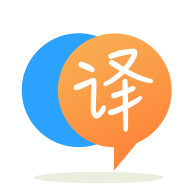
[英]Dependency Injection in Azure WebJobs SDK when using NoAutomaticTrigger?
[英]Dependency injection using Azure WebJobs SDK?
问题是 Azure WebJobs SDK 仅支持公共静态方法作为作业入口点,这意味着无法实现构造函数/属性注入。
我无法在官方 WebJobs SDK 文档/资源中找到有关此主题的任何信息。 我遇到的唯一的解决方案是基于对这个职位描述的服务定位器(反)模式在这里。
有没有一种好方法可以为基于 Azure WebJobs SDK 的项目使用“适当的”依赖项注入?
Azure WebJobs SDK现在支持实例方法。 将其与自定义IJobActivator相结合,您可以使用DI。
首先,创建可以使用您喜欢的DI容器解析作业类型的自定义IJobActivator:
public class MyActivator : IJobActivator
{
private readonly IUnityContainer _container;
public MyActivator(IUnityContainer container)
{
_container = container;
}
public T CreateInstance<T>()
{
return _container.Resolve<T>();
}
}
您需要使用自定义JobHostConfiguration注册此类:
var config = new JobHostConfiguration
{
JobActivator = new MyActivator(myContainer)
};
var host = new JobHost(config);
然后,您可以使用一个带有实例方法的简单类来完成您的工作(这里我使用的是Unity的构造函数注入功能):
public class MyFunctions
{
private readonly ISomeDependency _dependency;
public MyFunctions(ISomeDependency dependency)
{
_dependency = dependency;
}
public Task DoStuffAsync([QueueTrigger("queue")] string message)
{
Console.WriteLine("Injected dependency: {0}", _dependency);
return Task.FromResult(true);
}
}
这就是我使用新SDK处理范围的方法。 使用Alexander Molenkamp描述的IJobactivator。
public class ScopedMessagingProvider : MessagingProvider
{
private readonly ServiceBusConfiguration _config;
private readonly Container _container;
public ScopedMessagingProvider(ServiceBusConfiguration config, Container container)
: base(config)
{
_config = config;
_container = container;
}
public override MessageProcessor CreateMessageProcessor(string entityPath)
{
return new CustomMessageProcessor(_config.MessageOptions, _container);
}
private class CustomMessageProcessor : MessageProcessor
{
private readonly Container _container;
public CustomMessageProcessor(OnMessageOptions messageOptions, Container container)
: base(messageOptions)
{
_container = container;
}
public override Task<bool> BeginProcessingMessageAsync(BrokeredMessage message, CancellationToken cancellationToken)
{
_container.BeginExecutionContextScope();
return base.BeginProcessingMessageAsync(message, cancellationToken);
}
public override Task CompleteProcessingMessageAsync(BrokeredMessage message, FunctionResult result, CancellationToken cancellationToken)
{
var scope = _container.GetCurrentExecutionContextScope();
if (scope != null)
{
scope.Dispose();
}
return base.CompleteProcessingMessageAsync(message, result, cancellationToken);
}
}
}
您可以在JobHostConfiguration中使用自定义MessagingProvider
var serviceBusConfig = new ServiceBusConfiguration
{
ConnectionString = config.ServiceBusConnectionString
};
serviceBusConfig.MessagingProvider = new ScopedMessagingProvider(serviceBusConfig, container);
jobHostConfig.UseServiceBus(serviceBusConfig);
在询问了我自己关于如何处理范围的问题之后...我刚刚达成了这个解决方案:我认为这不是理想的,但我暂时找不到任何其他解决方案。
在我的例子中,我正在处理ServiceBusTrigger。
当我使用SimpleInjector时 ,IJobActivator接口的实现看起来像这样:
public class SimpleInjectorJobActivator : IJobActivator
{
private readonly Container _container;
public SimpleInjectorJobActivator(Container container)
{
_container = container;
}
public T CreateInstance<T>()
{
return (T)_container.GetInstance(typeof(T));
}
}
在这里,我正在处理Triggered webjobs。
所以我有两个依赖项:
单身人士:
public interface ISingletonDependency { } public class SingletonDependency : ISingletonDependency { }
而另一个只需要触发我的函数触发的时间:
public class ScopedDependency : IScopedDependency, IDisposable { public void Dispose() { //Dispose what need to be disposed... } }
因此,为了拥有一个独立于webjob运行的进程。 我已将我的流程封装到一个类中:
public interface IBrokeredMessageProcessor
{
Task ProcessAsync(BrokeredMessage incommingMessage, CancellationToken token);
}
public class BrokeredMessageProcessor : IBrokeredMessageProcessor
{
private readonly ISingletonDependency _singletonDependency;
private readonly IScopedDependency _scopedDependency;
public BrokeredMessageProcessor(ISingletonDependency singletonDependency, IScopedDependency scopedDependency)
{
_singletonDependency = singletonDependency;
_scopedDependency = scopedDependency;
}
public async Task ProcessAsync(BrokeredMessage incommingMessage, CancellationToken token)
{
...
}
}
所以现在当webjob启动时,我需要根据其范围注册我的依赖项:
class Program
{
private static void Main()
{
var container = new Container();
container.Options.DefaultScopedLifestyle = new ExecutionContextScopeLifestyle();
container.RegisterSingleton<ISingletonDependency, SingletonDependency>();
container.Register<IScopedDependency, ScopedDependency>(Lifestyle.Scoped);
container.Register<IBrokeredMessageProcessor, BrokeredMessageProcessor>(Lifestyle.Scoped);
container.Verify();
var config = new JobHostConfiguration
{
JobActivator = new SimpleInjectorJobActivator(container)
};
var servicebusConfig = new ServiceBusConfiguration
{
ConnectionString = CloudConfigurationManager.GetSetting("MyServiceBusConnectionString")
};
config.UseServiceBus(servicebusConfig);
var host = new JobHost(config);
host.RunAndBlock();
}
}
这是触发的工作:
它将范围处理为触发功能。
public class TriggeredJob { private readonly Container _container; public TriggeredJob(Container container) { _container = container; } public async Task TriggeredFunction([ServiceBusTrigger("queueName")] BrokeredMessage message, CancellationToken token) { using (var scope = _container.BeginExecutionContextScope()) { var processor = _container.GetInstance<IBrokeredMessageProcessor>(); await processor.ProcessAsync(message, token); } } }
我使用了一些依赖于子容器/范围概念的模式(取决于您选择的IoC容器的术语)。 不确定哪些支持它,但我可以告诉你StructureMap 2.6.x和AutoFac。
我们的想法是为每个进入的消息启动子范围,注入该请求唯一的上下文,从子范围解析顶级对象,然后运行您的进程。
这是一些使用AutoFac显示它的通用代码。 它确实从容器中直接解决,类似于您试图避免的反模式,但它被隔离到一个地方。
在这种情况下,它使用ServiceBusTrigger来激活作业,但可以是任何东西 - 作业主机可能有一个列表,用于不同的队列/进程。
public static void ServiceBusRequestHandler([ServiceBusTrigger("queuename")] ServiceBusRequest request)
{
ProcessMessage(request);
}
上述方法的所有实例都调用此方法。 它将子范围的创建包装在一个使用块中,以确保清理事物。 然后,将创建每个请求不同并包含其他依赖项(用户/客户端信息等)使用的上下文的任何对象,并将其注入子容器(在此示例中为IRequestContext)。 最后,执行工作的组件将从子容器中解析。
private static void ProcessMessage<T>(T request) where T : IServiceBusRequest
{
try
{
using (var childScope = _container.BeginLifetimeScope())
{
// create and inject things that hold the "context" of the message - user ids, etc
var builder = new ContainerBuilder();
builder.Register(c => new ServiceRequestContext(request.UserId)).As<IRequestContext>().InstancePerLifetimeScope();
builder.Update(childScope.ComponentRegistry);
// resolve the component doing the work from the child container explicitly, so all of its dependencies follow
var thing = childScope.Resolve<ThingThatDoesStuff>();
thing.Do(request);
}
}
catch (Exception ex)
{
}
}
该问题的所有答案现在都已过时。 使用最新的包,您可以轻松地立即获得构造函数注入。 只需要两步:
在非静态类中创建事件处理函数作为实例方法。 让我们将类QueueFunctions
。
将您的课程添加到服务列表中。
builder.ConfigureServices(services => { // Add // dependencies // here services.AddScoped<QueueFunctions>(); });
现在,您将能够通过构造函数注入依赖项。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.