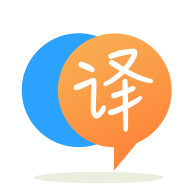
[英]Read a String from a txt file and store it into a char array in java
[英]Inputting string read from a .txt file into an array
我正在为我的高中软件项目制作像应用程序这样的抽认卡。 我能够通过将单词和它们各自的翻译写入文件来存储它们,但是我想知道是否存在一种将它们读入2d数组的方法。
我可以用逗号或其他字符分隔它们吗?
此外,还有一种方法可以链接单词及其相应的翻译。 例如,如果我将单词“ x”称为数组中的单词“ translated x”,是否有函数呢?
谢谢堆!
您可能想看看地图。 这样,您可以通过单词本身查找每个单词,而不用遍历数组。 映射使用键值对。 不幸的是,它们是通用的(您不能通过键的值来查找键)。 https://docs.oracle.com/javase/7/docs/api/java/util/Map.html
让我们来解决这个问题。
word
和一个translation
word
和translation
存储在数据结构中(@Glen Pierce关于使用地图的建议是一个很好的建议) 假设我们的文件如下所示,我们使用逗号来分隔单词和翻译(这也是我的西班牙语词汇的范围):
hello,hola
good,bueno
现在提供一些代码,让我们将文件读入地图。
// a map of word to translation
Map<String, String> wordMap = new HashMap<String, String>();
// a class that can read a file (we wrap the file reader in a buffered reader because it's more efficient to read a file in chunks larger than a single character)
BufferedReader fileReader = new BufferedReader(new FileReader("my-file.txt"));
// a line from the file
String line;
// read lines until we read a line that is null (i.e. no more lines)
while((line = fileReader.getLine()) != null) {
// split the line, returns an array of parts
String[] parts = line.split(",");
// store the parts in meaningful variables
String word = parts[0];
String translation = parts[1];
// now, store the word and the translation in the word map
wordMap.put(word, translation);
}
// close the reader (note: you should do this with a try/finally block so that if you throw an exception, you still close the reader)
fileReader.close();
现在,我们有了一个地图,其中包含文件中的所有单词和翻译。 给定单词,您可以像这样检索翻译:
String word = "hello";
String translation = wordMap.get(word);
System.out.println(word + " translates to " + translation);
输出:
hello translates to hola
我想下一步是让用户给您一个单词,并让您返回正确的翻译。 我留给你。
您是否需要将单词存储在文本文件中(即它们需要保留),还是可以将它们存储在内存中? 如果需要将它们写入文本文件,请尝试以下操作:
// Create a file
File file = new File("file.txt");
// Initialize a print writer to print to the file
PrintWriter pw = new PrintWriter(file);
Scanner keyboard = new Scanner(System.in);
// Populate
boolean stop = false;
do {
String word;
String translation;
System.out.print("Enter a word: ");
word = keyboard.nextLine().trim() + " ";
if (!word.equals("quit ")) {
pw.print(word);
System.out.print("Enter its translation: ");
translation = keyboard.nextLine().trim();
pw.println(translation);
} else {
stop = true;
}
} while (!stop);
// Close the print writer and write to the file
pw.close();
// Initialize a scanner to read the file
Scanner fileReader = new Scanner(file);
// Initialize a hash table to store the values from the file
Hashtable<String, String> words = new Hashtable<String, String>();
// Add the information from the file to the hash table
while (fileReader.hasNextLine()) {
String line = fileReader.nextLine();
String[] array = line.split(" ");
words.put(array[0], array[1]);
}
// Print the results
System.out.println("Results: ");
words.forEach((k, v) -> System.out.println(k + " " + v));
fileReader.close();
keyboard.close();
请注意,我在使用空格将单词与译文分开。 您可以轻松使用逗号,分号或其他内容。 只需将line.split(" ")
替换为line.split(< your separating character here>)
并将其连接到word = keyboard.nextLine().trim()
的末尾。
如果您不需要保存信息,而只需要收集用户的输入,则更为简单:
Scanner keyboard = new Scanner(System.in);
// Initialize a hash table to store the values from the user
Hashtable<String, String> words = new Hashtable<String, String>();
// Get the input from the user
boolean stop = false;
do {
String word;
String translation;
System.out.print("Enter a word: ");
word = keyboard.nextLine().trim();
if (!word.equals("quit")) {
System.out.print("Enter its translation: ");
translation = keyboard.nextLine().trim();
words.put(word, translation);
} else {
stop = true;
}
} while (!stop);
// Print the results
System.out.println("Results: ");
words.forEach((k, v) -> System.out.println(k + " " + v));
keyboard.close();
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.