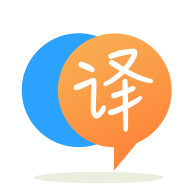
[英]Find a specified generic DbSet in a DbContext dynamically when I have an entity
[英]Find a generic DbSet in a DbContext dynamically
我知道这个问题已经被问过,但我找不到令我满意的答案。 我想要做的是根据其类型名称检索特定的DbSet<T>
。
我有以下内容:
[assembly: System.Runtime.CompilerServices.InternalsVisibleTo("MyDllAssemblyName")]
[assembly: System.Runtime.CompilerServices.InternalsVisibleTo("MyCallingAssemblyName")]
class MyDbContext : DbContext {
public DbSet<ModelA> A { get; set; }
public DbSet<ModelB> B { get; set; }
public dynamic GetByName_SwitchTest(string name) {
switch (name) {
case "A": return A;
case "B": return B;
}
}
public dynamic GetByName_ReflectionTest(string fullname)
{
Type targetType = Type.GetType(fullname);
var model = GetType()
.GetRuntimeProperties()
.Where(o =>
o.PropertyType.IsGenericType &&
o.PropertyType.GetGenericTypeDefinition() == typeof(DbSet<>) &&
o.PropertyType.GenericTypeArguments.Contains(targetType))
.FirstOrDefault();
if (null != model)
return model.GetValue(this);
return null;
}
}
无论是通过简单的开关还是反射,我都可以轻松获取类型本身。 但是我需要将类型作为动态返回,因为我不知道它将是什么 DbSet 类型。 然后在同一个程序集中的其他地方,我这样使用它:
// MyDbContext MyDbContextInstance..
var model = MyDbContextInstance.GetByName_SwitchTest("A");
var record1 = model.FirstOrDefault(); // It crashes here with RunTimeBinderException
此时model
包含一个InternalDbSet<ModelA>
类型的实例。 从那里,我对model
object 的任何使用都会得到一个 RunTimeBinderException: 'Microsoft.Data.Entity.Internal.InternalDbSet' does not contain a definition for 'FirstOrDefault'
调查 web,我发现了一篇解释该内容的博文(引用他的博客):
调用 FirstOrDefault() 失败的原因是 model 的类型信息在运行时不可用。 它不可用的原因是匿名类型不公开。 当该方法返回该匿名类型的实例时,它返回一个 System.Object,它引用了一个匿名类型的实例——一种其信息对主程序不可用的类型。
然后他指出了一个解决方案:
解决方法其实很简单。 我们所要做的就是打开 ClassLibrary1 项目的 AssemplyInfo.cs 并向其中添加以下行:
[assembly:InternalsVisibleTo("assembly-name")]
我确实在我的代码上尝试过这个解决方案,但它不起作用。 有关信息,我有一个 asp.net 5 解决方案,其中两个程序集在 dnx do.net46 上运行。 一个应用程序和一个包含我所有模型和 DbContext 的 dll。 不过,我所做的所有相关电话都位于 dll 上。
这个解决方案有机会工作吗? 我错过了什么吗? 任何指针将不胜感激?
提前致谢
[编辑]
我试图返回IQueryable<dynamic>
而不是dynamic
并且我可以执行基本查询model.FirstOrDefault();
但最重要的是,我也希望能够在一个字段上进行过滤:
var record = model.FirstOrDefault(item => item.MyProperty == true);
*免责声明:此回复并未对我的问题给出严格意义上的答案。 这是解决我自己的问题的另一种方法。 我知道这是针对特定情况的特定示例,它不适用于所有人。 我发布这种方法是希望它对某人有所帮助,但不会将其标记为答案,因为我仍然希望有一个真正的解决方案。
首先,让我们接受这样一个事实,即我们可以从当前代码中获得的唯一有用信息是记录是否存在。此后任何动态查询尝试都会引发 RuntimeBinderException。
然后让我们继续另一个事实; DbContext.Add(object) 和 DbContext.Update(object) 不是基于模板的,因此我们可以使用它们来保存我们的模型(而不是 db.A.Add() 或 db.A.Update() )
在我自己的情况下,不再需要制定程序
首先,我需要一个可以在我的所有模型中检索的字段,这显然应该是一种识别唯一记录的方法。
// IModel give me a reliable common field to all my models ( Fits my DB design maybe not yours though )
interface IModel { Guid Id { get; set; } }
// ModelA inherit IModel so that I always have access to an 'Id'
class ModelA : IModel {
public Guid Id { get; set; }
public int OtherField { get; set; }
}
// ModelB inherit IModel so that I always have access to an 'Id'
class ModelB : IModel {
public Guid Id { get; set; }
public string WhateverOtherField { get; set; }
}
我还没有找到一种动态进行智能查询的方法,所以我知道我可以可靠地识别一条记录并知道它是否存在。
class MyDbContext : DbContext {
public DbSet<ModelA> A { get; set; }
public DbSet<ModelB> B { get; set; }
// In my case, this method help me to know the next action I need to do
// The switch/case option is not pretty but might have better performance
// than Reflection. Anyhow, this is one's choice.
public bool HasRecord_SwitchTest(string name) {
switch (name) {
case "A": return A.AsNoTracking().Any(o => o.Id == id);
case "B": return B.AsNoTracking().Any(o => o.Id == id);
}
return false;
}
// In my case, this method help me to know the next action I need to do
public bool HasRecord_ReflectionTest(string fullname)
{
Type targetType = Type.GetType(fullname);
var model = GetType()
.GetRuntimeProperties()
.Where(o =>
o.PropertyType.IsGenericType &&
o.PropertyType.GetGenericTypeDefinition() == typeof(DbSet<>) &&
o.PropertyType.GenericTypeArguments.Contains(targetType))
.FirstOrDefault();
if (null != model)
return (bool)model.GetValue(this).AsNoTracking().Any(o => o.Id == id);
return false;
}
// Update and save immediately - simplified for example
public async Task<bool> UpdateDynamic(object content)
{
EntityEntry entry = Update(content, GraphBehavior.SingleObject);
return 1 == await SaveChangesAsync(true);
}
// Insert and save immediately - simplified for example
public async Task<bool> InsertDynamic(object content)
{
EntityEntry entry = Add(content, GraphBehavior.SingleObject);
return 1 == await SaveChangesAsync(true);
}
}
接下来,我需要对动态查询做的是一种将数据从服务器复制到客户端的方法。 (为了简化这个例子,我省略了大部分架构)
class ReplicationItem
{
public ReplicationAction Action { get; set; } // = Create, Update, Delete
public string ModelName { get; set; } // Model name
public Guid Id { get; set; } // Unique identified across whole platform
}
现在,这是连接位的例程
public async void ProcessReplicationItem(ReplicationItem replicationItem)
{
using (var db = new MyDbContext())
{
// Custom method that attempts to get remote value by Model Name and Id
// This is where I get the strongly typed object
var remoteRecord = await TryGetAsync(replicationItem.ModelName, replicationItem.Id);
bool hasRemoteRecord = remoteRecord.Content != null;
// Get to know if a local copy of this record exists.
bool hasLocalRecord = db.HasRecord_ReflectionTest(replicationItem.ModelName, replicationItem.Id);
// Ensure response is valid whether it is a successful get or error is meaningful ( ie. NotFound )
if (remoteRecord.Success || remoteRecord.ResponseCode == System.Net.HttpStatusCode.NotFound)
{
switch (replicationItem.Action)
{
case ReplicationAction.Create:
{
if (hasRemoteRecord)
{
if (hasLocalRecord)
await db.UpdateDynamic(remoteRecord.Content);
else
await db.InsertDynamic(remoteRecord.Content);
}
// else - Do nothing
break;
}
case ReplicationAction.Update:
[etc...]
}
}
}
}
// Get record from server and with 'response.Content.ReadAsAsync' type it
// already to the appropriately
public static async Task<Response> TryGetAsync(ReplicationItem item)
{
if (string.IsNullOrWhiteSpace(item.ModelName))
{
throw new ArgumentException("Missing a model name", nameof(item));
}
if (item.Id == Guid.Empty)
{
throw new ArgumentException("Missing a primary key", nameof(item));
}
// This black box, just extrapolate a uri based on model name and id
// typically "api/ModelA/{the-guid}"
string uri = GetPathFromMessage(item);
using (var client = new HttpClient())
{
client.BaseAddress = new Uri("http://localhost:12345");
HttpResponseMessage response = await client.GetAsync(uri);
if (response.IsSuccessStatusCode)
{
return new Response()
{
Content = await response.Content.ReadAsAsync(Type.GetType(item.ModelName)),
Success = true,
ResponseCode = response.StatusCode
};
}
else
{
return new Response()
{
Success = false,
ResponseCode = response.StatusCode
};
}
}
}
public class Response
{
public object Content { get; set; }
public bool Success { get; set; }
public HttpStatusCode ResponseCode { get; set; }
}
ps:我仍然对真实答案感兴趣,所以如果您有真实的答案可以分享,请继续发布其他答案。
那么,当我在编译期间不知道<T>
时,我是如何做到的。
首先需要获取类型,因为DbContext.Set方法返回一个非泛型 DbSet 实例,用于访问上下文和底层存储中给定类型的实体。
public virtual DbSet Set(Type entityType)
注意这里的参数是应该返回集合的实体类型。给定实体类型的集合是返回值。
var type = Assembly.GetExecutingAssembly().GetTypes().FirstOrDefault(t => t.Name == <Pass your table name>);
现在一旦我有了这种类型
if(type != null)
{
DbSet context = context.Set(type);
}
或者一个班轮将是
DbSet mySet = context.Set(Type.GetType("<Your Entity Name>"));
您可以使用它来获取特定类型的 DBSet:
public object GetByType(DbContextcontext, Type type) {
var methode = _context.GetType().GetMethod("Set", types: Type.EmptyTypes);
if (methode == null) {
return null;
}
return methode.MakeGenericMethod(type).Invoke(_context, null);
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.