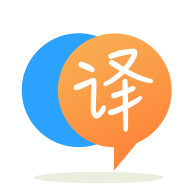
[英]How to add and remove object from vector<unique_ptr>...?
[英]How add/remove to/from vector<unique_ptr>?
我使用unique_ptr
而不是shared_ptr
因为它不计算引用,并且速度更快。 您可以将其更改为shared_ptr
。 我的问题是如何向智能指针向量正确添加/删除? 关键行在这里:
CEO->add(std::move(headSales));
CEO->remove(headSales);
码:
#ifndef EMPLOYEE_H
#define EMPLOYEE_H
#include <iostream>
#include <vector>
#include <memory>
class Employee
{
protected:
std::string name;
std::string department;
std::uint32_t salary;
std::vector<std::unique_ptr<Employee>> subordinates;
public:
Employee(std::string n, std::string d, std::uint32_t s) : name(n), department(d), salary(s)
{}
bool operator==(const Employee &e)
{
return name == e.name && department == e.department && salary == e.salary;
}
void add(std::unique_ptr<Employee> e)
{
subordinates.push_back(e);
}
void remove(const std::unique_ptr<Employee> e)
{
subordinates.erase(std::remove(subordinates.begin(), subordinates.end(), e), subordinates.end());
}
std::vector<std::unique_ptr<Employee>> getSubordinates() const
{
return subordinates;
}
};
#endif //EMPLOYEE_H
#include "Employee.h"
int main()
{
std::unique_ptr<Employee> CEO = std::make_shared<Employee>("John", "CEO", 30000);
std::unique_ptr<Employee> headSales = std::make_shared<Employee>("Robert", "Head Sales", 20000);
CEO->add(std::move(headSales));
CEO->remove(headSales);
}
您的代码不需要唯一或共享的指针。
#ifndef EMPLOYEE_H
#define EMPLOYEE_H
#include <iostream>
#include <vector>
#include <memory>
class Employee
{
protected:
std::string name;
std::string department;
std::uint32_t salary;
std::vector<Employee> subordinates;
public:
Employee(std::string n, std::string d, std::uint32_t s) : name(n), department(d), salary(s)
{}
bool operator==(const Employee &e) const
{
return name == e.name && department == e.department && salary == e.salary && subordinates == e.subordinates;
}
void add(Employee e)
{
subordinates.push_back(std::move(e));
}
void remove(Employee const& e)
{
subordinates.erase(std::remove(subordinates.begin(), subordinates.end(), e), subordinates.end());
}
std::vector<Employee> getSubordinates() const
{
return subordinates;
}
};
#endif //EMPLOYEE_H
#include "Employee.h"
int main()
{
Employee CEO = {"John", "CEO", 30000};
Employee headSales = {"Robert", "Head Sales", 20000};
CEO->add(headSales);
CEO->remove(headSales);
}
同样,您的==
应该是const
,并且应该比较subordinates
。 因为它现在是Employee
的向量,所以它递归调用==
。
std::unique_ptr<Employee> CEO = std::make_shared<Employee>("John", "CEO", 30000); ^^^^^^ ^^^^^^
这是错误的,因为共享指针不能转换为唯一指针。 尚不清楚您打算拥有共享所有权还是唯一所有权。
CEO->remove(headSales); subordinates.push_back(e);
这些是错误的,因为您要复制唯一的指针。 但是,唯一指针不可复制-否则它们将不会保持唯一。 相反,您必须从原始指针移开:
subordinates.push_back(std::move(e));
首先,使用唯一的指针作为Employee::remove
的值参数是不明智的。 下文提供了更多信息。
std::vector<std::unique_ptr<Employee>> getSubordinates() const { return subordinates; }
这次,您尝试复制唯一指针的向量。 问题仍然相同-指针不可复制。 因为函数是const,所以也不能移动向量。 也许您打算只授予读取权限。 这可以通过返回引用来实现:
const std::vector<std::unique_ptr<Employee>>& getSubordinates() const
CEO->add(std::move(headSales)); CEO->remove(headSales);
您使用的是已经移动过的指针。 唯一指针的所有权已唯一地转移到add
,因此在移动之后使用它不再有意义。
正如我所提到的,使用唯一指针作为按值作为remove的参数没有意义。 为了拥有唯一的指针,您将必须拥有该指针-唯一。 因此,向量不可能拥有它。 您可以改为引用向量中包含的指针,并使用该指针:
void remove(const std::unique_ptr<Employee>& e)
CEO->remove(CEO->getSubordinates().back());
您可以将其更改为
shared_ptr
。
好吧,那会简单得多。 将所有unique
替换为shared
,然后删除该std::move
,程序将格式正确。
PS。 您想要的std::remove
位于您忘记包含的<algorithm>
标头中。
PPS。 对我来说,目前尚不清楚为什么一个雇员应该拥有其他雇员的任何所有权-唯一或共享。 我建议所有雇员都由其他人拥有-例如Company
,而雇员仅具有非所有者协会。 我建议std::weak_ptr
。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.