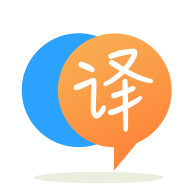
[英]How to pass parameters on onChange of html select with flask-wtf
[英]How to submit a Flask-WTF form with Ajax
我希望能够采用这种形式-通过条纹签出获得条纹ID(代码已实现且正在运行),然后通过ajax提交表单,并将条纹ID插入表单的隐藏值中。
什么jQuery代码可以让我做到这一点?
class signupForm(Form):
forename = StringField('Forename', validators = [ Required()])
surname = StringField('Surname', validators = [Required()])
username = StringField('Username', validators = [Required(), Length(min = 4, max = 20)])
password1 = PasswordField('Password', validators = [Required(), Length(min = 6, max=50)])
password2 = PasswordField('Password', validators = [Required(), Length(min = 6, max=50)])
email = StringField('Email', validators = [Email(), Required()])
phone = StringField('Phone number', validators = [Required()])
addressLine1 = StringField('Address Line 1', validators = [Required()])
addressLine2 = StringField('Address Line 2')
town = StringField('Town', validators = [Required()])
county = StringField('County', validators = [Required()])
postcode = StringField('Postcode', validators = [Required()])
recurringDonationQuantity = StringField('If you would like to make a monthly recurring donation, please enter the amount in Sterling below. <br> Recurring Donation Amount')
stripetoken = HiddenField('')
我的页面:
{% with messages = get_flashed_messages() %}
{% if messages %}
<div class="alert alert-warning" role="alert">
<ul>
{% for message in messages %}
<li>{{ message | safe }}</li>
{% endfor %}
</ul>
</div>
{% endif %}
{% endwith %}
{{ wtf.quick_form(form) }}
我也有此javascrpt页内
<script>
var handler = StripeCheckout.configure({
key: '{{key}}',
image: '{{ url_for("static", filename="logo.png") }}',
locale: 'english',
token: function(token,args) {
$('#stripe-token').val = token;
wt
console.log(token)
}
});
$('#pay').on('click', function(e) {
// Open Checkout with further options
if ('{{type}}' == 'normal') {
description = 'Normal Membership';
amount = 2500;
console.log('membership type is NORMAL')
} else {
description = 'Associate Membership';
amount = 2500;
console.log('membership type is ASSOCIATE')
}
handler.open({
name: 'My Organisation',
description: description,
amount: amount,
currency: 'GBP',
panelLabel: 'Okay',
billingAddress: true,
zipCode: true
});
e.preventDefault();
});
// Close Checkout on page navigation
$(window).on('popstate', function() {
handler.close();
});
</script>
我可能会使用jQuery的serialize
方法尝试类似的方法。
<script>
$(document).ready(function(){
$('#submit').click(function() {
console.log('submit clicked');
//This part keeps you D.R.Y.
url_params = $(this).serialize();
//left this code the same...
var csrftoken = $('meta[name=csrf-token]').attr('content')
$.ajaxSetup({
beforeSend: function(xhr, settings) {
if (!/^(GET|HEAD|OPTIONS|TRACE)$/i.test(settings.type) && !this.crossDomain) {
xhr.setRequestHeader("X-CSRFToken", csrftoken)
}
}
})
$.ajax({
//more changes here
url: url_params, //you may need to modify this to hit the
//correct URL depending on your forms action param
//ex: url: $(this).attr('action') + url_params,
type: 'GET', //GET instead of POST so we can use url_params
contentType:'application/json;charset=UTF-8',
success: function(response) {
console.log(response);
},
error: function(error) {
console.log(error);
}
});
});
});
</script>
希望这可以为您指明正确的方向,从而避免将太多字段硬编码到您的JavaScript中。
您也可以选择将ajax调用保留为'POST'
,如果您想推出自己的解决方案,则需要看一下jQuery的serializeArray
,或者请参阅我根据以下stackoverflow问题改编的以下代码:
// add a helper function to the $ jQuery object.
// this can be included in your page or hosted in a separate JS file.
$.fn.serializeObject = function()
{
var o = {};
var a = this.serializeArray();
$.each(a, function() {
if (o[this.name] !== undefined) {
if (!o[this.name].push) {
o[this.name] = [o[this.name]];
}
o[this.name].push(this.value || '');
} else {
o[this.name] = this.value || '';
}
});
return o;
};
// then inside your form page:
<script>
$(document).ready(function(){
$('#submit').click(function() {
console.log('submit clicked');
//This part keeps you D.R.Y.
data = $(this).serializeObject();
//left this code the same...
var csrftoken = $('meta[name=csrf-token]').attr('content')
$.ajaxSetup({
beforeSend: function(xhr, settings) {
if (!/^(GET|HEAD|OPTIONS|TRACE)$/i.test(settings.type) && !this.crossDomain) {
xhr.setRequestHeader("X-CSRFToken", csrftoken)
}
}
})
$.ajax({
//more changes here
data: data,
url: '',
contentType:'application/json;charset=UTF-8',
success: function(response) {
console.log(response);
},
error: function(error) {
console.log(error);
}
});
});
});
</script>
这里要摘录的关键是您可以使用javascript或jQuery读取<form>
数据并将其参数化。 您要尽可能避免对帖子data
硬编码。 这样做很麻烦,而将其更改为下一个路线则更大。
最后,我这样做是希望有一种更简单的方法:
<script>
$(document).ready(function(){
$('#submit').click(function() {
console.log('submit clicked')
data = {
'csrf_token': '{{ csrf_token() }}',
'csrftoken': '{{ csrf_token() }}',
'stripetoken': gtoken,
'forename': $('#forename').val(),
'surname': $('#surname').val(),
'username': $('#username').val(),
'password1': $('#password1').val(),
'password2': $('#password2').val(),
'email': $('#email').val(),
'phone': $('#phone').val(),
'addressLine1': $('#addressLine1').val(),
'addressLine2': $('#addressLine2').val(),
'town': $('#town').val(),
'county': $('#county').val(),
'postcode': $('#postcode').val(),
'recurringDonationQuantity': $('#recurringDonationQuantity').val(),
}
var csrftoken = $('meta[name=csrf-token]').attr('content')
$.ajaxSetup({
beforeSend: function(xhr, settings) {
if (!/^(GET|HEAD|OPTIONS|TRACE)$/i.test(settings.type) && !this.crossDomain) {
xhr.setRequestHeader("X-CSRFToken", csrftoken)
}
}
})
$.ajax({
url: '',
data: JSON.stringify(data, null, '\t'),
type: 'POST',
contentType:'application/json;charset=UTF-8',
success: function(response) {
console.log(response);
},
error: function(error) {
console.log(error);
}
});
});
});
</script>
csrf的事情太多了,因为我还没弄清楚哪一个使它起作用。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.