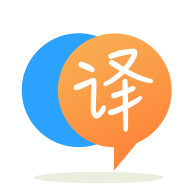
[英]How do I keep a div center when browser window goes smaller than div size?
[英]How to keep div in center-height when I resize the browser window?
我试图以高度为中心div,但是当我调整浏览器屏幕大小时它不起作用。
如何编辑它以在调整大小时实现可调节的margin-top?
谢谢
<script>
var h = $(window).height();
var parentHeight = h;
var childHeight = $('#a-middle').height();
$('#a-middle').css('margin-top', (parentHeight - childHeight) /2);
</script>
编辑:答案应该是js,因为flexbox不能在IE-9上运行
你应该坚持使用CSS解决方案,有几种方法可以实现这一点
.alignVertical {
position:relative;
display:inline-block;
top:50%;
transform:translateY(-50%);
}
jsfiddle: https ://jsfiddle.net/jorjmt70/
或使用flexbox
.parent {
display:flex;
height:100vh;
background-color:red;
justify-content:center;
align-items:center;
flex-direction:column;
}
jsfiddle: https ://jsfiddle.net/mdh9h876/
如果你想使用弹性盒使用autoprefixer来获得更深入的浏览器支持: https : //github.com/postcss/autoprefixer
虽然您可以使用纯CSS轻松完成此操作,但您的赏金表明您需要JS答案。
如果您对纯CSS答案感兴趣,请参阅此答案 , 该答案有多种不同方法可以垂直/水平居中。
您可以将jQuery简化为以下内容:
$('#a-middle').css('margin-top', function () {
return ($(window).height() - $(this).height()) / 2
});
然后你可以将它放在resize
事件监听器中并链接.resize()
方法,以便在浏览器加载时最初触发事件。
$(window).on('resize', function () {
$('#a-middle').css('margin-top', function () {
return ($(window).height() - $(this).height()) / 2
});
}).resize();
var verticalCentering = function () {
var el = document.querySelector('#a-middle');
el.style.marginTop = (window.innerHeight - el.offsetHeight) / 2 + 'px';
}
window.addEventListener('resize', verticalCentering);
verticalCentering();
对于一个名为'center-me'的div:
$(document).ready(centerDiv);
$(window).resize(centerDiv);
function centerDiv() {
var winHeight = $(document).innerHeight(),
divHeight = $('.center-me').height();
$('.center-me').css('marginTop', (winHeight - divHeight) / 2 );
}
您需要在文档准备就绪时调用它,首先使其居中,然后调整resize-event,以使其保持居中。
这是小提琴: 小提琴
要使div始终位于屏幕的中心,在将position属性设置为absolute之后,可以使用的属性是top和left属性。 但是,您需要在调整浏览器大小时动态设置这些属性。 这可以使用JQuery方法完成 - resize()。
/*css code*/
.div{
position:absolute;
top:100px;
left:50px;
background:black;
}
/*JS Code*/
function keep_div_centered()
{
window_height = $(window).height();
window_width = $(window).width();
obj_height = $('.keepincenter').height();
obj_width = $('.keepincenter').width();
$('.keepincenter').css('top',(window_height/2)-(obj_height/2)).css('left',(window_width/2)-(obj_width/2))
}
keep_div_centered();
$(window).resize(function(){
keep_div_centered();
})
/* HTML Code */
<div class="keepincenter"></div>
链接到小提琴 - http://jsfiddle.net/y0937t06/
CSS解决方案可以为您提供帮助。
div.center { height: 100px; width: 300px; top: 50%; left: 50%; margin-top: -50px; margin-left: -150px; position: absolute; background-color: red; }
<div class="center">Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</div>
这个CSS代码在IE8 +,Firefox和Chrome中运行良好。
但是您必须知道要正确调整的DIV的大小。 如果高度和宽度是动态的,您只需使用JavaScript相应地更新样式。 不要忘记将JS中的班级center
应用于您的DIV。
解释:
margin-top : - height / 2
因为top : 50%
仅垂直居中于DIV的顶部。 margin-left : - width / 2
因为left : 50%
只在DIV左边水平居中。 position : absolute
使DIV可以居中于所有页面。 这可以通过使用简单的CSS和IE8深度浏览器支持来实现 。
html, body { height: 100%; } .parent { display: table; table-layout: fixed; width: 100%; height: 100%; } .child { display: table-cell; vertical-align: middle; text-align: center; background: red; }
<div class="parent"> <div class="child">This is always centered.</div> </div>
使用表格布局使其变得简单:孩子可以垂直对齐(顶部,中间,底部)并占据所有可用高度。 你没有使用JavaScript,支持补丁的CSS或者不得不对任何数字进行硬编码,它应该可以正常工作。
根据你想要做的具体细节,flexbox或 - 作为最后的手段 - 可能需要JavaScript,但在大多数情况下display: table-cell
是你的朋友。
也就是说,如果旧浏览器可以接受不同的布局,只需使用@ Victor的答案:这就是flexbox的用途。
$(window).resize(function () {
var h = $(document).height();
var parentHeight = h;
var childHeight = $('#imagegallery').height();
$('#imagegallery').css('margin-top', (parentHeight - childHeight) / 2);
});
对我来说,这是CSS的圣杯:)我发现最可靠的方法是设置容器元素如下:
display: -webkit-flex;
-webkit-justify-content: center; /* horizontal */
-webkit-align-items: center; /* vertical */
它很简单,没有任何其他CSS属性的先决条件。
下面的小提琴将内容放置在垂直中心上方30px:
#container { width: 500px; height: 300px; background-color: red; display: -webkit-flex; -webkit-justify-content: center; -webkit-align-items: center; } #content { background-color: green; margin-bottom: 30px; }
<div id="container"> <span id="content">Content</span> </div>
处理当前窗口的window_resize事件并尝试在其上面放置代码。
它应该给你期望的功能。
当您想要垂直和水平居中绝对位置div时,此方法非常有用。 它也适用于IE8
您需要将外部和内部div设置为
position: absolute;
top: 0;
bottom: 0;
left: 0;
right: 0;
margin:auto;
margin:auto在这种情况下是中心div的基础。
您还可以设置.in div的高度和宽度,但是当您调整浏览器大小时,您仍会看到它垂直居中并居中。
* {
margin: 0;
padding: 0;
}
html, body {
position: relative;
height: 100%;
width: 100%;
}
.in, .out {
position: absolute;
top: 0;
bottom: 0;
left: 0;
right: 0;
margin:auto;
}
.in {
background-color: red;
height: 50%;
width:50%;
}
.out {
background-color: blue;
}
示例1 JSFIDDLE高度,宽度:百分比: http : //jsfiddle.net/a_incarnati/1o5zzcgh/3/
例2 - JSFIDDLE固定高度,固定宽度http://jsfiddle.net/a_incarnati/1o5zzcgh/4/
给出显示:table-cell; 到父级并使用vertical-align垂直对齐内容并给出填充以从顶部调整必要的间距。
.child{
display:table-cell;
vertical-align:top;
padding-top: 50px;
}
这将使整个保证金保持一致。
用css
首先给你的元素一个高度。
#a-middle {
height: 20px;
position: fixed;
top: calc(50% - 10px);
left: 0;
}
或使用js
$(function(){
$('#a-middle').each(function(){
var t = $(window).height()/2 - $(this).outerHeight()/2;
$(this).css({top: t + "px"});
});
});
$(window).resize(function(){
$('#a-middle').each(function(){
var t = $(window).height()/2 - $(this).outerHeight()/2;
$(this).css({top: t + "px"});
});
});
以前解决方案的问题在于,如果div大于可用的垂直尺寸,则无法将div居中。
如果您希望div占据页面的50%,您可以使用基于CSS垂直高度的单位:
.mydiv {
height: 50vh;
top: 50%;
left: 50%;
position: fixed;
width: 50vh;
margin-top: -25vh;
margin-left:-25vh;
border: solid black 1px;
}
因此,您的DIV不仅会居中,还会保持其比例。
使用窗口和div的宽度和高度,使用该div的margin-left和margin-top进行游戏。
$(function () {
makeDivCenter();
$(window).resize(function () {
makeDivCenter();
});
});
function makeDivCenter() {
var windowWidth = $(window).width();
var divWidth = $(".center").width();
var windowHeight = $(window).height();
var divHeight = $(".center").height();
$(".center").css({
'margin-left': (windowWidth / 2) - (divWidth / 2) + "px",
'margin-top': (windowHeight / 2) - (divHeight / 2) + "px"
});
}
以下是jsfiddle供您参考https://jsfiddle.net/fnuud7g6/
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.