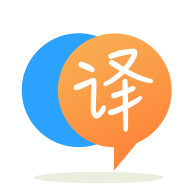
[英]How can I get the current location as a latitude and longitude in Android?
[英]Android - Why is my Current Location app always return 0 Latitude and 0 Longitude?
每当我调用getCurrentLocation()函数时,返回值始终为纬度:0.0和经度:0.0
我使用了实际的电话和仿真器,但两者仍然给出0和0。
这些在我的AndroidManifest.xml中
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.thesis.adrianangub.myapplication" >
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.INTERNET" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme" >
<!--
The API key for Google Maps-based APIs is defined as a string resource.
(See the file "res/values/google_maps_api.xml").
Note that the API key is linked to the encryption key used to sign the APK.
You need a different API key for each encryption key, including the release key that is used to
sign the APK for publishing.
You can define the keys for the debug and release targets in src/debug/ and src/release/.
-->
<meta-data
android:name="com.google.android.geo.API_KEY"
android:value="@string/google_maps_key" />
<activity
android:name=".MapsActivity"
android:label="@string/title_activity_maps" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
这些在我的MapsActivity.java中
package com.thesis.adrianangub.myapplication;
public class MapsActivity extends FragmentActivity implements OnMapReadyCallback, GoogleApiClient.ConnectionCallbacks, GoogleApiClient.OnConnectionFailedListener, GoogleMap.OnMarkerDragListener, GoogleMap.OnMapLongClickListener, View.OnClickListener
{
//Our Map
private GoogleMap mMap;
//To store longitude and latitude from map
private double longitude;
private double latitude;
//Buttons
private Button buttonSave;
private Button buttonCurrent;
private Button buttonView;
//Google ApiClient
private GoogleApiClient googleApiClient;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_maps);
// Obtain the SupportMapFragment and get notified when the map is ready to be used.
SupportMapFragment mapFragment = (SupportMapFragment) getSupportFragmentManager()
.findFragmentById(R.id.map);
mapFragment.getMapAsync(this);
//Initializing googleapi client
googleApiClient = new GoogleApiClient.Builder(this)
.addConnectionCallbacks(this)
.addOnConnectionFailedListener(this)
.addApi(LocationServices.API)
.build();
//Initializing views and adding onclick listeners
buttonSave = (Button) findViewById(R.id.buttonSave);
buttonCurrent = (Button) findViewById(R.id.buttonCurrent);
buttonView = (Button) findViewById(R.id.buttonView);
buttonSave.setOnClickListener(this);
buttonCurrent.setOnClickListener(this);
buttonView.setOnClickListener(this);
}
@Override
protected void onStart() {
googleApiClient.connect();
super.onStart();
}
@Override
protected void onStop() {
googleApiClient.disconnect();
super.onStop();
}
//Getting current location
private void getCurrentLocation() {
mMap.clear();
//Creating a location object
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// ActivityCompat#requestPermissions
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String[] permissions,
// int[] grantResults)
// to handle the case where the user grants the permission. See the documentation
// for ActivityCompat#requestPermissions for more details.
return;
}
Location location = LocationServices.FusedLocationApi.getLastLocation(googleApiClient);
if (location != null) {
//Getting longitude and latitude
longitude = location.getLongitude();
latitude = location.getLatitude();
//moving the map to location
moveMap();
}
}
//Function to move the map
private void moveMap() {
//String to display current latitude and longitude
String msg = latitude + ", "+longitude;
//Creating a LatLng Object to store Coordinates
LatLng latLng = new LatLng(latitude, longitude);
//Adding marker to map
mMap.addMarker(new MarkerOptions()
.position(latLng) //setting position
.draggable(true) //Making the marker draggable
.title("Current Location")); //Adding a title
//Moving the camera
mMap.moveCamera(CameraUpdateFactory.newLatLng(latLng));
//Animating the camera
mMap.animateCamera(CameraUpdateFactory.zoomTo(15));
//Displaying current coordinates in toast
Toast.makeText(this, msg, Toast.LENGTH_LONG).show();
}
@Override
public void onMapReady(GoogleMap googleMap) {
mMap = googleMap;
LatLng latLng = new LatLng(-34, 151);
mMap.addMarker(new MarkerOptions().position(latLng).draggable(true));
mMap.moveCamera(CameraUpdateFactory.newLatLng(latLng));
mMap.setOnMarkerDragListener(this);
mMap.setOnMapLongClickListener(this);
}
@Override
public void onConnected(Bundle bundle) {
getCurrentLocation();
}
@Override
public void onConnectionSuspended(int i) {
}
@Override
public void onConnectionFailed(ConnectionResult connectionResult) {
}
@Override
public void onMapLongClick(LatLng latLng) {
//Clearing all the markers
mMap.clear();
//Adding a new marker to the current pressed position
mMap.addMarker(new MarkerOptions()
.position(latLng)
.draggable(true));
}
@Override
public void onMarkerDragStart(Marker marker) {
}
@Override
public void onMarkerDrag(Marker marker) {
}
@Override
public void onMarkerDragEnd(Marker marker) {
//Getting the coordinates
latitude = marker.getPosition().latitude;
longitude = marker.getPosition().longitude;
//Moving the map
moveMap();
}
@Override
public void onClick(View v) {
if(v == buttonCurrent){
getCurrentLocation();
moveMap();
}
}
}
这是我的activity_maps.xml
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MapsActivity">
<fragment xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:map="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/map"
android:name="com.google.android.gms.maps.SupportMapFragment"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.thesis.adrianangub.myapplication.MapsActivity" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="bottom"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#cc3b60a7"
android:orientation="horizontal">
<Button
android:text="Curr"
android:id="@+id/buttonCurrent"
android:onClick="getCurrentLocation"
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_margin="15dp" />
<Button
android:text="Save"
android:id="@+id/buttonSave"
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_margin="15dp" />
<Button
android:text="View"
android:id="@+id/buttonView"
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_margin="15dp"/>
</LinearLayout>
</LinearLayout>
您必须请求棉花糖设备的许可。 参考此文档
在进行第一个活动时,在下面的代码中添加以下代码:
将其放在onCreate()方法顶部的两个变量下面:
您可以为棉花糖添加许多权限。 下面,我在字符串数组中添加了五个权限。 借助于此,我在oncreate方法之外授予了权限。
我们在字符串数组中添加的权限是什么,必须在清单中添加。
String[] perms = {"android.permission.CAMERA", "android.permission.WRITE_EXTERNAL_STORAGE", "android.permission.READ_EXTERNAL_STORAGE", "android.permission.ACCESS_FINE_LOCATION", "android.permission.ACCESS_COARSE_LOCATION"}; int permsRequestCode = 200;
将以下方法放在onCreate()方法中:
if(canMakeSmores()){
requestPermissions(perms, permsRequestCode);
}
将以下两个方法放在onCreate()方法之外:
private boolean canMakeSmores(){
return(Build.VERSION.SDK_INT>Build.VERSION_CODES.LOLLIPOP_MR1);
}
public void onRequestPermissionsResult(int permsRequestCode, String[] permissions, int[] grantResults){
switch(permsRequestCode){
case 200:
if(grantResults.length > 0){
boolean cameraAccepted = grantResults[0]==PackageManager.PERMISSION_GRANTED;
if(cameraAccepted){
}else {
requestPermissions(perms, permsRequestCode);
}
boolean writeAccepted = grantResults[1]==PackageManager.PERMISSION_GRANTED;
if(writeAccepted){
}else {
requestPermissions(perms, permsRequestCode);
}
boolean readAccepted = grantResults[2]==PackageManager.PERMISSION_GRANTED;
if(readAccepted){
}else {
requestPermissions(perms, permsRequestCode);
}
boolean fineLocationAccepted = grantResults[3]==PackageManager.PERMISSION_GRANTED;
if(fineLocationAccepted){
}else {
requestPermissions(perms, permsRequestCode);
}
boolean coarseLocationAccepted = grantResults[4]==PackageManager.PERMISSION_GRANTED;
if(coarseLocationAccepted){
}else {
requestPermissions(perms, permsRequestCode);
}
}
break;
}
}
表现:
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.ACCESS_GPS" />
<uses-permission android:name="android.permission.ACCESS_LOCATION" />
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.