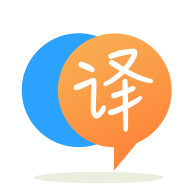
[英]reading from file using fscanf and saving into a array of struct c language
[英]Saving a string from a text file to a struct using fscanf (C)
示例文本文件:
234765 PETER
867574 SMITH
我正在尝试从文本文件中获取 id 和字符串并将其保存到结构中。 id 保存得很好,但字符串不是。
typedef struct student
{
int id[DATA_SIZE];
char *student[DATA_SIZE];
}studentinfo;
studentinfo list;
struct student *create_space(int size)
{
struct student *tmp = (struct student*)malloc(size*sizeof(struct student));
return(tmp);
}
struct student * readData(struct student*pointer,studentinfo v)
{
int count =0;
int tmpid;
char str[256];
FILE* in_file;
in_file = fopen("studentlist.txt","r");
while(fscanf(in_file,"%d",&tmpid)!= EOF && count<DATA_SIZE)
{
fscanf(in_file,"%s",v.student[count]);
//printf("%s\n",str );
v.id[count]=tmpid;
count++;
}
pointer =&v;
return pointer;
}
int main()
{
struct student *data;
struct student *sdata;
data = create_space(1);
sdata = readData(data,list);
//printf("%s\n",sdata->student[2] );
}
他们有几个问题:
fscanf()
读取格式化的输入,并返回读取的项目数。
这一行:
while(fscanf(in_file,"%d",&tmpid)!= EOF && count<DATA_SIZE)
可能是这样的:
while (count < DATA_SIZE && fscanf(in_file, "%d %255s", &list.id[count], str) == 2) {
这验证了在每行上成功读取了2
值。
您不是在检查in_file
返回NULL
。 这样做是安全的。 这对于malloc()
也是一样的。
您需要为char *students[DATA_SIZE]
正确创建空间,因为这是一个char *
指针数组。 一旦您通过malloc()
或strdup()
为此分配了空间,您就可以将内容复制到students
。
这是做这样的事情的一个例子:
while (count < DATA_SIZE && fscanf(in_file, "%d %255s", &list.id[count], str) == 2) { /* allocate space for one student */ list.student[count] = malloc(strlen(str)+1); if (!list.student[count]) { printf("Cannot allocate string\\n"); exit(EXIT_FAILURE); } /* copy it into array */ strcpy(list.student[count], str); count++; }
下面是一个示例,您可以使用它来帮助实现您想要的结果:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define DATA_SIZE 256
typedef struct {
int id[DATA_SIZE];
char *student[DATA_SIZE];
} studentinfo_t;
int main(void) {
FILE *in_file;
studentinfo_t list;
char str[DATA_SIZE];
size_t count = 0;
in_file = fopen("studentlist.txt", "r");
if (!in_file) {
fprintf(stderr, "%s\n", "Error reading file");
exit(EXIT_FAILURE);
}
while (count < DATA_SIZE && fscanf(in_file, "%d %255s", &list.id[count], str) == 2) {
list.student[count] = malloc(strlen(str)+1);
if (!list.student[count]) {
printf("Cannot allocate string\n");
exit(EXIT_FAILURE);
}
strcpy(list.student[count], str);
count++;
}
for (size_t i = 0; i < count; i++) {
printf("%d %s\n", list.id[i], list.student[i]);
}
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.