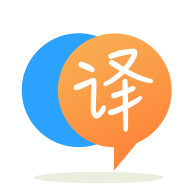
[英]How to add values from one dictionary to another based on matching keys?
[英]How to remove dictionary's keys and values based on another dictionary?
我希望根据另一个JSON字典的键和值删除一个JSON字典中的键和值。 从某种意义上说,我正在寻找一种“减法”。 假设我有JSON字典a
和b
:
a = {
"my_app":
{
"environment_variables":
{
"SOME_ENV_VAR":
[
"/tmp",
"tmp2"
]
},
"variables":
{ "my_var": "1",
"my_other_var": "2"
}
}
}
b = {
"my_app":
{
"environment_variables":
{
"SOME_ENV_VAR":
[
"/tmp"
]
},
"variables":
{ "my_var": "1" }
}
}
想象一下,你可以做a
- b
= c
其中c
看起来是这样的:
c = {
"my_app":
{
"environment_variables":
{
"SOME_ENV_VAR":
[
"/tmp2"
]
},
"variables":
{ "my_other_var": "2" }
}
}
如何才能做到这一点?
您可以使用字典中for key in dictionary:
遍历for key in dictionary:
并且可以使用del dictionary[key]
删除关键字,我想这就是您所需要的。 请参阅字典文档: https : //docs.python.org/2/tutorial/datastructures.html#dictionaries
以下内容满足您的需求:
def subtract(a, b):
result = {}
for key, value in a.items():
if key not in b or b[key] != value:
if not isinstance(value, dict):
if isinstance(value, list):
result[key] = [item for item in value if item not in b[key]]
else:
result[key] = value
continue
inner_dict = subtract(value, b[key])
if len(inner_dict) > 0:
result[key] = inner_dict
return result
它检查key
和value
是否都存在。 它可以del
项目,但我认为返回带有所需数据的新字典而不是修改原始字典要好得多。
c = subtract(a, b)
UPDATE
我刚刚更新了问题中提供的数据的最新版本。 现在,它也“减去”列表值。
更新2
工作示例: ipython笔记本
您可以这样做:
a
- > c
; b
内的每个key, value
对; top keys
是否具有相同的inner keys and values
,并从c
删除它们; empty values
keys
。 如果您的情况有所不同,则应修改代码(无dict(dict)
等)。
print(A)
print(B)
C = A.copy()
# INFO: Suppose your max depth is as follows: "A = dict(key:dict(), ...)"
for k0, v0 in B.items():
# Look for similiar outer keys (check if 'vars' or 'env_vars' in A)
if k0 in C:
# Look for similiar inner (keys, values)
for k1, v1 in v0.items():
# If we have e.g. 'my_var' in B and in C and values are the same
if k1 in C[k0] and v1 == C[k0][k1]:
del C[k0][k1]
# Remove empty 'vars', 'env_vars'
if not C[k0]:
del C[k0]
print(C)
{'environment_variables': {'SOME_ENV_VAR': ['/tmp']},
'variables': {'my_var': '2', 'someones_var': '1'}}
{'environment_variables': {'SOME_ENV_VAR': ['/tmp']},
'variables': {'my_var': '2'}}
{'variables': {'someones_var': '1'}}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.