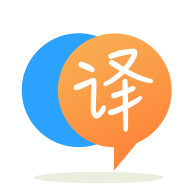
[英]How do I keep a div center when browser window goes smaller than div size?
[英]How to keep div in center-height when I resize the browser window?
我試圖以高度為中心div,但是當我調整瀏覽器屏幕大小時它不起作用。
如何編輯它以在調整大小時實現可調節的margin-top?
謝謝
<script>
var h = $(window).height();
var parentHeight = h;
var childHeight = $('#a-middle').height();
$('#a-middle').css('margin-top', (parentHeight - childHeight) /2);
</script>
編輯:答案應該是js,因為flexbox不能在IE-9上運行
你應該堅持使用CSS解決方案,有幾種方法可以實現這一點
.alignVertical {
position:relative;
display:inline-block;
top:50%;
transform:translateY(-50%);
}
jsfiddle: https ://jsfiddle.net/jorjmt70/
或使用flexbox
.parent {
display:flex;
height:100vh;
background-color:red;
justify-content:center;
align-items:center;
flex-direction:column;
}
jsfiddle: https ://jsfiddle.net/mdh9h876/
如果你想使用彈性盒使用autoprefixer來獲得更深入的瀏覽器支持: https : //github.com/postcss/autoprefixer
雖然您可以使用純CSS輕松完成此操作,但您的賞金表明您需要JS答案。
如果您對純CSS答案感興趣,請參閱此答案 , 該答案有多種不同方法可以垂直/水平居中。
您可以將jQuery簡化為以下內容:
$('#a-middle').css('margin-top', function () {
return ($(window).height() - $(this).height()) / 2
});
然后你可以將它放在resize
事件監聽器中並鏈接.resize()
方法,以便在瀏覽器加載時最初觸發事件。
$(window).on('resize', function () {
$('#a-middle').css('margin-top', function () {
return ($(window).height() - $(this).height()) / 2
});
}).resize();
var verticalCentering = function () {
var el = document.querySelector('#a-middle');
el.style.marginTop = (window.innerHeight - el.offsetHeight) / 2 + 'px';
}
window.addEventListener('resize', verticalCentering);
verticalCentering();
對於一個名為'center-me'的div:
$(document).ready(centerDiv);
$(window).resize(centerDiv);
function centerDiv() {
var winHeight = $(document).innerHeight(),
divHeight = $('.center-me').height();
$('.center-me').css('marginTop', (winHeight - divHeight) / 2 );
}
您需要在文檔准備就緒時調用它,首先使其居中,然后調整resize-event,以使其保持居中。
這是小提琴: 小提琴
要使div始終位於屏幕的中心,在將position屬性設置為absolute之后,可以使用的屬性是top和left屬性。 但是,您需要在調整瀏覽器大小時動態設置這些屬性。 這可以使用JQuery方法完成 - resize()。
/*css code*/
.div{
position:absolute;
top:100px;
left:50px;
background:black;
}
/*JS Code*/
function keep_div_centered()
{
window_height = $(window).height();
window_width = $(window).width();
obj_height = $('.keepincenter').height();
obj_width = $('.keepincenter').width();
$('.keepincenter').css('top',(window_height/2)-(obj_height/2)).css('left',(window_width/2)-(obj_width/2))
}
keep_div_centered();
$(window).resize(function(){
keep_div_centered();
})
/* HTML Code */
<div class="keepincenter"></div>
鏈接到小提琴 - http://jsfiddle.net/y0937t06/
CSS解決方案可以為您提供幫助。
div.center { height: 100px; width: 300px; top: 50%; left: 50%; margin-top: -50px; margin-left: -150px; position: absolute; background-color: red; }
<div class="center">Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</div>
這個CSS代碼在IE8 +,Firefox和Chrome中運行良好。
但是您必須知道要正確調整的DIV的大小。 如果高度和寬度是動態的,您只需使用JavaScript相應地更新樣式。 不要忘記將JS中的班級center
應用於您的DIV。
解釋:
margin-top : - height / 2
因為top : 50%
僅垂直居中於DIV的頂部。 margin-left : - width / 2
因為left : 50%
只在DIV左邊水平居中。 position : absolute
使DIV可以居中於所有頁面。 這可以通過使用簡單的CSS和IE8深度瀏覽器支持來實現 。
html, body { height: 100%; } .parent { display: table; table-layout: fixed; width: 100%; height: 100%; } .child { display: table-cell; vertical-align: middle; text-align: center; background: red; }
<div class="parent"> <div class="child">This is always centered.</div> </div>
使用表格布局使其變得簡單:孩子可以垂直對齊(頂部,中間,底部)並占據所有可用高度。 你沒有使用JavaScript,支持補丁的CSS或者不得不對任何數字進行硬編碼,它應該可以正常工作。
根據你想要做的具體細節,flexbox或 - 作為最后的手段 - 可能需要JavaScript,但在大多數情況下display: table-cell
是你的朋友。
也就是說,如果舊瀏覽器可以接受不同的布局,只需使用@ Victor的答案:這就是flexbox的用途。
$(window).resize(function () {
var h = $(document).height();
var parentHeight = h;
var childHeight = $('#imagegallery').height();
$('#imagegallery').css('margin-top', (parentHeight - childHeight) / 2);
});
對我來說,這是CSS的聖杯:)我發現最可靠的方法是設置容器元素如下:
display: -webkit-flex;
-webkit-justify-content: center; /* horizontal */
-webkit-align-items: center; /* vertical */
它很簡單,沒有任何其他CSS屬性的先決條件。
下面的小提琴將內容放置在垂直中心上方30px:
#container { width: 500px; height: 300px; background-color: red; display: -webkit-flex; -webkit-justify-content: center; -webkit-align-items: center; } #content { background-color: green; margin-bottom: 30px; }
<div id="container"> <span id="content">Content</span> </div>
處理當前窗口的window_resize事件並嘗試在其上面放置代碼。
它應該給你期望的功能。
當您想要垂直和水平居中絕對位置div時,此方法非常有用。 它也適用於IE8
您需要將外部和內部div設置為
position: absolute;
top: 0;
bottom: 0;
left: 0;
right: 0;
margin:auto;
margin:auto在這種情況下是中心div的基礎。
您還可以設置.in div的高度和寬度,但是當您調整瀏覽器大小時,您仍會看到它垂直居中並居中。
* {
margin: 0;
padding: 0;
}
html, body {
position: relative;
height: 100%;
width: 100%;
}
.in, .out {
position: absolute;
top: 0;
bottom: 0;
left: 0;
right: 0;
margin:auto;
}
.in {
background-color: red;
height: 50%;
width:50%;
}
.out {
background-color: blue;
}
示例1 JSFIDDLE高度,寬度:百分比: http : //jsfiddle.net/a_incarnati/1o5zzcgh/3/
例2 - JSFIDDLE固定高度,固定寬度http://jsfiddle.net/a_incarnati/1o5zzcgh/4/
給出顯示:table-cell; 到父級並使用vertical-align垂直對齊內容並給出填充以從頂部調整必要的間距。
.child{
display:table-cell;
vertical-align:top;
padding-top: 50px;
}
這將使整個保證金保持一致。
用css
首先給你的元素一個高度。
#a-middle {
height: 20px;
position: fixed;
top: calc(50% - 10px);
left: 0;
}
或使用js
$(function(){
$('#a-middle').each(function(){
var t = $(window).height()/2 - $(this).outerHeight()/2;
$(this).css({top: t + "px"});
});
});
$(window).resize(function(){
$('#a-middle').each(function(){
var t = $(window).height()/2 - $(this).outerHeight()/2;
$(this).css({top: t + "px"});
});
});
以前解決方案的問題在於,如果div大於可用的垂直尺寸,則無法將div居中。
如果您希望div占據頁面的50%,您可以使用基於CSS垂直高度的單位:
.mydiv {
height: 50vh;
top: 50%;
left: 50%;
position: fixed;
width: 50vh;
margin-top: -25vh;
margin-left:-25vh;
border: solid black 1px;
}
因此,您的DIV不僅會居中,還會保持其比例。
使用窗口和div的寬度和高度,使用該div的margin-left和margin-top進行游戲。
$(function () {
makeDivCenter();
$(window).resize(function () {
makeDivCenter();
});
});
function makeDivCenter() {
var windowWidth = $(window).width();
var divWidth = $(".center").width();
var windowHeight = $(window).height();
var divHeight = $(".center").height();
$(".center").css({
'margin-left': (windowWidth / 2) - (divWidth / 2) + "px",
'margin-top': (windowHeight / 2) - (divHeight / 2) + "px"
});
}
以下是jsfiddle供您參考https://jsfiddle.net/fnuud7g6/
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.