(c[i], b[e]);
break;
}
}
}
printf("\nNumber of unique words in %s are:%d", a, i);
return 0;
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.