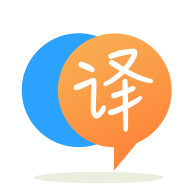
[英]I need help to store my image in internal directory and its path is insert in SQLite
[英]How do I change the directory path of my program whilst its running
Private Sub _2013results_Load(sender As Object, e As EventArgs) Handles MyBase.Load
dBprovider = "Provider=Microsoft.ACE.OLEDB.12.0;Data Source =" 'This is the type of connection I've chose to link to the database
'Change the following to your access database location
datafile = "C:\Year 13\SheetFormats.accdb"
connString = dBprovider & datafile
myConnection.ConnectionString = connString
End Sub
特别是在“ datafile”行上,该行具有数据库文件的路径,这是要发送给其他学生,他们可能与我的路径不同,因此有什么方法可以创建警报来允许用户修改代码以适合他们的路径
希望这可以帮助您到达想要去的地方。 一旦熟悉了此代码,就可以考虑将Private dbFilePath As String
交换为用户设置,以便每次用户使用应用程序时都可以Private dbFilePath As String
更改。
Imports System.IO
Public Class Form1
Private dbFilePath As String = "" 'this is where I will store the path to the DB file
Private Sub btn_Go_Click(sender As Object, e As EventArgs) Handles btn_Go.Click
If dbFilePath = "" Then
'if the DB path is empty, the user must provide one
GetDbFilePath()
btn_Go_Click(sender, e)
Else
'there is already a path
_2013results_Load("", EventArgs.Empty)
'on your TO DO list, implement code so that if the user already specified a path but he/she wants to update it.
End If
End Sub
''' <summary>
''' This sub will 'prompt' the user for a path
''' </summary>
Private Sub GetDbFilePath()
Dim message, title, defaultValue As String
Dim myvalue As Object
message = "Please enter the path of your db file"
title = "DB path grabber"
defaultValue = ""
myvalue = InputBox(message, title, defaultValue)
If myvalue IsNot "" AndAlso File.Exists(myvalue) Then
'if the user entered something, and that something is a valid path
dbFilePath = myvalue
End If
End Sub
Private Sub _2013results_Load(sender As Object, e As EventArgs) Handles MyBase.Load
If dbFilePath = "" Then
Return
End If
Dim dBprovider As String
dBprovider = "Provider=Microsoft.ACE.OLEDB.12.0;Data Source =" 'This is the type of connection I've chose to link to the database
'Change the following to your access database location
Dim connString = dBprovider & dbFilePath
Dim myconnection As OleDb.OleDbConnection = New OleDb.OleDbConnection()
myconnection.ConnectionString = connString
End Sub
End Class
嗯...这里是使用用户设置。 这是一个非常基本的实现,但是它几乎不需要完成任何工作。 这种方式假设您有一个名为“ settings_DbFilePath”的用户设置。 要创建这样的设置...
在解决方案资源管理器->您的解决方案->您的项目->设置中
名称:settings_DbFilePath
类型:字符串
范围:用户
值:
Private Sub GetDbFilePath_UserSettingsWay()
Dim message, title, defaultValue As String
Dim myvalue As Object
message = "Please enter the path of your db file"
title = "DB path grabber"
defaultValue = ""
myvalue = InputBox(message, title, defaultValue)
If myvalue IsNot "" AndAlso File.Exists(myvalue) Then
'if the user entered something, and that something is a valid path
My.MySettings.Default.settings_DbFilePath = myvalue
My.MySettings.Default.Save()
End If
End Sub
Private Sub _2013results_Load_UserSettingsWay(sender As Object, e As EventArgs) Handles MyBase.Load
If My.MySettings.Default.settings_DbFilePath = "" Then
Return
End If
Dim dBprovider As String
dBprovider = "Provider=Microsoft.ACE.OLEDB.12.0;Data Source =" 'This is the type of connection I've chose to link to the database
'Change the following to your access database location
Dim connString = dBprovider & My.MySettings.Default.settings_DbFilePath
Dim myconnection As OleDb.OleDbConnection = New OleDb.OleDbConnection()
myconnection.ConnectionString = connString
End Sub
这是第三个替代方法,因此您可以更好地了解其他两种方法在做什么,因此可以查明应在代码中的什么地方实现这些方法。 在此代码段中,我基本上只是重构并向现有的_2013results_Load
方法中添加了一些内容。 但是,这第三种选择确实希望您已经创建了一个名为settings_DbFilePath
的string
类型的用户设置,如选项#2中所述。
Private Sub _2013results_Load(sender As Object, e As EventArgs) Handles MyBase.Load
Dim dBprovider As String
dBprovider = "Provider=Microsoft.ACE.OLEDB.12.0;Data Source =" 'This is the type of connection I've chose to link to the database
'Now lets ask the user for the DB path
Dim message, title, defaultValue As String 'initialize some strings
Dim userAnswer As Object 'initialize an object
message = "Please enter the path of your db file"
title = "DB path grabber"
defaultValue = ""
userAnswer = InputBox(message, title, defaultValue) 'This line triggers the prompt
If userAnswer IsNot "" AndAlso File.Exists(userAnswer) Then
'if the user entered something, and that something is a valid path
My.MySettings.Default.settings_DbFilePath = userAnswer 'park that value in the user setting
My.MySettings.Default.Save() 'save the settings
Else
Return 'the user did not provide a valid path so get out of here without even attempting to connect!
End If
Dim userAnswerPulledFromSettings As String
userAnswerPulledFromSettings = My.MySettings.Default.settings_DbFilePath
Dim connString = dBprovider & userAnswerPulledFromSettings 'put your connection string together
Dim myconnection As OleDb.OleDbConnection = New OleDb.OleDbConnection() 'initialize your connection object
myconnection.ConnectionString = connString 'use your connection string
End Sub
简而言之
datafile = "C:\Year 13\SheetFormats.accdb"
成为
'Now lets ask the user for the DB path
Dim message, title, defaultValue As String 'initialize some strings
Dim userAnswer As Object 'initialize an object
message = "Please enter the path of your db file"
title = "DB path grabber"
defaultValue = ""
userAnswer = InputBox(message, title, defaultValue) 'This line triggers the prompt
If userAnswer IsNot "" AndAlso File.Exists(userAnswer) Then
'if the user entered something, and that something is a valid path
My.MySettings.Default.settings_DbFilePath = userAnswer 'park that value in the user setting
My.MySettings.Default.Save() 'save the settings
Else
Return 'the user did not provide a valid path so get out of here without even attempting to connect!
End If
Dim userAnswerPulledFromSettings As String
userAnswerPulledFromSettings = My.MySettings.Default.settings_DbFilePath
为什么不只是添加配置文件。 您可以在安装应用程序时生成它,然后在需要时让用户从那里更改路径。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.