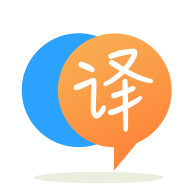
[英]C# How can I used a string to reference a pre-existing variable by the same name?
[英]How to pull a variable out of a string I need to compare to another, pre-existing string c#
我有一个规则引擎,该引擎将字符串作为规则的名称,并将其与字符串谓词字典进行比较。 我正在编写一个规则,该规则将比较两个日期时间,如果两个日期时间匹配,则返回true,但允许窗口的秒数可配置。 理想情况下,我希望我的字符串/谓词键值对看起来像
{"Allow <x> seconds", AllowXSeconds}
应用该规则的用户将决定他们希望在原始日期时间的任一侧有一个10秒的窗口,因此他们会在配置中说“允许10秒”。 我希望我的代码能够识别出用户想要应用“允许秒数”规则,然后拉出“ 10”,以便可以将其用作规则逻辑中的变量。 我宁愿不使用正则表达式,因为该类已经构建,并且我不知道是否可以这种方式对其进行重构。 但是,如果没有人对如何做到这一点有任何聪明的想法,我会做的。 在此先感谢所有聪明的人!
您可以使用string.StartsWith
和string.EndsWith
进行验证,然后使用string.Substring
获得所需的值,并使用int.TryParse
尝试解析该值并验证它是否为整数
string str = "Allow 10 seconds";
int result;
if (str.StartsWith("Allow ")
&& str.EndsWith(" seconds")
&& int.TryParse(str.Substring(6, str.Length - 14), out result))
{
Console.WriteLine(result);
}
else
{
Console.WriteLine("Invalid");
}
另外,如果需要,还可以使用StartsWith
和EndsWith
重载,从而也允许不区分大小写的匹配。
这看起来像是正则表达式的理想选择。
这是一个LINQPad程序,它演示:
void Main()
{
var lines = new[]
{
"Allow 10 seconds",
"Allow 5 seconds",
"Use 5mb"
};
var rules = new Rule[]
{
new Rule(
@"^Allow\s+(?<seconds>\d+)\s+seconds?$",
ma => AllowSeconds(int.Parse(ma.Groups["seconds"].Value)))
};
foreach (var line in lines)
{
bool wasMatched = rules.Any(rule => rule.Visit(line));
if (!wasMatched)
Console.WriteLine($"not matched: {line}");
}
}
public void AllowSeconds(int seconds)
{
Console.WriteLine($"allow: {seconds} second(s)");
}
public class Rule
{
public Rule(string pattern, Action<Match> action)
{
Pattern = pattern;
Action = action;
}
public string Pattern { get; }
public Action<Match> Action { get; }
public bool Visit(string line)
{
var match = Regex.Match(line, Pattern);
if (match.Success)
Action(match);
return match.Success;
}
}
输出:
allow: 10 second(s)
allow: 5 second(s)
not matched: Use 5mb
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.