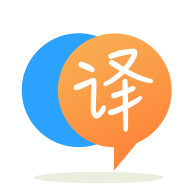
[英]How to create a container that holds different types of function pointers in C++?
[英]How to create a queue of function pointers in C++
我正在尝试使用C ++创建一个合作的调度程序,为此我需要一个包含函数指针的队列。
在这种情况下,C ++队列STL库是否有帮助?
这显然是std::queue
旨在帮助解决的确切问题。
我会改变一些事情。 一种方法是存储std::function
而不是指向函数的原始指针:
struct task {
int id;
std::function<void()> f;
};
这使您可以传递基本上可以像函数一样调用的所有内容,而不仅仅是指向实际函数的指针。 举一个显而易见的例子,您可以使用lambda表达式:
task t { 1, [] {cout << "having fun!\n"; } };
q.push(t);
auto tsk = q.front();
tsk.f();
由于您几乎只能对任务进行处理,因此我还要考虑为task
提供重载的operator()
来进行调用: void operator()() { f(); }
void operator()() { f(); }
,因此,如果您只想调用任务,则可以执行以下操作:
auto f = q.front();
f();
您的测试程序已扩展为包括以下内容:
#include <iostream>
#include <queue>
#include <functional>
using namespace std;
struct task {
int id;
std::function<void()> f;
void operator()() { f(); }
};
queue<struct task> q;
void fun(void) {
cout << "Having fun!" << endl;
}
int main() {
cout << "Creating a task object" << endl;
task t;
t.id = 1;
t.f = &fun;
cout << "Calling function directly from object" << endl;
t.f();
cout << "adding the task into the queue" << endl;
q.push(t);
cout << "calling the function from the queue" << endl;
task tsk = q.front();
tsk.f();
q.pop();
q.push({ 1, [] {std::cout << "Even more fun\n"; } });
auto t2 = q.front();
t2.f(); // invoke conventionally
t2(); // invoke via operator()
q.pop();
}
似乎我找到了一种使用结构来实现功能队列的简单方法。 可能不是完美的或高效的,目前就行了。
#include <iostream>
#include <queue>
using namespace std;
struct task {
int id;
void (*fptr) (void);
};
queue<struct task> q;
void fun(void) {
cout<<"Having fun!"<<endl;
}
int main() {
cout<<"Creating a task object"<<endl;
task t;
t.id = 1;
t.fptr = &fun;
cout<<"Calling function directly from object"<<endl;
t.fptr();
cout << "adding the task into the queue"<<endl;
q.push(t);
cout << "calling the function from the queue"<<endl;
task tsk = q.front();
tsk.fptr();
q.pop();
return 0;
}
OUTPUT :
Creating a task object
Calling function directly from object
Having fun!
adding the task into the queue
calling the function from the queue
Having fun!
我不会使用std :: queue格式中描述的队列。
它不允许您在任何给定位置插入。 您应该建立自己的。
如果像下面这样操作并添加优先级和名称,那么您可以轻松地提出逻辑来切换任务,切换/更新优先级,甚至插入所需的位置。
我会说链表总是有速度问题。 您还可以对结构数组执行类似的操作,并使用数组逻辑来移动和插入。
struct function_T {
string name;
uint8_t priority;
void(*func_ptr)(void);
};
struct node_T {
node_T * previous = NULL;
function_T fptr;
node_T * next;
};
int main(void){
function_T task1;
task1.name = "task1";
task1.priority = 1;
task1.func_ptr = func4;
node_T first_node;
first_node.previous = NULL;
first_node.fptr = task1;
first_node.next = NULL;
first_node.fptr.func_ptr();
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.