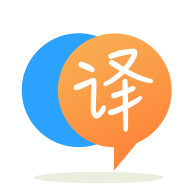
[英]C++ need help figuring out word count in function. (Ex. Hello World = 2)
[英]How to “Fold a word” from a string. EX. “STACK” becomes “SKTCA”. C++
我试图弄清楚如何从字符串中折叠单词。 例如,折叠后的“代码”将变为“ ceod”。 基本上从第一个字符开始,然后得到最后一个,然后是第二个字符。 我知道第一步是从循环开始,但我不知道该如何获得最后一个字符。 任何帮助都会很棒。 这是我的代码。
#include <iostream>
using namespace std;
int main () {
string fold;
cout << "Enter a word: ";
cin >> fold;
string temp;
string backwards;
string wrap;
for (unsigned int i = 0; i < fold.length(); i++){
temp = temp + fold[i];
}
backwards= string(temp.rbegin(),temp.rend());
for(unsigned int i = 0; i < temp.length(); i++) {
wrap = fold.replace(backwards[i]);
}
cout << wrap;
}
谢谢
@Supreme,有很多方法可以完成您的任务,我将发布其中一种方法。 但是正如@John指出的那样,您必须尝试自己完成它,因为真正的编程要花很多精力。 使用此解决方案作为一种可能性的参考,并找到许多其他可能性。
int main()
{
string in;
cout <<"enter: "; cin >> in;
string fold;
for (int i=0, j=in.length()-1; i<in.length()/2; i++, j--)
{
fold += in[i];
fold += in[j];
}
if( in.length()%2 != 0) // if string lenght is odd, pick the middle
fold += in[in.length()/2];
cout << endl << fold ;
return 0;
}
祝好运 !
解决这种形式的问题有两种方法,数学上精确的方法是创建一个生成器函数,该函数以正确的顺序返回数字。
一个更简单的计划是修改字符串以实际解决该问题。
我们需要一个返回字符串中索引的函数。 我们有2个序列-递增和递减,它们是交错的。
顺序1:
0, 1 , 2, 3.
顺序2
len-1, len-2, len-3, len-4.
给定它们是交错的,我们希望偶数值来自序列1,奇数值来自序列2。
因此,我们的解决方案是对于给定的新索引,选择要使用的序列,然后从该序列返回下一个值。
int generator( int idx, int len )
{
ASSERT( idx < len );
if( idx %2 == 0 ) { // even - first sequence
return idx/2;
} else {
return (len- (1 + idx/2);
}
}
然后可以从函数折叠中调用它。
std::string fold(const char * src)
{
std::string result;
std::string source(src);
for (size_t i = 0; i < source.length(); i++) {
result += source.at(generator(i, source.length()));
}
return result;
}
尽管效率较低,但可能更容易考虑。 我们正在获取字符串的第一个或最后一个字符。 我们将使用字符串操作来获得正确的结果。
std::string fold2(const char * src)
{
std::string source = src;
enum whereToTake { fromStart, fromEnd };
std::string result;
enum whereToTake next = fromStart;
while (source.length() > 0) {
if (next == fromStart) {
result += source.at(0);
source = source.substr(1);
next = fromEnd;
}
else {
result += source.at(source.length() - 1); // last char
source = source.substr(0, source.length() - 1); // eat last char
next = fromStart;
}
}
return result;
}
您可以利用反向迭代器的概念来编写基于Usman Riaz答案中提供的解决方案的通用算法。
从原始字符串的两端组成采摘字符的字符串。 当您到达中心时,如果字符数为奇数,请在中间添加字符。
这是一个可能的实现:
#include <iostream>
#include <string>
#include <vector>
#include <utility>
#include <algorithm>
#include <iterator>
template <class ForwardIt, class OutputIt>
OutputIt fold(ForwardIt source, ForwardIt end, OutputIt output)
{
auto reverse_source = std::reverse_iterator<ForwardIt>(end);
auto reverse_source_end = std::reverse_iterator<ForwardIt>(source);
auto source_end = std::next(source, std::distance(source, end) / 2);
while ( source != source_end )
{
*output++ = *source++;
*output++ = *reverse_source++;
}
if ( source != reverse_source.base() )
{
*output++ = *source;
}
return output;
}
int main() {
std::vector<std::pair<std::string, std::string>> tests {
{"", ""}, {"a", "a"}, {"stack", "sktca"}, {"steack", "sktcea"}
};
for ( auto const &test : tests )
{
std::string result;
fold(
std::begin(test.first), std::end(test.first),
std::back_inserter(result)
);
std::cout << (result == test.second ? " OK " : "FAILED: ")
<< '\"' << test.first << "\" --> \"" << result << "\"\n";
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.