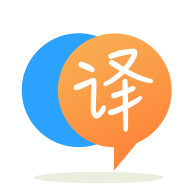
[英]How can I bind data to two complex type parameters of Action method by Ajax POST
[英]How can I AJAX POST this complex type to my MVC action?
我试图将复杂数据类型POST到MVC控制器操作,但数据为null或包含默认值。
这是客户端(它将type
参数设置为data.complexType
[例如, type: data.complexType
]并将其发布):
这是POST操作的问题:
它从GET中获得了很好的复杂类型,但是现在我怎样才能将相同的复杂类型POST回控制器,使其不再包含null值或默认值?
这是我的HomeController.cs
:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace WebApplication0.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
[HttpPost]
public ActionResult PostComplexType(ComplexType type)
{
return Json(new { complexType = type });
}
public ActionResult GetComplexType()
{
var result = new ComplexType
{
TypeOne = 1,
TypeTwo = "2",
TypeThree = new ComplexNestedType
{
TypeOne = 1,
TypeTwo = "2"
}
};
return Json(new { complexType = result }, JsonRequestBehavior.AllowGet);
}
}
public class ComplexType
{
public Int32 TypeOne { get; set; }
public String TypeTwo { get; set; }
public ComplexNestedType TypeThree { get; set; }
}
public class ComplexNestedType
{
public Int32 TypeOne { get; set; }
public String TypeTwo { get; set; }
}
}
这是我的Index.cshtml
:
@{
ViewBag.Title = "Index";
Layout = "~/Views/Shared/_Layout.cshtml";
}
@section scripts {
<script>
$("#exampleButton").click(function () {
$.ajax({
url: "@Url.Action("GetComplexType", "Home")",
type: "get",
error: function () {
alert("There was an error calling GetComplexType().")
},
success: function (data) {
debugger;
$.ajax({
url: "@Url.Action("PostComplexType", "Home")",
type: "post",
data: {
type: data.complexType
},
error: function () {
alert("There was an error calling PostComplexType().")
},
success: function (data) {
debugger;
}
});
}
});
});
</script>
}
<button id="exampleButton" type="button">Example</button>
编辑 :我尝试直接设置data: data.complexType
,但它不会解析嵌套类型:
你可以使用JSON.stringify
$.ajax({
url: "@Url.Action("PostComplexType", "Home")",
type: "post",
data: JSON.stringify(data.complexType),
contentType: "application/json; charset=UTF-8",
error: function () {
alert("There was an error calling PostComplexType().")
},
success: function (data) {
debugger;
}
});
这是另一种方法。 大多数情况下,我们必须使用不同控件中的值来填充这些类型。 这种方法可以很自由地使用这些类型执行您想要的操作。
<script type="text/javascript">
jQuery(document).ready(function ($) {
var complexNestedType = {TypeOne:1, TypeTwo:'2'}
var complexType = { TypeOne: 3, TypeTwo: '4', TypeThree: complexNestedType };
var data = { type: complexType };
var posting = $.post("/Home/PostComplexType", data, function () {
});
posting.done(function (response) {
console.log(response);
if (response != null) {
alert('success');
} else{
alert('failed');
}
});
});
</script>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.