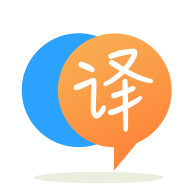
[英]In this program(Java) I'm trying to make a dice roller. How do I make it so it rolls a bunch of times and adds the rolls?
[英]How do I simulate 3000 dice rolls and count the number of times doubles are rolled? JAVA
我是编程领域的新手,所以如果您能帮助我变得更好,我将深表感谢。 我对该程序的目标是模拟3000个骰子掷骰,并使用while循环为每个不同的可能双掷对计算掷双骰的次数。 结果应在对话框中打印出来。
Random random;
random = new Random();
diceRolls = 1;
snakeEyes = 1;
doubleTwos = 1;
doubleThrees = 1;
doubleFours = 1;
doubleFives = 1;
doubleSixes = 1;
while (diceRolls <= finalDiceRoll) {
int diceRolls = 1;
int die1 = random.nextInt(6) + 1;
int die2 = random.nextInt(6) + 1;
if (die1 == 1 && die2 == 1){
//snakeEyes = snakeEyes + 1;
snakeEyes++;
}
else if (die1 == 2 && die2 == 2 ) {
doubleTwos++;
}
else if (die1 == 3 && die2 == 3) {
doubleThrees++;
}
else if (die1 == 4 && die2 == 4) {
doubleFours++;
}
else if (die1 == 5 && die2 == 5) {
doubleFives++;
}
else if (die1 == 6 && die2 == 6) {
doubleSixes++;
}
JOptionPane.showMessageDialog (null, "You rolled snake eyes " + snakeEyes + " times\nYou rolled double twos " + doubleTwos + " times\nYou"
+ " rolled double threes " + doubleThrees + " times\nYou rolled double fours " + doubleFours + " times\nYou"
+ " rolled double fives " + doubleFives + " times\nYou rolled double sixes " + doubleSixes + " times");
}
我在这里遇到的问题是,我从程序中获得的结果似乎并不“合理”。 例如,在3000个骰子卷中,每双我得到1对。 我究竟做错了什么?
将showMessageDialog
移动到while循环之外,并增加diceRolls
变量。 用0初始化所有其他整数变量。
更好(更简洁)的方法是使用二维数组或地图。
Random random = new Random();
int snakeEyes = 0;
int doubleTwos = 0;
int doubleThrees = 0;
int doubleFours = 0;
int doubleFives = 0;
int doubleSixes = 0;
int diceRolls = 1;
while (diceRolls <= 3000) {
int die1 = random.nextInt(6) + 1;
int die2 = random.nextInt(6) + 1;
if (die1 == 1 && die2 == 1) {
snakeEyes++;
} else if (die1 == 2 && die2 == 2) {
doubleTwos++;
} else if (die1 == 3 && die2 == 3) {
doubleThrees++;
} else if (die1 == 4 && die2 == 4) {
doubleFours++;
} else if (die1 == 5 && die2 == 5) {
doubleFives++;
} else if (die1 == 6 && die2 == 6) {
doubleSixes++;
}
diceRolls++;
}
JOptionPane.showMessageDialog(null, "You rolled snake eyes " + snakeEyes + " times\nYou rolled double twos " + doubleTwos + " times\nYou"
+ " rolled double threes " + doubleThrees + " times\nYou rolled double fours " + doubleFours + " times\nYou"
+ " rolled double fives " + doubleFives + " times\nYou rolled double sixes " + doubleSixes + " times");
上述简称:
Random random = new Random();
int[] doubled = new int[6];
for (int diceRolls = 0; diceRolls < 3000; diceRolls++) {
int die1 = random.nextInt(6);
int die2 = random.nextInt(6);
if (die1 == die2)
doubled[die1]++;
}
JOptionPane.showMessageDialog(null, "You rolled snake eyes " + doubled[0] + " times\nYou rolled double twos " + doubled[1] + " times\nYou"
+ " rolled double threes " + doubled[2] + " times\nYou rolled double fours " + doubled[3] + " times\nYou"
+ " rolled double fives " + doubled[4] + " times\nYou rolled double sixes " + doubled[5] + " times");
您无需在代码中进行一些更改,
1.用0
初始化所有变量。
2.将while-loop
条件更改为
while (diceRolls <= finalDiceRoll) // hoping that finalDiceRoll = 3000
3移除int diceRolls = 1;
从while-loop
并添加diceRolls++;
在while-loop
结束while-loop
。
4.将JOptionPane
放在while-loop
之外。 如果不是,则必须关闭JOptionPane
dailog 3000次。
将这些更改应用于代码后,您将了解做错了什么,并且代码可以正常运行。
计数逻辑应如下所示:
doubleTwos = 0;
doubleThrees = 0;
doubleFours = 0;
doubleFives = 0;
doubleSixes = 0;
finalDiceRoll = 3000;
int diceRolls = 0;
while (diceRolls < finalDiceRoll) {
++diceRolls;
循环后带有任何消息。
使用数组,您可以保存键入:
int[] doubleCounts = new int[6]; // By 0 based dice values
for (int i = 0; i < finalDiceRoll; ++i) {
int die1 = random.nextInt(6); // With dice values 0-5
int die2 = random.nextInt(6);
if (die1 == die2) {
++doubleCounts[die1];
}
}
int snakeEyes = doubleCounts[0];
int doubleTwos = doubleCounts[1];
...
简化。 消除重复。 当可以使用数组时,请勿使用多个变量。
int[] doubles = new int[7]; // make the index the number rolled (ignore index 0)
int diceRolls = 0;
while (diceRolls++ < 3000) {
int die1 = random.nextInt(6) + 1;
int die2 = random.nextInt(6) + 1;
if (die1 == die2)
doubles[die1]++;
}
String output = "";
for (int i = 1; i <= 6; i++)
output += "You rolled double " + i + "'s " + doubles[i] + " times\n";
JOptionPane.showMessageDialog (null, output);
这就是您所需要的。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.