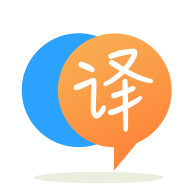
[英]How to use two functions that return different types as a function pointer in C?
[英]How to use pointer from 2 different functions in a third function in C
我正在尝试编写一个计算“图像”属性的程序。 “图像”是2的0和1的数组,0是空白,1是黑色像素。
我需要计算整齐度,仅是pixelHeight / pixelWidth,但是我无法正确获取指针的语法。 我想在我的第三个(高大)函数中调用前两个函数的指针。
#include <stdio.h>
#include <math.h>
#define MAX_X 20
#define MAX_Y 20
int countBlackPixels(int image[MAX_X][MAX_Y]);
int findYPixel(int image[MAX_X][MAX_Y]);
int findXPixel(int image[MAX_X][MAX_Y]);
double findTallness(int image[MAX_X][MAX_Y]);
//function prototypes here
void processImage(int image[MAX_X][MAX_Y]) {
int nBlackPixels;
nBlackPixels = countBlackPixels(image);
printf("Pixel-count: %d\n", nBlackPixels);
int *pixelHeight;
*pixelHeight = findYPixel(image);
printf("Height: %d\n", *pixelHeight);
int *pixelWidth;
*pixelWidth = findXPixel(image);
printf("Width: %d\n", *pixelWidth);
double tallness;
tallness = findTallness(tallness);
printf("Tallness: %.6lf\n", tallness);
}
//Count Black Pixels
int countBlackPixels(int image[MAX_X][MAX_Y]) {
int x, y, blackPixelCount;
blackPixelCount = 0;
x = 0;
while (x < MAX_X) {
y = 0;
while (y < MAX_X) {
if (image[x][y] == 1) {
blackPixelCount = blackPixelCount + 1;
//printf("black pixel at x=%d y=%d\n", x, y); // example debug printf
}
y = y + 1;
}
x = x + 1;
}
return blackPixelCount;
}
//Height
//Loops through from bottom-left to the right, and then up
//through the Y axis, find the last occurance of Y,
//adds one to overcome array's zero count, and
//returns this value as the pixel height
int * findYPixel(int image[MAX_X][MAX_Y]) {
int x, y, *pixelHeight;
y = 0;
*pixelHeight = -1;
while (y < MAX_X) {
x = 0;
while (x < MAX_X) {
if (image[x][y] == 1) {
*pixelHeight = y+1;
}
x = x + 1;
}
y = y + 1;
}
return pixelHeight;
}
//Width
//Loops through from bottom-left to the top, and then across
//through the X axis, find the last occurance of X, adds 1
//to deal with array 0 count, and
//returns this value as the pixel width
int * findXPixel(int image[MAX_X][MAX_Y]) {
int x, y, *pixelWidth;
x = 0;
while (x < MAX_X) {
y = 0;
while (y < MAX_Y) {
if (image[x][y] == 1) {
*pixelWidth = x+1;
}
y = y + 1;
}
x = x + 1;
}
return pixelWidth;
}
//Tallness function
double findTallness(int *pixelWidth, int *pixelHeight) {
double tallness;
tallness = *pixelWidth / *pixelHeight;
printf("tall=%.6lf\n", tallness);
return tallness;
}
谁能看到问题出在哪里?
请检查您的函数声明。 它们与函数定义不兼容。 我已经修改了代码,使其可以如下编译
#include <stdio.h>
#include <math.h>
#include <stdlib.h>
#define MAX_X 20
#define MAX_Y 20
int countBlackPixels(int image[MAX_X][MAX_Y]);
int* findYPixel(int image[MAX_X][MAX_Y]);
int* findXPixel(int image[MAX_X][MAX_Y]);
double findTallness(int *,int *);
//function prototypes here
void processImage(int image[MAX_X][MAX_Y]) {
int nBlackPixels;
nBlackPixels = countBlackPixels(image);
printf("Pixel-count: %d\n", nBlackPixels);
int *pixelHeight;
pixelHeight = findYPixel(image);
printf("Height: %d\n", *pixelHeight);
int *pixelWidth;
pixelWidth = findXPixel(image);
printf("Width: %d\n", *pixelWidth);
double tallness;
tallness = findTallness(pixelWidth, pixelHeight);
printf("Tallness: %.6lf\n", tallness);
}
//Count Black Pixels
int countBlackPixels(int image[MAX_X][MAX_Y]) {
int x, y, blackPixelCount;
blackPixelCount = 0;
x = 0;
while (x < MAX_X) {
y = 0;
while (y < MAX_X) {
if (image[x][y] == 1) {
blackPixelCount = blackPixelCount + 1;
//printf("black pixel at x=%d y=%d\n", x, y); // example debug printf
}
y = y + 1;
}
x = x + 1;
}
return blackPixelCount;
}
//Height
//Loops through from bottom-left to the right, and then up
//through the Y axis, find the last occurance of Y,
//adds one to overcome array's zero count, and
//returns this value as the pixel height
int * findYPixel(int image[MAX_X][MAX_Y]) {
int x, y, *pixelHeight;
pixelHeight = malloc(sizeof(int));
y = 0;
*pixelHeight = -1;
while (y < MAX_X) {
x = 0;
while (x < MAX_X) {
if (image[x][y] == 1) {
*pixelHeight = y+1;
}
x = x + 1;
}
y = y + 1;
}
return pixelHeight;
}
//Width
//Loops through from bottom-left to the top, and then across
//through the X axis, find the last occurance of X, adds 1
//to deal with array 0 count, and
//returns this value as the pixel width
int * findXPixel(int image[MAX_X][MAX_Y]) {
int x, y, *pixelWidth;
pixelWidth = malloc(sizeof(int));
x = 0;
while (x < MAX_X) {
y = 0;
while (y < MAX_Y) {
if (image[x][y] == 1) {
*pixelWidth = x+1;
}
y = y + 1;
}
x = x + 1;
}
return pixelWidth;
}
//Tallness function
double findTallness(int *pixelWidth, int *pixelHeight) {
double tallness;
tallness = (1.0 * *pixelWidth) / *pixelHeight;
printf("tall=%.6lf\n", tallness);
return tallness;
}
您没有分配内存在findXPixel
和findYPixel
存储整数。 要分配内存,请按如下所示初始化变量:
pixelHeight = malloc(sizeof(int));
同样, pixelWidth = malloc(sizeof(int));
另外,在processImage
,您不需要取消对指针*pixelHeight
和*pixelWidth
引用。 用作
pixelHeight = findYPixel(image);
pixelWidth = findXPixel(image);
同样,替换tallness = *pixelWidth / *pixelHeight;
与
tallness = (*pixelWidth * 1.0)/ *pixelHeight;
执行此类计算时,您需要将int
为double
。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.