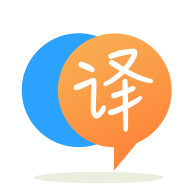
[英]Waiting for a timer elapsed event to complete before application/service closes/stops
[英]How to prevent Timer Elapsed event from occurring if forms application closes C#
我在Windows窗体应用程序(Visual C#)中有一个计时器,当我想退出应用程序时,它会导致问题。
计时器被定义为表单类的成员:
partial class Form1
{
//These are the members in question:
internal ComACRServerLib.Channel channel;
private System.Timers.Timer updateStuff;
}
在表单应用程序的构造函数中声明/构造计时器:
public Form1()
{
InitializeComponent();
updateStuff = new System.Timers.Timer();
updateStuff.Elapsed += new System.Timers.ElapsedEventHandler(updateStuff_Elapsed);
}
只需按一下按钮即可启动和配置计时器:
private void btnAcquire_Click(object sender, EventArgs e)
{
updateStuff.Interval = 100;
updateStuff.Enabled = true;
updateStuff.AutoReset = true;
updateStuff.Start();
}
当计时器过去时,它调用updateStuff_Elapsed
,它获取要用setText
显示的信息(有一些代码可以确保调用setText
是线程安全的)。
private void updateStuff_Elapsed(object sender, System.Timers.ElapsedEventArgs e)
{
if (!channel.isOffline)
{
object[] status = channel.GetACRCustom("P6144");
setText(System.Convert.ToString(status[0]));
}
}
public delegate void setTextDelegate(string text);
public void setText(string text)
{
if (this.lblTest.InvokeRequired == true)
{
setTextDelegate d = new setTextDelegate(setText);
this.Invoke(d, new object[] { text });
}
else
{
lblTest.Text = text;
}
}
在应用程序退出时,我尝试摆脱计时器,并使用以下内容阻止它再次触发:
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
updateStuff.AutoReset = false;
updateStuff.Stop();
updateStuff.Close();
updateStuff.Dispose();
components.Dispose();
}
base.Dispose(disposing);
}
但是如果计时器是自动运行的并且我退出程序,我总是得到错误,Timer Elapsed事件updateStuff_elapsed
调用的例程试图使用已经处理过的资源! 即使我已经尽力停止并在处理之前销毁计时器。
当应用程序关闭时,如何停止计时器的触发?
编辑
我尝试移动Dispose
代码以试图强制关闭计时器,但没有运气。 我还尝试使用updateStuff.Elapsed -= updateStuff_Elapsed
在停止和处理之前删除事件调用;
protected override void Dispose(bool disposing)
{
//now this code HAS to run always.
updateStuff.Elapsed -= updateStuff_Elapsed;
updateStuff.AutoReset = false;
updateStuff.Stop();
updateStuff.Close();
updateStuff.Dispose();
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
如System.Timers.Timer的文档中所述,Timer的事件处理程序调用在ThreadPool线程上排队。 因此,您必须假设事件处理程序可以一次调用多次,或者可以在禁用Timer后调用。 因此,事件处理程序必须设计为正确处理这些情况。
首先,将Timer的SynchronizingObject属性设置为Form的实例。 这将把所有事件处理程序的调用编组到UI线程,因此我们不需要打扰表单字段的锁定(我们将始终从同一个UI线程访问所有内容)。 使用此属性集,您也不需要在setText方法中调用this.Invoke(...)。
public Form1()
{
updateStuff = new System.Timers.Timer();
updateStuff.SynchronizingObject = this;
...
}
public void setText(string text)
{
lblTest.Text = text;
}
然后创建标志,让你知道,计时器是否被处置。 然后只需在事件处理程序中检查此标志:
partial class Form1
{
private bool Disposed;
....
}
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
updateStuff.Dispose();
Disposed = true;
}
base.Dispose(disposing);
}
private void updateStuff_Elapsed(object sender, System.Timers.ElapsedEventArgs e)
{
if(!Disposed)
{
if (!channel.isOffline)
{
object[] status = channel.GetACRCustom("P6144");
setText(System.Convert.ToString(status[0]));
}
}
}
处理dispose事件太晚了(在垃圾收集期间)。 您需要在FormClosing事件上执行清理过程。
为了扩展Ron和Marc所说的,解决方案是覆盖Form1
的子类的Form
的OnFormClosing
方法:
protected override void OnFormClosing(FormClosingEventArgs e)
{
//It appears it needs everything here to be turned off...amazing.
updateStuff.Elapsed -= updateStuff_Elapsed;
updateStuff.AutoReset = false;
updateStuff.Stop();
updateStuff.Dispose();
base.OnFormClosing(e);
}
另一个解决方案是将计时器放在与用户界面相同的线程上,正如Nuf所说。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.