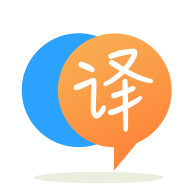
[英]Chrome automation extension locked when running Selenium test case
[英]Selenium Automation Test Case for Google Search
package com.test.webdriver;
import static org.junit.Assert.fail;
import java.util.concurrent.TimeUnit;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
public class TestExample {
private WebDriver driver;
private String baseUrl;
private StringBuffer verificationErrors = new StringBuffer();
@Before
public void setUp() throws Exception {
driver = new FirefoxDriver();
baseUrl = "http://www.google.com";
driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS);
}
@Test
public void testExample() throws Exception {
driver.get(baseUrl + "/webhp?tab=ww&ei=PaDQU4j6N4-QuATW2oB4&ved=0CBMQ1S4");
driver.findElement(By.xpath("id=gbqfq")).sendKeys("Test");
driver.findElement(By.xpath("id=gbqfba")).click();
}
@After
public void tearDown() throws Exception {
driver.quit();
String verificationErrorString = verificationErrors.toString();
if (!"".equals(verificationErrorString)) {
fail(verificationErrorString);
}
}
}
有人可以告诉我为什么上面的代码不起作用吗? 我在eclipse中运行上述报价并出现以下错误
Eclipse版本:3.7.1 Firefox版本:30
首先,您的xpath不正确,并且在文本框中键入内容后,不再显示您尝试单击的按钮。
这会起作用,
driver.findElement(By.xpath("//*[@id='gbqfq']")).sendKeys("Test");
driver.findElement(By.xpath("//*[@id='gbqfb']/span")).click();
您使用的是其他按钮的错误路径。 为什么?
bcoz,当您在Google中键入内容时,您尝试单击的按钮(Google按钮)将消失,并且将出现一个新页面(您会注意到文本框移至右上角,并且将出现一个蓝色搜索图标)。
因此,请注意应用程序中正在发生的事情,然后尝试使其自动化。
您的xpath无效。尝试:
“// * [@ id中= 'gbqfba']”
大多数浏览器将允许您测试Xpath。
或者,css选择器将是;
“#gbqfba”
或者Selenium还允许您通过id查找;
“gbqfba”
我个人更喜欢CSS选择器。 您选择XPath的原因是什么?
嗨,这应该现在可以工作了。我已经在“注释”框中留下了一些注释。希望这可以帮助您找到有助于定位的链接
https://thenewcircle.com/static/bookshelf/selenium_tutorial/locators.html
http://www.w3schools.com/xpath/xpath_syntax.asp
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
public class TestExample {
private WebDriver driver;
private String baseUrl;
private StringBuffer verificationErrors = new StringBuffer();
@Before
public void setUp() throws Exception {
driver = new FirefoxDriver();
baseUrl = "http://www.google.com";
driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS);}
@Test
public void testExample() throws Exception {
driver.get(baseUrl + "/webhp?tab=ww&ei=PaDQU4j6N4-QuATW2oB4&ved=0CBMQ1S4");
driver.findElement(By.xpath("//*[@id='gbqfq']")).sendKeys("Test");
/**Comment
The element with id "gbqfba" becomes invisble as page nagivates to results/ suggestion page
instead a button with id "gbqfb" becomes available to click to submit
driver.findElement(By.xpath("//*[@id='gbqfba']")).click(); */
//I decided to submit whatever is entered into the SearchField here and everything passes
driver.findElement(By.xpath("//*[@id='gbqfq']")).submit();
}
@After
public void tearDown() throws Exception {
driver.quit();
String verificationErrorString = verificationErrors.toString();
if (!"".equals(verificationErrorString)) {
fail(verificationErrorString);
}
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.