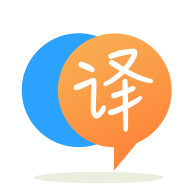
[英]How can I calculate the crop size (rect) of the UIImage with different resolution
[英]How to Crop a UiImage to specific Size?
嗨,我正在使用UIimage,我需要将图像裁剪为特定的尺寸,假设我的特定尺寸的宽度等于设备的宽度,高度固定为200。我尝试了以下示例
-(UIImage *)resizeImage:(UIImage *)image
{
float h=200;
float w=400;
float actualHeight = image.size.height;
float actualWidth = image.size.width;
float maxHeight = h;
float maxWidth = w;
float imgRatio = actualWidth/actualHeight;
float maxRatio = maxWidth/maxHeight;
float compressionQuality = 0.8;//50 percent compression
if (actualHeight > maxHeight || actualWidth > maxWidth)
{
if(imgRatio < maxRatio)
{
//adjust width according to maxHeight
imgRatio = maxHeight / actualHeight;
actualWidth = imgRatio * actualWidth;
actualHeight = maxHeight;
}
else if(imgRatio > maxRatio)
{
//adjust height according to maxWidth
imgRatio = maxWidth / actualWidth;
actualHeight = imgRatio * actualHeight;
actualWidth = maxWidth;
}
else
{
actualHeight = maxHeight;
actualWidth = maxWidth;
}
}
CGRect rect = CGRectMake(0.0, 0.0, actualWidth, actualHeight);
UIGraphicsBeginImageContext(rect.size);
//UIGraphicsBeginImageContextWithOptions(rect.size,NO,0.0);
[image drawInRect:rect];
UIImage *img = UIGraphicsGetImageFromCurrentImageContext();
NSData *imageData = UIImageJPEGRepresentation(img, compressionQuality);
UIGraphicsEndImageContext();
return [UIImage imageWithData:imageData];
}
以下是我的实际图像
裁剪后我变得像下面
我所有的示例都在拉伸图像,但是我不希望拉伸就需要缩放图像。我想念的地方在哪里?请帮助我。
您使用的代码将调整图像的大小,我们将其用于图像压缩或图像调整大小。 如果要裁剪图像,有两种方法。 1)选择图像后,允许用户进行编辑。 您可以通过将allowEditing属性添加到选择器来实现此目的,例如,
pickerView.allowEditing = true
这将为您提供矩形以裁剪图像,您可以从选择器视图的didFinish方法中检索编辑的图像
2)以编程方式
这是我正在使用的功能
func croppImage(originalImage:UIImage, toRect rect:CGRect) -> UIImage{
var imageRef:CGImageRef = CGImageCreateWithImageInRect(originalImage.CGImage, rect)
var croppedimage:UIImage = UIImage(CGImage:imageRef)
return croppedimage
}
上面的代码将返回您的裁剪图像。
这些会将您的图像裁剪为所需的尺寸。
- (CGImageRef)newTransformedImage:(CGAffineTransform)transform
sourceImage:(CGImageRef)sourceImage
sourceSize:(CGSize)sourceSize
sourceOrientation:(UIImageOrientation)sourceOrientation
outputWidth:(CGFloat)outputWidth
cropSize:(CGSize)cropSize
imageViewSize:(CGSize)imageViewSize
{
CGImageRef source = [self newScaledImage:sourceImage
withOrientation:sourceOrientation
toSize:sourceSize
withQuality:kCGInterpolationNone];
CGFloat aspect = cropSize.height/cropSize.width;
CGSize outputSize = CGSizeMake(outputWidth, outputWidth*aspect);
CGContextRef context = CGBitmapContextCreate(NULL,
outputSize.width,
outputSize.height,
CGImageGetBitsPerComponent(source),
0,
CGImageGetColorSpace(source),
CGImageGetBitmapInfo(source));
CGContextSetFillColorWithColor(context, [[UIColor clearColor] CGColor]);
CGContextFillRect(context, CGRectMake(0, 0, outputSize.width, outputSize.height));
CGAffineTransform uiCoords = CGAffineTransformMakeScale(outputSize.width / cropSize.width,
outputSize.height / cropSize.height);
uiCoords = CGAffineTransformTranslate(uiCoords, cropSize.width/2.0, cropSize.height / 2.0);
uiCoords = CGAffineTransformScale(uiCoords, 1.0, -1.0);
CGContextConcatCTM(context, uiCoords);
CGContextConcatCTM(context, transform);
CGContextScaleCTM(context, 1.0, -1.0);
CGContextDrawImage(context, CGRectMake(-imageViewSize.width/2.0,
-imageViewSize.height/2.0,
imageViewSize.width,
imageViewSize.height)
, source);
CGImageRef resultRef = CGBitmapContextCreateImage(context);
CGContextRelease(context);
CGImageRelease(source);
return resultRef;
}
- (CGImageRef)newScaledImage:(CGImageRef)source withOrientation:(UIImageOrientation)orientation toSize:(CGSize)size withQuality:(CGInterpolationQuality)quality
{
CGSize srcSize = size;
CGFloat rotation = 0.0;
switch(orientation)
{
case UIImageOrientationUp: {
rotation = 0;
} break;
case UIImageOrientationDown: {
rotation = M_PI;
} break;
case UIImageOrientationLeft:{
rotation = M_PI_2;
srcSize = CGSizeMake(size.height, size.width);
} break;
case UIImageOrientationRight: {
rotation = -M_PI_2;
srcSize = CGSizeMake(size.height, size.width);
} break;
default:
break;
}
CGContextRef context = CGBitmapContextCreate(NULL,
size.width,
size.height,
8, //CGImageGetBitsPerComponent(source),
0,
CGImageGetColorSpace(source),
(CGBitmapInfo)kCGImageAlphaNoneSkipFirst //CGImageGetBitmapInfo(source)
);
CGContextSetInterpolationQuality(context, quality);
CGContextTranslateCTM(context, size.width/2, size.height/2);
CGContextRotateCTM(context,rotation);
CGContextDrawImage(context, CGRectMake(-srcSize.width/2 ,
-srcSize.height/2,
srcSize.width,
srcSize.height),
source);
CGImageRef resultRef = CGBitmapContextCreateImage(context);
CGContextRelease(context);
return resultRef;
}
///您想要裁剪的位置。
CGImageRef imageRef = [self newTransformedImage:transform
sourceImage:self.image.CGImage
sourceSize:self.image.size
sourceOrientation:self.image.imageOrientation
outputWidth:self.image.size.width
cropSize:self.photoView.cropView.frame.size
imageViewSize:self.photoView.photoContentView.bounds.size];
UIImage *image = [UIImage imageWithCGImage:imageRef];
CGImageRelease(imageRef);
谢谢。 享受编码:)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.