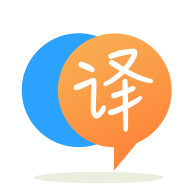
[英]Cloned jquery elements are triggering events to parent <div> instead of child<div>?
[英]jQuery incrementing a cloned elements instead of cloned div
我有一个HTML脚本,其中包含一个下拉列表和一个文本框,我只需要克隆这两个而不是整个div,然后将数据发送到AJAX,每个带有文本框的下拉列表将形成一个数组,该数组应被添加为表中的一行,这就是我现在所拥有的:
<div class="col-sm-4 rounded" style="background-color: #D3D3D3">
<div class="row clonedInput" id="clonedInput1">
<div class="col-sm-6 ">
<label for="diagnosis_data">Medication</label>
<fieldset class="form-group">
<select class="form-control select" name="diagnosis_data" id="diagnosis_data">
<option value="choose">Select</option>
</select>
</fieldset>
<!-- End class="col-sm-6" -->
</div>
<div class="col-sm-6">
<label for="medication_quantity">Quantity</label>
<fieldset class="form-group">
<input type="number" class="form-control" name="medication_quantity" id="medication_quantity">
</fieldset>
<!-- End class="col-sm-6" -->
</div>
<!-- End class="col-sm-6" -->
</div>
<div class="actions pull-right">
<button class="btn btn-danger clone">Add More</button>
<button class="btn btn-danger remove">Remove</button>
</div>
<!-- End class="col-sm-4" -->
</div>
这是jQuery脚本:
$(document).ready(function()
{
$("button.clone").on("click", clone);
$("button.remove").on("click", remove);
})
var regex = /^(.+?)(\d+)$/i;
var cloneIndex = $(".clonedInput").length;
function clone(){
$(this).closest(".rounded").clone()
.insertAfter(".rounded:last")
.attr("id", "rounded" + (cloneIndex+1))
.find("*")
.each(function() {
var id = this.id || "";
var match = id.match(regex) || [];
if (match.length == 3) {
this.id = id.split('-')[0] +'-'+(cloneIndex);
}
})
.on('click', 'button.clone', clone)
.on('click', 'button.remove', remove);
cloneIndex++;
}
function remove(){
$(this).parent().parent(".rounded").remove();
}
问题是整个div都将被克隆,而div id才被更改:
这是每个div的ID递增:
我只需要克隆2个元素,而不是整个div和按钮
最后,我不需要使用Ajax和PHP将它们添加到数据库中
在这里,您可以使用代码。
在这段代码中,我对clone()
进行了更改
这里的变化
var cloneIndex = $(".clonedInput").length;
这应该在clone()
因此它将在克隆的html中将子元素的正确增量值作为id
传递 下面的代码只是使clonedInput
克隆不是整个div
编辑
我也编辑删除功能。
它只会删除被克隆的最后一个元素。
希望这对您有帮助。 :)
$(document).ready(function() { $("button.clone").on("click", clone); $("button.remove").on("click", remove); }); var regex = /^(.+?)(\\d+)$/i; function clone() { var cloneIndex = $(".clonedInput").length; $(".rounded").find("#clonedInput1").clone().insertAfter(".clonedInput:last").attr("id", "clonedInput" + (cloneIndex+1)); } function remove() { $(".rounded").find(".clonedInput:last").remove(); }
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> <div class="col-sm-4 rounded" style="background-color: #D3D3D3"> <div class="row clonedInput" id="clonedInput1"> <div class="col-sm-6 "> <label for="diagnosis_data">Medication</label> <fieldset class="form-group"> <select class="form-control select" name="diagnosis_data" id="diagnosis_data"> <option value="choose">Select</option> </select> </fieldset> <!-- End class="col-sm-6" --> </div> <div class="col-sm-6"> <label for="medication_quantity">Quantity</label> <fieldset class="form-group"> <input type="number" class="form-control" name="medication_quantity" id="medication_quantity"> </fieldset> <!-- End class="col-sm-6" --> </div> <!-- End class="col-sm-6" --> </div> <div class="actions pull-right"> <button class="btn btn-danger clone">Add More</button> <button class="btn btn-danger remove">Remove</button> </div> <!-- End class="col-sm-4" --> </div>
您可以向actions
类添加样式,以防止其显示在所有克隆的元素上
的CSS
.actions {
display: none;
}
.clonedInput:first-child .actions {
display: block;
}
同样对于删除功能,您可以使用.closest()
代替.parent().parent()
$(this).closest(".rounded").remove();
有很多东西可以优化和替换,但是我已经编辑了您的代码。 我相信这是最简单的学习方法。 这些编辑在注释中标记为“ STACKOVERFLOW EDIT”。
$(document).ready(function() {
$("button.clone").on("click", clone);
$("button.remove").on("click", remove);
$("button.submit").on("click", submit_form); // STACKOVERFLOW EDIT: execute the submit function
});
var regex = /^(.+?)(\d+)$/i;
function clone() {
var cloneIndex = $(".clonedInput").length;
$(".rounded").find("#clonedInput1").clone().insertAfter(".clonedInput:last").attr("id", "clonedInput" + (cloneIndex + 1));
}
function remove() {
if($(".clonedInput").length > 1) { // STACKOVERFLOW EDIT: Make sure that you will not remove the first div (the one thet you clone)
$(".rounded").find(".clonedInput:last").remove();
} // STACKOVERFLOW EDIT
}
// STACKOVERFLOW EDIT: define the submit function to be able to sent the data
function submit_form() {
var ajax_data = $('#submit_form').serialize(); // The data of your form
$.ajax({
type: "POST",
url: 'path_to_your_script.php', // This URL should be accessable by web browser. It will proccess the form data and save it to the database.
data: ajax_data,
success: function(ajax_result){ // The result of your ajax request
alert(ajax_result); // Process the result the way you whant to
},
});
}
HTML:
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="col-sm-4 rounded" style="background-color: #D3D3D3">
<form action="" method="post" id="submit_form"> <!-- STACKOVERFLOW EDIT: generate a form to allow you to get the data in easy way -->
<div class="row clonedInput" id="clonedInput1">
<div class="col-sm-6 ">
<label for="diagnosis_data">Medication</label>
<fieldset class="form-group">
<select class="form-control select" name="diagnosis_data[]" id="diagnosis_data"> <!-- STACKOVERFLOW EDIT: Add [] so that you may receive the values as arrays -->
<option value="choose">Select</option>
</select>
</fieldset>
<!-- End class="col-sm-6" -->
</div>
<div class="col-sm-6">
<label for="medication_quantity">Quantity</label>
<fieldset class="form-group">
<input type="number" class="form-control" name="medication_quantity[]" id="medication_quantity"> <!-- STACKOVERFLOW EDIT: Add [] so that you may receive the values as arrays -->
</fieldset>
<!-- End class="col-sm-6" -->
</div>
<!-- End class="col-sm-6" -->
</div>
</form> <!-- STACKOVERFLOW EDIT -->
<div class="actions pull-right">
<button class="btn btn-danger clone">Add More</button>
<button class="btn btn-danger remove">Remove</button>
<button class="btn btn-danger submit">Submit</button>
</div>
<!-- End class="col-sm-4" -->
</div>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.