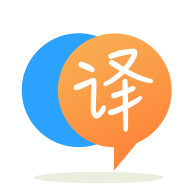
[英]Terminate function call after a certain amount of time has elapsed in Python
[英]How to terminate a thread in python after a certain amount of time?
我有多个线程运行while循环。 我想在给定的时间后终止这些线程。 我知道与此类似的其他问题,但是我看不到如何将这些答案转移到我的代码中。
def function1(arg1, arg2, arg3, duration):
t_end = time.time() + duration
while time.time() < t_end:
#do some stuff
for i in range(100):
t = Thread(target = function1, args=(arg1, arg2, arg3, 10))
t.start()
这将打开100个线程,但它们永远不会关闭。 在指定的时间(本示例中为10秒)之后,如何关闭这些线程? 我的函数打开一个套接字。
混合使用终止线程(可在此处找到信息: 有什么方法可以杀死Python中的线程吗? )
和线程计时器对象: https : //docs.python.org/2/library/threading.html#timer-objects
下面的代码对我TypeError
,但是不断抛出TypeError
的事实使我感到困惑。 我似乎找不到太多有关为何发生或如何预防的信息:
threadingtest.py
#!/usr/bin/env python3
import time
import threading
class StoppableThread(threading.Thread):
"""Thread class with a stop() method. The thread itself has to check
regularly for the stopped() condition."""
def __init__(self):
super(StoppableThread, self).__init__()
self._stop = threading.Event()
def stop(self):
self._stop.set()
try:
self.join()
except TypeError as tE:
print("Shutting down")
def stopped(self):
return self._stop.isSet()
class MyStoppableThread(StoppableThread):
def __init__(self, *args):
super(MyStoppableThread, self).__init__()
self.args = args # Use these in the thread
def run(self):
print("Started my thread with arguments {}".format(self.args))
while not self.stopped():
time.sleep(1)
# THIS IS WHERE YOU DO THINGS
if __name__ == "__main__":
threads = []
for i in range(100):
t = MyStoppableThread(i, 'a', 'b', 'c')
t.start()
threads.append(t)
print("\n:: all threads created\n")
time.sleep(5)
print("\n:: killing all threads\n");
for t in threads:
t.stop()
您可以将回调传递给每个线程。 并创建一个线程列表。
threadlist = {}
def cb(id, currtime):
t = threadlist[id]
d = currtime - t.starttime
if d > 10:
return True
else:
return False
def function1(arg1, arg2, arg3, duration, cb, threadid):
t_end = time.time() + duration
while time.time() < t_end:
#do some stuff
if cb(threadid, time.time()):
break
for i in range(100):
t = Thread(target = function1, args=(arg1, arg2, arg3, 10, cb, i))
threadlist[id] = {"starttime": time.time(), "thread": t}
t.start()
并检查:
time.sleep(15)
for item in threadlist.values():
print(item.thread.is_alive())
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.