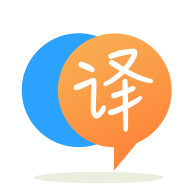
[英]How to compare user input to a file using a user.&pass. system while in a temporary loop for 3 times in C
[英]how to compare user input to a string for a while loop in c programming
我们正在尝试根据用户输入的内容执行if语句。 例如,如果用户输入Yen,那么我们希望执行if语句并计算到JPY的转换。 在用户输入了多少加仑气体后,便进行了计算,并剩下了总成本。 然后,我们要让用户选择他们想要将总成本转换成的货币。 我们给了用户三个选择。 如果用户输入的东西不是给定选项中的任何一个,则允许用户再次重新输入一个选项。 一个用户确实输入了三个选项之一,然后我们希望执行货币转换。
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <math.h>
#include <string.h>
#include <stdbool.h>
float gas_gallons;
float cost_today_gallons;
int main()
{
char input;
printf("Please enter the number of gallons of gasoline: ");
scanf("%c", &input);
while (!isdigit(input))
{
printf("\nYou need to enter a digit. Please enter the number of gallons of gasoline: ");
scanf("%c", &input);
}
if (isdigit(input))
{
printf("\nThe users input was %c", input);
gas_gallons = input - '0';
printf("\nAs a float it is now %f", gas_gallons);
float carbon_dioxide_pounds = gas_gallons * 19.64;
printf("\n%.2f gallons of gasoline produces approximately %f pounds of carbon dioxide.", gas_gallons, carbon_dioxide_pounds );
float barrels_crude_oil = gas_gallons/19.0;
printf("\n%.2f gallons of gasoline requires %f barrels of crude oil.", gas_gallons, barrels_crude_oil);
cost_today_gallons = gas_gallons*2.738;
printf("\n%.2f gallons of gasoline costs a total average of %f US dollars today.", gas_gallons, cost_today_gallons);
}
char currency[100];
printf("\nChoose a currency you want to see your total cost in (Euro, Pound, or Yen): ");
scanf("%c", ¤cy);
printf("\nThe currency chosen is %c", currency);
char Yen[100];
char Euro[100];
char Pound[100];
while (strcmp(currency, Yen) != 0 || strcmp(currency, Euro) != 0 || strcmp(currency, Pound) != 0)
{
printf("\nYou need choose one of these currencies (Euro, Pound or Yen). Please enter one: ");
scanf("%s", ¤cy);
}
if (strcmp(currency, Yen) == 1)
{
float yen_total_cost = cost_today_gallons*123.07;
printf("\n%.2f gallons of gasoline costs a total average of %f US dollars today.", gas_gallons, yen_total_cost);
}
if (strcmp(currency, Euro) == 1)
{
float euro_total_cost = cost_today_gallons*0.92;
printf("\n%.2f gallons of gasoline costs a total average of %f US dollars today.", gas_gallons, euro_total_cost);
}
if (strcmp(currency, Pound) == 1)
{
float pound_total_cost = cost_today_gallons*0.65;
printf("\n%.2f gallons of gasoline costs a total average of %f US dollars today.", gas_gallons, pound_total_cost);
}
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.