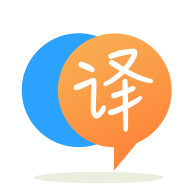
[英]Adding a delay without Thread.sleep and a while loop doing nothing
[英]Adding a delay/ Thread.sleep
所以我正在用Java做一个基本的答题游戏。 到目前为止,我已经进行了两次升级,并且必须要有一个老板形象。 我希望这样,一旦达到一定程度,老板的形象就会在5秒钟后消失,并且会出现一个JLabel,说“您打败了”老板的名字”。但是您听到了一些声音”。 我的问题是,一旦达到设定的分数,老板的形象就不会消失。 这是我的代码
// The "Clicker" class.
import java.util.concurrent.TimeUnit;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Insets;
import javax.swing.*;
import java.awt.event.*;
public class Clicker extends JFrame implements ActionListener
{
static int multiply = 1;//Multiplier
static boolean x2 = false;//Multiplier bought or not boolean
static boolean add10 = false;//+10 bought or not boolean
static int score = 950;//Startingn points 0
static JFrame j = new JFrame ("Clicker");
JLabel l = new JLabel ("Points: " + String.valueOf (score));//Points Label
static JLabel name = new JLabel ();//Name Label
JTextField jt = new JTextField ("Name");//Name Text Box
JButton click = new JButton ();//+1 Button
public static void main (String[] args)
{
Clicker run = new Clicker ();//Object of class
} // main method
public Clicker ()
{
JLabel boss1 = new JLabel ();//Boss Label
JButton multiplier = new JButton ("x2(5000)");//X2 upgrade button
JButton plus10 = new JButton ("+10(100 Points)");//+10 upgrade button
JButton enter = new JButton ("Enter");//Enter name
JPanel p = new JPanel ();//Main Pane
JButton reset = new JButton ("Reset");//Reset Button
multiplier.addActionListener (new ActionListener ()//X2 MULTIPLIER ACTION PERFORMED
{
public void actionPerformed (ActionEvent e){
if (score > 5000 || score == 5000)// If you have enough to buy upgrade
{
if (x2 == false)// If you did not previously but upgrade
{
score = score - 5000;//Subtract 5000 points
l.setText ("Points: " + score);//Redisplay Points
click.setVisible (false);
x2 = true;//Set true to show you bought upgrade
}
}
if (x2 == true)//If bought upgrade
{
multiply = 2;//Set multiplier by 2
multiplier.setVisible(false);//set boolean to true so they can't rebuy
}
else if (score < 5000)//If you dont have enough points
{
JOptionPane.showMessageDialog (null, "You do not have enough points.", null, JOptionPane.INFORMATION_MESSAGE);//Dont have enough points message
}
}
});
plus10.addActionListener (new ActionListener ()//PLUS10 BUTTON ACTION PERFORM
{
public void actionPerformed (ActionEvent e)
{
if (score > 100 || score == 100)//If you have more than 100 points
{
if (add10 == false)//For first time buy
{
score = score - 100;
l.setText ("Points: " + score);
click.setVisible (false);//Remove +1 button and replace with +10
Insets insets = p.getInsets ();
plus10.setBounds (75+ insets.left, 250 + insets.top, 150, 100);//Move +10 button to where the +1 button was
add10 = true;//Set true so it doesn't take 100 points off again
}
}
if (add10 == true)
{
score = score + 10 * (multiply);//Adding 10
l.setText ("Points: " + score);//Redisplay score
}
else if (score < 100)//If score is less than 100
{
JOptionPane.showMessageDialog (null, "You do not have enough points.", null, JOptionPane.INFORMATION_MESSAGE);//Dont have enough points for upgrade
}
}
}
);
enter.addActionListener (new ActionListener ()//ENTER NAME ACTION PERFORMED
{
public void actionPerformed (ActionEvent e)
{
String input = jt.getText ();
name.setText (input);
}
}
);
jt.addActionListener (new ActionListener ()//NAME TEXTBOX ACTION PERFORMED
{
public void actionPerformed (ActionEvent e)
{
String input = jt.getText ();
name.setText (input);
}
}
);
reset.addActionListener (new ActionListener ()//RESET BUTTON ACTION PERFORMED
{
public void actionPerformed (ActionEvent e)
{
score = 0;
l.setText ("Points: " + score);
}
}
);
/////////////////////////////////////////////////BOSS ATTACK
int boss1health;
boss1health = 1000;
if(score >= boss1health){
try{
boss1.setVisible(false);
Thread.sleep(5000);
}
catch(InterruptedException e){
Thread.currentThread().interrupt();
}
JLabel boss1defeat = new JLabel ("You defeating MewTwo. But you see another threat approuching.");
}
/////////////////////////////////////////////////BOSS ATTACK
ImageIcon boss1image = new ImageIcon(getClass().getResource("mewtwospirte.png") );
boss1.setIcon(boss1image);//SETTING BOSS1 IMAGE TO MEWTWO
ImageIcon cimage = new ImageIcon(getClass().getResource("plus1.png") );//PLUS ONE IMAGE
click.setIcon(cimage);//SETTING PLUS ONE IMAGE TO +1 CLICKER
//Change Color
name.setBackground (Color.WHITE);
jt.setBackground (Color.WHITE);
p.setBackground (Color.WHITE);
p.setBackground(Color.WHITE);
click.setBackground(Color.WHITE);
j.getContentPane ().add (p);//ADD PANE TO JFRAME
p.setLayout (null);//Set Pane to Absolute Layout
Insets insets = p.getInsets ();//Basically Coordinates/Pixels of JFrame
Dimension size = jt.getPreferredSize ();//Gets Size based off font, etc
jt.setBounds (0 + insets.left, 5 + insets.top, 75, size.height);//Placing the function
size = enter.getPreferredSize ();//Gets Size based off font, etc
enter.setBounds (75 + insets.left, 5 + insets.top, size.width, size.height);//Placing the function
size = click.getPreferredSize ();//Gets Size based off font, etc
click.setBounds (75+ insets.left, 250 + insets.top, 150, 100);//Placing the function
size = reset.getPreferredSize ();//Gets Size based off font, etc
reset.setBounds (0 + insets.left, 370 + insets.top, size.width, size.height);//Placing the function
size = name.getPreferredSize ();//Gets Size based off font, etc
name.setBounds (0 + insets.left, 20 + insets.top, 50, 50);//Placing the function
size = l.getPreferredSize ();//Gets Size based off font, etc
l.setBounds (175 + insets.left, 30 + insets.top, 100, size.height);//Placing the function
size = plus10.getPreferredSize ();//Gets Size based off font, etc
plus10.setBounds (165 + insets.left, 370 + insets.top, size.width, size.height);//Placing the function
size = multiplier.getPreferredSize();//Gets Size based off font, etc
multiplier.setBounds(170 + insets.left, 5 + insets.top, size.width, size.height);//Placing the function
boss1.setBounds(75 + insets.left, 75 + insets.top, 175, 175);//Placing the function
//ADDING ALL THE COMPONENETS/FUNCTIONS
p.add(boss1);
p.add(multiplier);
p.add (plus10);
p.add (jt);
p.add (name);
p.add (reset);
p.add (l);
p.add (click);
p.add (enter);
click.addActionListener (this);
j.setResizable (false);//Set the JFrame to not be resizable
j.setSize (300, 450);//Set JFrame Size
j.setVisible (true);//Set JFrame to be visible
}
public void actionPerformed (ActionEvent e)//Action performed when clicking the +1 button
{
score = score + 1 * (multiply);
l.setText ("Points: " + score);
}
}
我知道在您击败老板后,我还没有添加JLabel来显示消息。 我想解决的问题是老板形象首先变得不可见。 谢谢您的帮助
尝试这个:
if(score >= boss1health){
try{
new Handler().postDelayed(new Runnable() {
@Override
public void run() {
boss1.setVisible(false);
}
}, 5000);
}
catch(InterruptedException e){
Thread.currentThread().interrupt();
}
JLabel boss1defeat = new JLabel ("You defeating MewTwo. But you see another threat approuching.");
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.