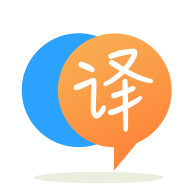
[英]How do I get a variable in one function to another function in python?
[英]How do I make a variable from another function work on another Python?
所以这是我的整个代码,当我说window_Calculate()
没有定义高度时,我遇到了麻烦。 我的目标是使用公式50 + (height * width) * 100
。 在window_Size
,用户必须输入height
和width
但是如果他们键入不是数字的任何内容,则应该返回该height
和width
,以便他们可以输入实际数字。 总体而言,在其他功能中未定义height
,并且如果用户输入数字以外的任何内容, height
都不会返回到输入。 如何定义height
并返回输入?
import time
def Intro():
print("Welcome to Wendy's Windows.")
time.sleep(2)
print("This is a window replacement business.")
time.sleep(2)
print("Enter your size in the box on the left and the price will be displayed below.")
time.sleep(2.5)
def window_Size():
print("Put height first then put width.")
time.sleep(1.5)
height = input("Height: ")
try:
int(height)
except ValueError:
try:
float(height)
except ValueError:
print("Enter only numbers.")
return(height)
else:
print(height + "m")
width = input("Width: ")
try:
int(width)
except ValueError:
try:
float(width)
except ValueError:
print("Enter only numbers.")
return(width)
else:
print(width + "m")
def window_Calculate():
window_Calculate = '50' + (height * width) * '100'
def window_Cost():
print("Your price is" + window_Calculate + "$")
window_Size()
window_Calculate()
window_Cost()
因此,这里有几件事:
window_Size()
的try/except
类型测试 window_Size()
调用return(height)
但不存储它 window_Calculate()
您正在尝试使用的是所谓的global variable
。 在几乎任何编程语言中,都不鼓励使用它们。 相反,你应该使用一个local variable
被称为函数内作用域return
可变后向上级调用函数。
下面,我指出了您程序中的一些亮点,并给出了更改后的程序再现。 虽然是一种解决方案,但我不了解您对这些概念有多少了解,因此对您的程序进行一点点修改即可清楚地说明要点。 但是,可以对其进行显着更改,使其更加Python化和完善。
1号:
def window_Size():
print("Put height first then put width.")
time.sleep(1.5) # bit annoying to sleep all the time?
height = input("Height: ")
try:
int(height) # type test of int, that's fine
except ValueError: # correct catch
try:
float(height) # type test of float, that's fine
except ValueError: # correct catch; must be invalid
print("Enter only numbers.") # message for user
return(height) # wait why did you return??
else: # wont be executed if above return() is called (i.e. for str())
print(height + "m")
width = input("Width: ")
try:
int(width)
except ValueError:
try:
float(width)
except ValueError:
print("Enter only numbers.")
return(width) # All the same notes above apply
else:
print(width + "m")
因此,您使用的try/except/else
很好,但是在单个函数中有2个return()
调用; 一被调用, window_Size()
将立即退出(即,如果调用return(height)
则不width
。此外,要返回多个值,可以使用tuple
。
2号:
window_Size() # this functions returns values, but isnt storing
3号
window_Calculate() # this functions uses returns values, but isnt passed any
解:
import time
def intro():
print("Welcome to Wendy's Windows.")
time.sleep(2) # personally I'd get rid of all time.sleep() calls
print("This is a window replacement business.")
time.sleep(2)
print("Enter your size in the box on the left and the price will be displayed below.")
time.sleep(2.5)
process_customer()
def process_customer():
size = window_size()
# get rid of window_calculate() all together
total = window_cost(size)
print("Your price is" + str(total) + "$")
def window_size():
print("Put height first then put width.")
while True:
height = input("Height: ")
try:
h = int(height)
break
except ValueError:
try:
h = float(height)
break
except ValueError:
print("Enter only numbers.")
print(height + "m")
while True:
width = input("Width: ")
try:
w = int(width)
break
except ValueError:
try:
w = float(width)
break
except ValueError:
print("Enter only numbers.")
print(width + "m")
return h, w
def window_cost(size):
return 50 + (size[0] + size[1]) * 100 # before 50 and 100 were str()
intro()
您应该确保height,width和window_Calculate是全局变量,以便可以在A函数中更改它们,并在B函数中使用它们。
pstatix的答案是最好的解决方案,应该接受
您需要每个方法都可以获取或设置“全局”变量。 尝试这个:
import time
window_height = 0
window_width = 0
window_value = 0
def Intro():
print("Welcome to Wendy's Windows.")
time.sleep(2)
print("This is a window replacement business.")
time.sleep(2)
print("Enter your size in the box on the left and the price will be displayed below.")
time.sleep(2.5)
def window_Size():
global window_height
global window_width
print("Put height first then put width.")
time.sleep(1.5)
height = input("Height: ")
try:
int(height)
except ValueError:
try:
float(height)
except ValueError:
print("Enter only numbers.")
return(height)
else:
print(str(height) + "m")
window_height = height
width = input("Width: ")
try:
int(width)
except ValueError:
try:
float(width)
except ValueError:
print("Enter only numbers.")
return(width)
else:
print(str(width) + "m")
window_width = width
def window_Calculate():
global window_value
window_value = 50 + window_height * window_width * 100
def window_Cost():
print("Your price is $" + str(window_value))
window_Size()
window_Calculate()
window_Cost()
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.