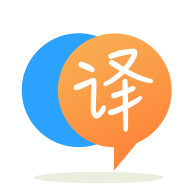
[英]Progressbar (ttk.Progressbar) with python in tkinter not showing
[英]Reverse ttk.Progressbar()
很好奇如何将ttk.Progressbar()填充到最大值(用户指定的任何数字),并以指定的增量逐步减小条形。 因此,请使用100%填充的条(例如,用户将100指定为最大值),并每15秒将其降低25%。 这是我所拥有的:
#import module for update frequency and gui. ttk for progressbar
import threading,ttk
from Tkinter import *
#establishs counter for testing purposes
counter = 0
#establish window
root = Tk()
def main():
#user inputs health
health = float(input("Enter health:"))
#user inputs how much damage each bullet does
dps = float(input("Enter Damage per shot:"))
#user inputs the fire rate of the weapon
spm = float(input("Enter Fire Rate:"))
#from user inputs establish how many shots it takes to reduce health to or below zero
if ((health / dps).is_integer()) is False: #checks if the stk value will be a float
stk = int(health / dps) + 1 #since stk value is a float go up to next whole number. 33dps doesn't kill in 3 shots.
else: #if stk value is an integer, establishes stk variable
stk = health / dps
delay_in_seconds = float(60 / spm)
#establishes the time to kill in seconds, take one from stk to account for delay of gunfire
ttki = ((stk - 1) * delay_in_seconds)
# establish progressbar
progre = ttk.Progressbar(root, orient='horizontal', maximum=health, mode='determinate')
progre.pack(fill=X)
# test on how to test for frequency of updating GUI once I figure out how in the heck to build it
def DPS_Timer():
global counter
print counter
if counter != (stk-1):
counter += 1
progre.step(float((health/stk)))
root.after(int(ttki*1000/stk), DPS_Timer)
else:
progre.stop()
# establish GUI Button
B1 = Button(root, text='start', command=DPS_Timer).pack(fill=X)
root.mainloop()
main()
所以基本上我想要这部分代码
# establish progressbar
progre = ttk.Progressbar(root, orient='horizontal', maximum=health, mode='determinate')
progre.pack(fill=X)
# test on how to test for frequency of updating GUI once I figure out how in the heck to build it
def DPS_Timer():
global counter
print counter
if counter != (stk-1):
counter += 1
progre.step(float((health/stk)))
root.after(int(ttki*1000/stk), DPS_Timer)
else:
progre.stop()
# establish GUI Button
B1 = Button(root, text='start', command=DPS_Timer).pack(fill=X)
这样说:“嘿ttk.Progressbar(),以100% /健康的最大值描述该条,我需要您以此速率在此步长值处减小它。” 这也可能提供一些我没有提供的见解: http : //infohost.nmt.edu/tcc/help/pubs/tkinter/web/ttk-Progressbar.html和https://docs.python.org/ 2 / library / ttk.html#index-0
原始代码已修改为基于GUI的代码,因此这是我的新代码,该代码存在一个问题,即在选择“开始”按钮之前,ttk.Progressbar()会初始化为已经缺少的块。 只是要指定,当选择开始按钮时,即使在删除第一个块之前,也需要延迟一段时间。
#import module for update frequency and gui. ttk for progressbar
import ttk
from Tkinter import *
#establish window
root = Tk()
E1 = Entry(root)
E2 = Entry(root)
E3 = Entry(root)
global health, dps, spm, ttki, stk, delay_in_seconds, progre, counter
def captcha_health():
global health, dps, spm, ttki, stk, delay_in_seconds, progre, counter
try:
health = float(E1.get())
except ValueError:
exit()
Entry2()
def captcha_dps():
global health, dps, spm, ttki, stk, delay_in_seconds, progre, counter
try:
dps = float(E2.get())
except ValueError:
exit()
Entry3()
def captcha_spm():
global health, dps, spm, ttki, stk, delay_in_seconds, progre, counter
try:
spm = float(E3.get())
except ValueError:
exit()
estvar()
def Entry2():
global health, dps, spm, ttki, stk, delay_in_seconds, progre, counter
# user inputs how much damage each bullet does
E2.grid(sticky='we')
DB = Button(root, text='enter damage/shot', command=captcha_dps).grid()
def Entry3():
global health, dps, spm, ttki, stk, delay_in_seconds, progre, counter
# user inputs the fire rate of the weapon
E3.grid(sticky='we')
SB = Button(root, text='enter fire rate', command=captcha_spm).grid()
def estvar():
global health, dps, spm, ttki, stk, delay_in_seconds, progre, counter
# establishs counter for testing purposes
counter = 0
# from user inputs establish how many shots it takes to reduce health to or below zero
if ((health / dps).is_integer()) is False: # checks if the stk value will be a float
stk = int(
health / dps) + 1 # since stk value is a float go up to next whole number. 33dps doesn't kill in 3 shots.
else: # if stk value is an integer, establishes stk variable
stk = health / dps
delay_in_seconds = float(60 / spm)
# establishes the time to kill in seconds, take one from stk to account for delay of gunfire
ttki = ((stk - 1) * delay_in_seconds)
guiest()
def DPS_Timer():
global health, dps, spm, ttki, stk, delay_in_seconds, progre, counter
counter += 1
progre.step(-1*dps)
if counter < stk:
root.after(int(ttki*1000/stk), DPS_Timer)
def guiest():
global health, dps, spm, ttki, stk, delay_in_seconds, progre, counter
# establish GUI Button
ttkLabel = Label(root, text='Time to kill: ' + str(ttki)).grid(sticky=W)
stkLabel = Label(root, text='Shots to kill: ' + str(stk)).grid(sticky=W)
hhLabel = Label(root, text='Health: ' + str(health)).grid(sticky=W)
dpsLabel = Label(root, text='Damage/shot: ' + str(dps)).grid(sticky=W)
spmLabel = Label(root, text='Fire rate: ' + str(spm)).grid(sticky=W)
delayLabel = Label(root, text='Delay between shots: ' + str(delay_in_seconds)).grid(sticky=W)
B1 = Button(root, text='start', command=DPS_Timer).grid(sticky='we')
# establish progressbar
progre = ttk.Progressbar(root, orient='horizontal', maximum=health, mode='determinate')
progre.grid(sticky='we')
progre.step(health-1)
#user inputs health
E1.grid(sticky='we')
HB = Button(root, text='enter health value', command=captcha_health).grid()
root.mainloop()
为了将健康指标设置为100%,我们可以使用
progre.step(health - 1)
就在 DPS_Timer()函数的上方 。 从那里开始,除了步长值外,您的功能大部分都是正确的。 我们希望该步骤为负值,因为我们正在减小运行状况栏的值。 同样,除非我们在某个时候使用.start(),否则.stop()是没有用的,因此我们可以完全删除该代码块。 一旦counter等于stk,就不会再调用root.after(),因此我们不需要else语句。 这就是给我们的:
def DPS_Timer():
global counter
print(counter)
if counter < stk:
counter += 1
progre.step(-1*health/stk)
root.after(int(ttki*1000/stk), DPS_Timer)
另外,您的progre.step()并不能真正消除dps造成的损害。 我们可以用
progre.step(health - 1)
def DPS_Timer():
global counter
print(counter)
counter += 1
progre.step(-1*dps)
if counter < stk:
root.after(int(ttki*100/stk), DPS_Timer)
(我移动了if语句,因此不叫DPS_Timer额外的时间)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.