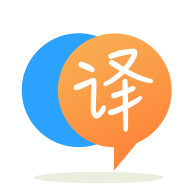
[英]Capture image from webcam and save in folder using PHP and JavaScript
[英]How to capture an image and save from webcam using grails?
尝试从网络摄像头捕获图像并希望保存在驱动器上
使用Grails 2.3.7
脚本代码
var video = document.querySelector("#videoElement");
var imageW;
//check for getUserMedia support
navigator.getUserMedia = navigator.getUserMedia
|| navigator.webkitGetUserMedia
|| navigator.mozGetUserMedia
|| navigator.msGetUserMedia || navigator.oGetUserMedia;
if (navigator.getUserMedia) {
// get webcam feed if available
navigator.getUserMedia({
video : true
}, handleVideo, videoError);
}
function handleVideo(stream) {
video.src = window.URL.createObjectURL(stream);
}
function videoError(e) {
}
var v, canvas, context, w, h;
var imgtag = document.getElementById('imgtag');
var sel = document.getElementById('"avatar"');
document.addEventListener('DOMContentLoaded', function() {
v = document.getElementById('videoElement');
canvas = document.getElementById('canvas');
context = canvas.getContext('2d');
w = canvas.width;
h = canvas.height;
}, false);
function draw(v, c, w, h) {
if (v.paused || v.ended)
return false; // if no video, exit here
context.drawImage(v, 0, 0, w, h); // draw video feed to canvas
var uri = canvas.toDataURL("image/png"); // convert canvas to data URI
imageW = canvas.toDataURL("image/png");
imageW = imageW.replace('data:image/png;base64,', '');
imgtag.src = uri;
}
document.getElementById('save').addEventListener('click',
function(e) {
draw(v, context, w, h);
});
var fr;
sel.addEventListener('change', function(e) {
var f = sel.files[0];
fr = new FileReader();
fr.onload = receivedData;
fr.readAsDataURL(f);
})
function receivedData() {
imgtag.src = fr.result;
}
function webImageSubmit() {
alert(imageW);
var pars = "id=" + $('#id').val() + "&imageW=" + imageW;
$.ajax({
type : 'POST',
url : '/controller_name/saveWebCamImage',
data : pars,
error : function(request, status, error) {
document.getElementById('load').style.visibility = "hidden";
},
beforeSend : function() {
document.getElementById('load').style.visibility = "visible";
},
success : function(data) {
location.reload();
}
});
}
控制器端:
def encodedData = javax.xml.bind.DatatypeConverter.parseBase64Binary(params.imageW.toString());
def defaultPath = "/images/userImages";
def webRootDir = servletContext.getRealPath("/");
def systemDir = new File(webRootDir, defaultPath);
if (!systemDir.exists()) {
systemDir.mkdirs();
}
String file_name = "webImage.jpg";
def csvFileDir = new File( systemDir, file_name);
new File(systemDir, file_name).withOutputStream {
it.write(encodedData);
};
但是图像未保存在驱动器上。
请帮助我..谢谢..
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script>
$(document).ready(function(){
const vid = document.querySelector('video');
navigator.mediaDevices.getUserMedia({video: true}) // request cam
.then(stream => {
vid.srcObject = stream; // don't use createObjectURL(MediaStream)
return vid.play(); // returns a Promise
})
.then(()=>{ // enable the button
const btn = document.querySelector('button');
btn.disabled = false;
btn.onclick = e => {
takeASnap()
.then(download);
};
});
function takeASnap(){
const canvas = document.createElement('canvas'); // create a canvas
const ctx = canvas.getContext('2d'); // get its context
canvas.width = vid.videoWidth; // set its size to the one of the video
canvas.height = vid.videoHeight;
ctx.drawImage(vid, 0,0); // the video
return new Promise((res, rej)=>{
canvas.toBlob(res, 'image/jpeg'); // request a Blob from the canvas
});
}
function download(blob){
// uses the <a download> to download a Blob
let a = document.createElement('a');
a.href = URL.createObjectURL(blob);
a.download = 'screenshot.jpg';
document.body.appendChild(a);
a.click();
}
});
</script>
</head>
<body>
<button disabled>
take a snapshot</button>
<video></video>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<button disabled>start recording</button>
<video></video>
<script>
const vid = document.querySelector('video');
navigator.mediaDevices.getUserMedia({video: true}) // request cam
.then(stream => {
vid.srcObject = stream; // don't use createObjectURL(MediaStream)
return vid.play(); // returns a Promise
})
.then(()=>{ // enable the button
const btn = document.querySelector('button');
btn.disabled = false;
btn.onclick = startRecording;
});
function startRecording(){
// switch button's behavior
const btn = this;
btn.textContent = 'stop recording';
btn.onclick = stopRecording;
const chunks = []; // here we will save all video data
const rec = new MediaRecorder(vid.srcObject);
// this event contains our data
rec.ondataavailable = e => chunks.push(e.data);
// when done, concatenate our chunks in a single Blob
rec.onstop = e => download(new Blob(chunks));
rec.start();
function stopRecording(){
rec.stop();
// switch button's behavior
btn.textContent = 'start recording';
btn.onclick = startRecording;
}
}
function download(blob){
// uses the <a download> to download a Blob
let a = document.createElement('a');
a.href = URL.createObjectURL(blob);
a.download = 'recorded.webm';
document.body.appendChild(a);
a.click();
}
</script>
</body>
</html>
<!doctype html>
<html lang="en">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>WebcamJS Test Page</title>
<style type="text/css">
body { font-family: Helvetica, sans-serif; }
h2, h3 { margin-top:0; }
form { margin-top: 15px; }
form input { margin-right: 15px; }
#results { float:right; margin:20px; padding:20px; border:1px solid; background:#ccc; }
</style>
</head>
<body>
<div id="results">Your captured image will appear here...</div>
<h1>WebcamJS Test Page</h1>
<h3>Demonstrates 320x240 capture & display with preview mode</h3>
<div id="my_camera"></div>
<!-- First, include the Webcam.js JavaScript Library -->
<script type="text/javascript" src="webcam.min.js"></script>
<!-- Configure a few settings and attach camera -->
<script language="JavaScript">
Webcam.set({
width: 320,
height: 240,
image_format: 'jpeg',
jpeg_quality: 90
});
Webcam.attach( '#my_camera' );
</script>
<!-- A button for taking snaps -->
<form>
<div id="pre_take_buttons">
<input type=button value="Take Snapshot" onClick="preview_snapshot()">
</div>
<div id="post_take_buttons" style="display:none">
<input type=button value="< Take Another" onClick="cancel_preview()">
<input type=button value="Save Photo >" onClick="save_photo()" style="font-weight:bold;">
</div>
</form>
<!-- Code to handle taking the snapshot and displaying it locally -->
<script language="JavaScript">
function preview_snapshot() {
// freeze camera so user can preview pic
Webcam.freeze();
// swap button sets
document.getElementById('pre_take_buttons').style.display = 'none';
document.getElementById('post_take_buttons').style.display = '';
}
function cancel_preview() {
// cancel preview freeze and return to live camera feed
Webcam.unfreeze();
// swap buttons back
document.getElementById('pre_take_buttons').style.display = '';
document.getElementById('post_take_buttons').style.display = 'none';
}
function save_photo() {
// actually snap photo (from preview freeze) and display it
Webcam.snap( function(data_uri) {
// display results in page
document.getElementById('results').innerHTML =
'<h2>Here is your image:</h2>' +
'<img src="'+data_uri+'"/>';
// swap buttons back
document.getElementById('pre_take_buttons').style.display = '';
document.getElementById('post_take_buttons').style.display = 'none';
} );
}
</script>
</body>
</html>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.