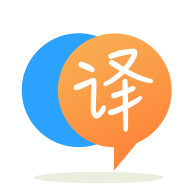
[英]How many clock cycles does cost AVX/SSE exponentiation on modern x86_64 CPU?
[英]How many CPU cycles does it take to execute my simple function?
出于教育目的,我想知道在对函数进行优化(不同级别)和编译后执行一个函数需要花费多少CPU周期。 有没有一种方法可以分析代码或可执行文件以获得可重现的答案? 我在64位Windows 7 Pro上使用带有MinGW的Eclipse Luna。
#include <math.h>
#include "main.h"
#define EPS 1e-15 // EPS a small number ~ machine precision
#define R2D 57.295779513082320876798154814105 //multiply radian with R2D to get degrees
#define D2R 0.01745329251994329576923690768489 //multiply degrees with D2R to get radians
#define TWO_PI 6.283185307179586476925286766559 //2*Pi
double _stdcall CourseInitial (double *lat1, double *lon1, double *lat2, double *lon2)
{
double radLat1 = D2R * *lat1;
double radLat2 = D2R * *lat2;
double radDeltaLon = D2R * (*lon2 - *lon1);
double tc = 0;
if (cos(radLat1) < EPS) { // EPS a small number ~ machine precision
if (radLat1 > 0) {
tc = 180; // Starting at N pole
} else {
tc = 0; // Starting at S pole
}
} else {
// Calculate true course [-180, 180)
tc = R2D * atan2(sin(radDeltaLon),
cos(radLat1) * tan(radLat2) - sin(radLat1) * cos(radDeltaLon)
);
}
if (fabs(tc) < EPS) {
tc = 0; //Prevents fmod(tc, 360) from returning 360 due to rounding error
} else {
tc += 360; //tc [180, 540)
}
return fmod(tc, 360); // returns tc [0, 360)
}
int main(void)
{
double lat1 = 67
double lon1 = 15;
double lat2 = 71;
double lon2 = 24;
double tc = 0;
tc = CourseInitial(&lat1, &lon1, &lat2, &lon2);
printf("The course from point 1 to 2 is: %.1f\n", tc);
return 0;
}
我这样做:
#include <math.h>
#include <cstdlib>
#include <cstdio>
#define EPS 1e-15 // EPS a small number ~ machine precision
#define R2D 57.295779513082320876798154814105 //multiply radian with R2D to get degrees
#define D2R 0.01745329251994329576923690768489 //multiply degrees with D2R to get radians
#define TWO_PI 6.283185307179586476925286766559 //2*Pi
double CourseInitial (double *lat1, double *lon1, double *lat2, double *lon2)
{
double radLat1 = D2R * *lat1;
double radLat2 = D2R * *lat2;
double radDeltaLon = D2R * (*lon2 - *lon1);
double tc = 0;
if (cos(radLat1) < EPS) { // EPS a small number ~ machine precision
if (radLat1 > 0) {
tc = 180; // Starting at N pole
} else {
tc = 0; // Starting at S pole
}
} else {
// Calculate true course [-180, 180)
tc = R2D * atan2(sin(radDeltaLon),
cos(radLat1) * tan(radLat2) - sin(radLat1) * cos(radDeltaLon)
);
}
if (fabs(tc) < EPS) {
tc = 0; //Prevents fmod(tc, 360) from returning 360 due to rounding error
} else {
tc += 360; //tc [180, 540)
}
return fmod(tc, 360); // returns tc [0, 360)
}
struct LatLon
{
double lat, lon;
};
struct CoursePoint
{
LatLon a, b;
};
const int SIZE = 1000000;
CoursePoint cps[SIZE];
double tc[SIZE];
LatLon RandomLatLon()
{
LatLon l;
l.lat = rand() % 90;
l.lon = rand() % 60;
return l;
}
static __inline__ unsigned long long rdtsc(void)
{
unsigned hi, lo;
__asm__ __volatile__ ("rdtsc" : "=a"(lo), "=d"(hi));
return ( (unsigned long long)lo)|( ((unsigned long long)hi)<<32 );
}
int main(void)
{
for(int i = 0; i < SIZE; i++)
{
cps[i].a = RandomLatLon();
cps[i].b = RandomLatLon();
}
unsigned long long t = rdtsc();
for(int i = 0; i < SIZE; i++)
{
tc[i] = CourseInitial(&cps[i].a.lat, &cps[i].a.lon, &cps[i].b.lat, &cps[i].b.lon);
}
t = rdtsc() - t;
printf("Time=%f\n", t/(double)SIZE);
double tot = 0;
for(int i = 0; i < SIZE; i++)
{
tot += tc[i];
}
printf("Sum of courses: %f\n", tot);
return 0;
}
每次迭代大约需要850-1000个周期。
如果您不运行这样的长时间循环,则杂散的事物将影响实际性能。 编译器优化选项的差别很小,添加-ffast-math的影响要大于-O0和-O3。 不同的编译器也有所不同。
g ++ 4.9.2:
-O0 1013 cycles
-O1 879 cycles
-O2 878 cycles
-O3 877 cycles
Add -ffast-math:
-O0 978
-O1 855 (re-run gives 890)
-O2 882
-O3 848 (re-run gives 850)
Clang ++(截至几周前为3.7):
-O0 998 cycles
-O1 954 cycles
-O2 955 cycles
-O3 957 cycles
Add -ffast-math:
-O0 967
-O1 872
-O2 865
-O3 875
截至昨日的Clang ++:
-O0 1001 cycles
-O1 956 cycles
-O2 948 cycles
-O3 949 cycles
Add -ffast-math:
-O0 969
-O1 871
-O2 869
-O3 873
请注意,小于10个时钟周期的差异可能在统计上并不显着。 我确实报告过一次跑步,但在此之前我尝试了几次,以确保大多数时候我得到相同的答案。
请注意,不同的处理器将产生完全不同的结果,并且不同的编译器版本显然会显示出一些差异。
编辑:只是为了好玩,我将其重写为Pascal,并通过我的Pascal编译器运行它,看看它也可以做什么。
用我的Pascal编译器编译的代码使用-O2(可用的最高级别)需要881个时钟周期。
FreePascal是Linux的“官方” Pascal编译器,没有可用的时钟周期计数器,所以我只做了time ./course
,结果大约是0.44s,而我的编译器代码是0.37s。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.