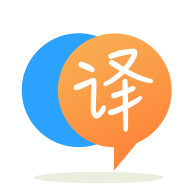
[英]How can I add key values into a dictionary from reading in each line of a .txt file and update values?
[英]How to create Python dictionary with multiple 'lists' for each key by reading from .txt file?
我有一个大文本文件,看起来像:
1 27 21 22
1 151 24 26
1 48 24 31
2 14 6 8
2 98 13 16
.
.
.
我想用它创建一个字典。 每个列表的第一个数字应该是字典中的键,应该采用以下格式:
{1: [(27,21,22),(151,24,26),(48,24,31)],
2: [(14,6,8),(98,13,16)]}
我有以下代码(总点数是文本文件第一列中的最大数字(即字典中的最大键)):
from collections import defaultdict
info = defaultdict(list)
filetxt = 'file.txt'
i = 1
with open(filetxt, 'r') as file:
for i in range(1, num_cities + 1):
info[i] = 0
for line in file:
splitLine = line.split()
if info[int(splitLine[0])] == 0:
info[int(splitLine[0])] = ([",".join(splitLine[1:])])
else:
info[int(splitLine[0])].append((",".join(splitLine[1:])))
哪个输出
{1: ['27,21,22','151,24,26','48,24,31'],
2: ['14,6,8','98,13,16']}
我想要这个字典的原因是因为我想在给定键的字典的每个“内部列表”中运行for循环:
for first, second, third, in dictionary:
....
我不能用我当前的代码执行此操作,因为字典的格式略有不同(它在上面的for循环中需要3个值,但是接收的值超过3个),但它可以使用第一个字典格式。
任何人都可以建议解决这个问题吗?
result = {}
with open(filetxt, 'r') as f:
for line in f:
# split the read line based on whitespace
idx, c1, c2, c3 = line.split()
# setdefault will set default value, if the key doesn't exist and
# return the value corresponding to the key. In this case, it returns a list and
# you append all the three values as a tuple to it
result.setdefault(idx, []).append((int(c1), int(c2), int(c3)))
编辑:由于您希望键也是一个整数,您可以将int
函数map
到拆分值,如下所示
idx, c1, c2, c3 = map(int, line.split())
result.setdefault(idx, []).append((c1, c2, c3))
您正在将值转换回逗号分隔的字符串,这些字符串不能用于数据中for first, second, third in data
- 所以只需将它们保留为列表splitLine[1:]
(或转换为tuple
)。
您不需要使用defaultdict
初始化for
循环。 您也不需要使用defaultdict
进行条件检查。
你的代码没有多余的代码:
with open(filetxt, 'r') as file:
for line in file:
splitLine = line.split()
info[int(splitLine[0])].append(splitLine[1:])
一个细微的差别是如果你想在int
上运行我会在前面进行转换:
with open(filetxt, 'r') as file:
for line in file:
splitLine = list(map(int, line.split())) # list wrapper for Py3
info[splitLine[0]].append(splitLine[1:])
实际上在Py3中,我会这样做:
idx, *cs = map(int, line.split())
info[idx].append(cs)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.