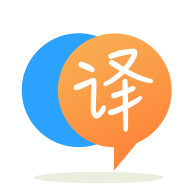
[英]Is there any way to check if any or all characters are present in a string in C?
[英]C: How to find that a certain number of characters are present in a string in any sequence?
所以给定的任务是创建一个函数来检查任何字符串if
例子:
checkabcde(“someaxbxcxdxemm”) -> 1
checkabcde(“someOtherValue”) -> 0
checkabcde(“xyabcdeping”) -> 2
checkabcde(“someaxuxdxlxammabcde”) -> 2
在我的方法中,我已经能够找到子串是“abcde”但无法确定该字符串在任何序列中包含'a','b','c','d','e'
int checkabcde(char str[]) {
char str2[] = {
'a',
'b',
'c',
'd',
'e'
};
char str3[5]; //to be filled with elements from str2 when found inconsecutive order
int i, z, x, f;
z = 0; //position for str3
f = 0; //flag for similarity comparison of str2 and str3
for (i = 0; i < strlen(str); i++) {
for (x = 0; x < strlen(str2); x++) {
if (str[i] == str2[x]) {
if ((str[i] == 'a') && (str[i + 1] == 'b') && (str[i + 2] == 'c') && (str[i + 3] == 'd') && (str[i + 4] == 'e')) {
return 2;
} else {
if (str3[z] != str[z - 1]) {
str3[z] = str2[x];
z++;
}
}
}
}
}
for (i = 0; i < 5; i++) {
for (x = 0; x < 5; x++) {
if (str2[i] == str3[x]) {
f++;
}
}
}
if (f == 5) {
return 1;
} else if (f1 == 0) {
return 0;
}
}
编辑:不允许指针
s1
所有char使用f[256]
set f[ch] = 1
然后检查s2
每个char的f[ch] == 1
strstr()
参见http://man7.org/linux/man-pages/man3/strstr.3.html 以下code
可以工作:
#include <stdio.h>
#include <string.h>
int check(const char* s1, const char* s2) {
int f[256];
memset(f, 0, sizeof(f));
// check 1
for (int i = 0; s1[i] != '\0'; ++i)
f[s1[i]] = 1;
for (int i = 0; s2[i] != '\0'; ++i)
if (f[s2[i]] == 0)
return 0;
// check 2
return strstr(s1, s2) == NULL ? 1 : 2;
}
int main(void) {
printf("%d\n", check("someaxbxcxdxemm", "abcde"));
printf("%d\n", check("someOtherValue", "abcde"));
printf("%d\n", check("xyabcdeping", "abcde"));
printf("%d\n", check("someaxuxdxlxammabcde", "abcde"));
return 0;
}
除了查找子字符串之外,您还可以使用单独的结构来检查各个字符。
可以使用五个bool的数组来存储每个角色的礼物。 例如:
bool chars[5] = {false};
for (int i = 0; i < strlen(str); i++) {
char c = str[i];
switch(c) {
case 'a':
chars[0] = true;
break;
case 'b':
chars[1] = true;
break;
// include cases for each character you are looking for
}
}
如果最后chars
数组中的每个条目都为true,则表示该字符串包含所有字符。
除了您已经在做的匹配子字符串之外,您还可以执行此操作。
尝试这样的事情:
int checkabcde(char str[]) {
// Check if string contains substring "abcde"
if (strstr(str, "abcde") != NULL) {
return 2;
}
int charCounts[5] = {0, 0, 0, 0, 0};
int length = strlen(str);
int i = 0;
// Keep counts of each occurrence of a,b,c,d,e
for(; i < length; i++) {
// If "abcde" contains the current character
if (strchr("abcde", str[i]) != NULL) {
charCounts[str[i] - 'a']++;
}
}
i = 0;
// Check if any of the counts for a,b,c,d,e are 0
for (; i < 5; i++) {
if (charCounts[i] == 0) {
return 0;
}
}
// Otherwise we must have found at least 1 of each a,b,c,d,e
return 1;
}
在str
的每个索引处, sub
循环查找子串匹配。 如果找到,则返回2。 found
循环查看该字符str [index]是否是abcde
。 如果是,则将check
数组中的匹配索引设置为space。
在处理了str
所有字符后,比较abcde
以进行check
。 如果匹配,则str
缺少该匹配字符。 返回0。
#include <stdio.h>
#include <string.h>
int checkabcde ( char str[]) {
char abcde[] = "abcde";
char check[] = "abcde";
int index = 0;
int match = 1;
int length = strlen ( str);
int span = strlen ( abcde);
while ( str[index]) {
if ( str[index] == abcde[0]) {
match = 1;
for ( int sub = 0; sub < span; ++sub) {
if ( index + sub > length || str[index + sub] != abcde[sub]) {
match = 0;
break;
}
}
if ( match) {
return 2;
}
}
for ( int found = 0; index + found < length && found < span; ++found) {
if ( str[index + found] == abcde[found]) {
check[found] = ' ';
}
}
index++;
}
for ( int found = 0; found < span; ++found) {
if ( check[found] == abcde[found]) {
return 0;
}
}
return 1;
}
int main( void) {
char lines[][30] = {
"someaxbxcxdxemm"
, "someOtherValue"
, "xyabcdeping"
, "someaxuxdxlxammabcde"
, "vu4ndljeibn2c9n@aiendjba"
, "dbcaeddeaabcceabcde"
};
for ( int each = 0; each < 6; ++each) {
printf ( "for [%s] result = %d\n", lines[each], checkabcde ( lines[each]));
}
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.