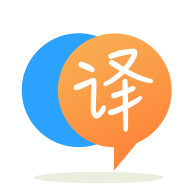
[英]How to read text from a .txt file and then store it in a record (data structure)?
[英]Read from a text file to fill a structure type
[代码正在运行,谢谢您的帮助。]
我可以使程序打印出第一组struct auto_t
。 当我尝试打印其他集时,什么也没发生,或者出现错误。
这就是我要做的。
定义结构类型auto_t代表汽车。 包括制造商和型号(字符串),里程表读数,制造和购买日期(使用另一个用户定义的类型,称为date_t)和储气罐(使用用户定义的类型tank_t,其组件具有储罐容量和电流的组件)的组件。燃油水平,以加仑为单位)。 编写I / O函数scan_date
, scan_tank
, scan_auto
, print_date
, print_tank
和print_auto
,还编写一个驱动程序函数,该函数重复填充并显示一个自动结构变量,直到在输入文件中遇到EOF
为止。
这是一个尝试的小数据集:
Mercury Sable 99842 1 18 2001 5 30 1991 16 12.5
Mazda Navajo 123961 2 20 1993 6 15 1993 19.3 16.7
这是有效的代码:
[如果您发现我错过的代码有什么问题,我不介意反馈]
#include <stdio.h>
#define SIZE 20
// the structures
typedef struct //struct for date
{
int month,day,year;
} date_t;
typedef struct //struct for the tank info
{
double capacity;
double curent_Fuel;
} tank_t;
typedef struct //the struct for the automobie
{
char make[SIZE];
char model[SIZE];
int odometer;
date_t manufact;
date_t purchase;
tank_t tank;
} auto_t;
//the function
void print_date(date_t da);
void print_tank(tank_t ta);
void print_auto(auto_t au);
int scan_date(date_t *date);
int scan_tank(tank_t *tank);
int scan_automobile(auto_t *automo);
//Start of program
int main (void)
{
auto_t car;
int stat = 1;
FILE *Car_data; //file used
Car_data = fopen("car.txt", "r");// has the date for the cars like make, model ect.
if (Car_data==NULL){
printf("ERROR: File failed to open");
getch();
exit(1);
fclose(Car_data);}
else
while(stat>0)
{
stat=fscanf(Car_data, "%s %s %d %d %d %d %d %d %d %lf %lf", &car.make,
&car.model,
&car.odometer,
&car.manufact.month,
&car.manufact.day,
&car.manufact.year,
&car.purchase.month,
&car.purchase.day,
&car.purchase.year,
&car.tank.capacity,
&car.tank.curent_Fuel);
if (stat==11)
{
print_auto(car);
printf("Maufactured date:");
print_date(car.manufact);
printf("\nPurchased date:");
print_date(car.purchase);
printf("\nTank capacity and current fuel");
print_tank(car.tank);
}
}
getch(); // Just used to keep the data on the sreen for testing pupose
return(0);
}
int scan_date(date_t *date)
{
int res;
res=scanf("%d %d %d", &(*date).month, &(*date).day, &(*date).year);
if(res==3)
res=1;
else if(res !=EOF)
res=0;
return(res);
}
int scan_tank(tank_t *tank)
{
int res;
res=scanf("%lf %lf", &(*tank).capacity, &(*tank).curent_Fuel);
if(res==2)
res=1;
else if(res !=EOF)
res=0;
return(res);
}
int scan_automobile(auto_t *automo)
{
int res;
res=scanf("%s %s %d %d %d %d %d %d %d %lf %lf", &(*automo).make,
&(*automo).model,
&(*automo).odometer,
&(*automo).manufact.month,
&(*automo).manufact.day,
&(*automo).manufact.year,
&(*automo).purchase.month,
&(*automo).purchase.day,
&(*automo).purchase.year,
&(*automo).tank.capacity,
&(*automo).tank.curent_Fuel);
if(res==11)
res=1;
else if(res !=EOF)
res=0;
return(res);
}
void print_date(date_t da)
{
printf("\n%d-%d-%d", da.month, da.day, da.year);
}
void print_tank(tank_t ta)
{
printf("\n%2.2lf %2.2lf\n", ta.capacity, ta.curent_Fuel);
}
void print_auto(auto_t au)
{
printf("\nVehicle \n%s %s %d %d %d %d %d %d %d %lf %lf\n", au.make,
au.model,
au.odometer,
au.manufact.month,
au.manufact.day,
au.manufact.year,
au.purchase.month,
au.purchase.day,
au.purchase.year,
au.tank.capacity,
au.tank.curent_Fuel);
}
我在您的代码中不了解的几件事可能会给您带来麻烦:
if (Car_data==NULL){
printf("ERROR: File failed to open");
getch();
exit(0);
fclose(Car_data);}
为什么在处理错误时使用exit(0)? 可能会检查这个 。
if(stat==11)
print_auto(car);
// rest of the code
除了缩进,我不确定在这里是否能做到这一点, 如果(stat == 11) ,则只会执行print_auto(car) 。 如果您希望条件和循环包含多于一行代码,则需要执行此操作:
if(condition)
{
// code
}
else
{
// code
}
loop()
{
// code
}
我认为您的错误很有可能来自此。
这不是真正的答案,但是格式化代码的方式很容易出错:
例如这段代码:
res=scanf("%lf %lf", &(*tank).capacity, &(*tank).curent_Fuel);
if(res==2)
res=1;
else if(res !=EOF)
res=0;
return(res);
应该这样格式化:
res=scanf("%lf %lf", &(*tank).capacity, &(*tank).curent_Fuel);
if(res==2)
res=1;
else if(res !=EOF)
res=0;
return(res);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.