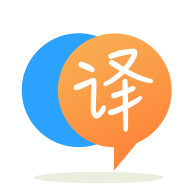
[英]Using subprocess to ping an address and get the average ping output in Python?
[英]Check if ping was successful using subprocess in python
我通过使用python的子进程模块的ping命令打开cmd window来在python中执行ping命令。
例如:
import subprocess
p = subprocess.Popen('ping 127.0.0.1')
之后,我检查 output 是否包含“来自‘ip’的回复:”,以查看 ping 是否成功。
这适用于 cmd 为英文的所有情况。
我如何检查 cmd 语言的 ping 是否成功?
在 Linux 上使用 python,我会使用 check_output()
subprocess.check_output(["ping", "-c", "1", "127.0.0.1"])
如果 ping 成功,这将返回 true
我知道这适用于 Linux,我认为它也适用于 Windows。
更新:未注释的代码也适用于 Windows
import subprocess
p = subprocess.Popen('ping 127.0.0.1')
# Linux Version p = subprocess.Popen(['ping','127.0.0.1','-c','1',"-W","2"])
# The -c means that the ping will stop afer 1 package is replied
# and the -W 2 is the timelimit
p.wait()
print p.poll()
如果 p.poll() 为 0,则 ping 成功,如果为 1,则无法到达目的地。
许多 IP 地址的版本将是:
import subprocess
iplist=["127.0.0.1","8.8.8.8"]
for ip in iplist:
p = subprocess.Popen('ping '+ip,stdout=subprocess.PIPE)
# the stdout=subprocess.PIPE will hide the output of the ping command
p.wait()
if p.poll():
print ip+" is down"
else:
print ip+" is up"
# You end with a log of all the ip addresses
对于窗户:
import subprocess
hostname = "10.20.16.30"
output = subprocess.Popen(["ping.exe",hostname],stdout =
subprocess.PIPE).communicate()[0]
print(output)
if ('unreachable' in output):
print("Offline")
@elfosardo 如果 ping 成功,您的解决方案不会返回 true。 如果返回代码非零,它会返回命令的输出或 CalledProcessError 异常。 按照您的建议使用 check_output() ,即使不是最好的解决方案,这里也是一个可能的解决方案:
import subprocess
def ping():
try:
subprocess.check_output(["ping", "-c", "1", "127.0.1.1"])
return True
except subprocess.CalledProcessError:
return False
适用于 Windows。
对于 Python 2
import ipaddress, subprocess
myIpAddress = raw_input('Enter an IP Address with a CIDR. >>> ') #192.168.0.1/24
myAdd = ipaddress.ip_interface(unicode(myIpAddress))
myNet = ipaddress.ip_network(myAdd, strict = False) # False lets you enter any ip address in the /24 ip address block.
for i in myNet:
canPing = subprocess.call('ping /n 2 /w 1000 %s' % str(i))
if canPing == 0:
print('I can ping %s' % str(i))
if canPing == 1:
print('%s is not responding' % str(i))
对于 Python 3
import ipaddress, subprocess
myIpAddress = input('Enter an IP Address with a CIDR >>> ') #192.168.0.1/24
myAdd = ipaddress.ip_interface(myIpAddress)
myNet = ipaddress.ip_network(myAdd, strict = False) # False lets you enter any ip address in the /24 ip address block.
for i in myNet:
canPing = subprocess.call('ping /n 2 /w 1000 %s' % str(i))
if canPing == 0:
print('I can ping %s.' % str(i))
if canPing == 1:
print('%s is not responding' % str(i))
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.