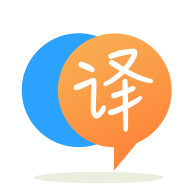
[英]In C++ is it possible to return an element of the class from a class member function?
[英]Return object from class member function in C++
上面的代码中的任务是编写计算新点的成员函数,该点是另外两个点的数量。 而且我不知道如何返回对象或该怎么办。 这是代码,该函数标有三个!!!。 该函数必须返回某些内容,我不能使其无效,因为不允许对void的引用。
class point {
private:
float x;
float y;
public:
point();
point(float xcoord, float ycoord);
void print();
float dist(point p1, point p2);
!!! float &add(point p1, point p2);
float &X();
float &Y();
~point();
};
float & point::X() { return x; }
float & point::Y() { return y; }
point::point() {
cout << "Creating POINT (0,0)" << endl;
x = y = 0.0;
}
point::point(float xcoord, float ycoord) {
cout << "Creating POINt (" << xcoord << "," << ycoord << ")" << endl;
x = xcoord;
y = ycoord;
}
void point::print() {
cout << "POINT (" << x << "," << y << ")";
}
float point::dist(point p1, point p2) {
return sqrt((p1.x - p2.x)*(p1.x - p2.x) + (p1.y - p2.y)*(p1.y - p2.y));
}
!!!// float & point::add(point p1, point p2) {
point z;
z.X() = p1.X() + p2.X();
z.Y() = p1.Y() + p2.Y();
z.print();
}
point::~point() {
cout << "Deleting ";
print();
cout << endl;
}
int main() {
point a(3, 4), b(10, 4);
cout << "Distance between"; a.print();
cout << " and "; b.print();
cout << " is " << a.dist(a, b) << endl;
}
我做到了! 这是必须添加的功能
//prototype
point &add(point& p1, point& p2);
//function itself
point & point::add(point& p1, point& p2) {
point z;
z.x = p1.X() + p2.X();
z.y = p1.Y() + p2.Y();
z.print();
return z;
}
非常感谢ForceBru! 和你们所有人
您也可以返回一个point
:
point point::add(const point& p1, const point& p2) {
point result;
result.x = p1.x + p2.x;
result.y = p1.y + p2.y;
return result;
}
请注意,此处不需要使用X()
和Y()
函数,因为此方法已经可以访问私有成员。
也可以进行操作员重载
/*friend*/ point operator+ (const point& one, const point& two) {
// the code is the same
}
int main() {
point one(2,5), two(3,6), three;
three.add(one, two);
std::cout << "Added " << one.print() << " and " << two.print();
std::cout << " and got " << three.print() << std::endl;
return 0;
}
编辑 :正如评论中所说,您不应返回对在add
函数内创建的对象的引用,因为在这种情况下,您只能返回对类成员和static
变量的引用。
您可以在此处使用运算符重载:
point point::operator+(const point & obj) {
point obj3;
obj3.x = this->x + obj.x;
return obj3;
}
返回对象加上两点。
举个简单的例子:
class Addition {
int a;
public:
void SetValue(int x);
int GetValue();
Addition operator+(const Addition & obj1);
};
void Addition::SetValue(int x)
{
a = x;
}
int Addition::GetValue()
{
return a;
}
Addition Addition::operator+(const Addition &obj1)
{
Addition obj3;
obj3.a = this->a + obj1.a;
return obj3;
}
int _tmain(int argc, _TCHAR* argv[])
{
Addition obj1;
int Temp;
std::cout<<"Enter Value for First Object : "<<std::endl;
std::cin>>Temp;
obj1.SetValue(Temp);
Addition obj2;
std::cout<<"Enter Value for Second Object : "<<std::endl;
std::cin>>Temp;
obj2.SetValue(Temp);
Addition obj3;
obj3 = obj1 + obj2;
std::cout<<"Addition of point is "<<obj3.GetValue()<<std::endl;
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.