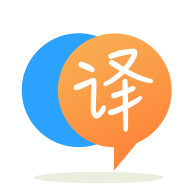
[英]Read a String from a txt file and store it into a char array in java
[英]Inputting string read from a .txt file into an array
我正在為我的高中軟件項目制作像應用程序這樣的抽認卡。 我能夠通過將單詞和它們各自的翻譯寫入文件來存儲它們,但是我想知道是否存在一種將它們讀入2d數組的方法。
我可以用逗號或其他字符分隔它們嗎?
此外,還有一種方法可以鏈接單詞及其相應的翻譯。 例如,如果我將單詞“ x”稱為數組中的單詞“ translated x”,是否有函數呢?
謝謝堆!
您可能想看看地圖。 這樣,您可以通過單詞本身查找每個單詞,而不用遍歷數組。 映射使用鍵值對。 不幸的是,它們是通用的(您不能通過鍵的值來查找鍵)。 https://docs.oracle.com/javase/7/docs/api/java/util/Map.html
讓我們來解決這個問題。
word
和一個translation
word
和translation
存儲在數據結構中(@Glen Pierce關於使用地圖的建議是一個很好的建議) 假設我們的文件如下所示,我們使用逗號來分隔單詞和翻譯(這也是我的西班牙語詞匯的范圍):
hello,hola
good,bueno
現在提供一些代碼,讓我們將文件讀入地圖。
// a map of word to translation
Map<String, String> wordMap = new HashMap<String, String>();
// a class that can read a file (we wrap the file reader in a buffered reader because it's more efficient to read a file in chunks larger than a single character)
BufferedReader fileReader = new BufferedReader(new FileReader("my-file.txt"));
// a line from the file
String line;
// read lines until we read a line that is null (i.e. no more lines)
while((line = fileReader.getLine()) != null) {
// split the line, returns an array of parts
String[] parts = line.split(",");
// store the parts in meaningful variables
String word = parts[0];
String translation = parts[1];
// now, store the word and the translation in the word map
wordMap.put(word, translation);
}
// close the reader (note: you should do this with a try/finally block so that if you throw an exception, you still close the reader)
fileReader.close();
現在,我們有了一個地圖,其中包含文件中的所有單詞和翻譯。 給定單詞,您可以像這樣檢索翻譯:
String word = "hello";
String translation = wordMap.get(word);
System.out.println(word + " translates to " + translation);
輸出:
hello translates to hola
我想下一步是讓用戶給您一個單詞,並讓您返回正確的翻譯。 我留給你。
您是否需要將單詞存儲在文本文件中(即它們需要保留),還是可以將它們存儲在內存中? 如果需要將它們寫入文本文件,請嘗試以下操作:
// Create a file
File file = new File("file.txt");
// Initialize a print writer to print to the file
PrintWriter pw = new PrintWriter(file);
Scanner keyboard = new Scanner(System.in);
// Populate
boolean stop = false;
do {
String word;
String translation;
System.out.print("Enter a word: ");
word = keyboard.nextLine().trim() + " ";
if (!word.equals("quit ")) {
pw.print(word);
System.out.print("Enter its translation: ");
translation = keyboard.nextLine().trim();
pw.println(translation);
} else {
stop = true;
}
} while (!stop);
// Close the print writer and write to the file
pw.close();
// Initialize a scanner to read the file
Scanner fileReader = new Scanner(file);
// Initialize a hash table to store the values from the file
Hashtable<String, String> words = new Hashtable<String, String>();
// Add the information from the file to the hash table
while (fileReader.hasNextLine()) {
String line = fileReader.nextLine();
String[] array = line.split(" ");
words.put(array[0], array[1]);
}
// Print the results
System.out.println("Results: ");
words.forEach((k, v) -> System.out.println(k + " " + v));
fileReader.close();
keyboard.close();
請注意,我在使用空格將單詞與譯文分開。 您可以輕松使用逗號,分號或其他內容。 只需將line.split(" ")
替換為line.split(< your separating character here>)
並將其連接到word = keyboard.nextLine().trim()
的末尾。
如果您不需要保存信息,而只需要收集用戶的輸入,則更為簡單:
Scanner keyboard = new Scanner(System.in);
// Initialize a hash table to store the values from the user
Hashtable<String, String> words = new Hashtable<String, String>();
// Get the input from the user
boolean stop = false;
do {
String word;
String translation;
System.out.print("Enter a word: ");
word = keyboard.nextLine().trim();
if (!word.equals("quit")) {
System.out.print("Enter its translation: ");
translation = keyboard.nextLine().trim();
words.put(word, translation);
} else {
stop = true;
}
} while (!stop);
// Print the results
System.out.println("Results: ");
words.forEach((k, v) -> System.out.println(k + " " + v));
keyboard.close();
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.