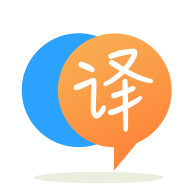
[英]get more than 10 documents from firestore in one round trip using Flutter
[英]Google Firestore - How to get several documents by multiple ids in one round-trip?
我想知道是否可以在到 Firestore 数据库的一次往返(网络调用)中通过 id 列表获取多个文档。
如果您在 Node 中:
https://github.com/googleapis/nodejs-firestore/blob/master/dev/src/index.ts#L978
/**
* Retrieves multiple documents from Firestore.
*
* @param {...DocumentReference} documents - The document references
* to receive.
* @returns {Promise<Array.<DocumentSnapshot>>} A Promise that
* contains an array with the resulting document snapshots.
*
* @example
* let documentRef1 = firestore.doc('col/doc1');
* let documentRef2 = firestore.doc('col/doc2');
*
* firestore.getAll(documentRef1, documentRef2).then(docs => {
* console.log(`First document: ${JSON.stringify(docs[0])}`);
* console.log(`Second document: ${JSON.stringify(docs[1])}`);
* });
*/
这是专门针对服务器SDK的
更新: “Cloud Firestore [客户端 sdk] 现在支持 IN 查询!”
https://firebase.googleblog.com/2019/11/cloud-firestore-now-supports-in-queries.html
myCollection.where(firestore.FieldPath.documentId(), 'in', ["123","456","789"])
他们刚刚宣布了这个功能, https://firebase.googleblog.com/2019/11/cloud-firestore-now-supports-in-queries.html 。
现在您可以使用类似的查询,但请注意输入大小不能大于 10。
userCollection.where('uid', 'in', ["1231","222","2131"])
在实践中,你会像这样使用 firestore.getAll
async getUsers({userIds}) {
const refs = userIds.map(id => this.firestore.doc(`users/${id}`))
const users = await this.firestore.getAll(...refs)
console.log(users.map(doc => doc.data()))
}
或使用承诺语法
getUsers({userIds}) {
const refs = userIds.map(id => this.firestore.doc(`users/${id}`))
this.firestore.getAll(...refs).then(users => console.log(users.map(doc => doc.data())))
}
你可以使用这样的函数:
function getById (path, ids) {
return firestore.getAll(
[].concat(ids).map(id => firestore.doc(`${path}/${id}`))
)
}
可以使用单个 ID 调用它:
getById('collection', 'some_id')
或一组 ID:
getById('collection', ['some_id', 'some_other_id'])
不,目前无法使用 Cloud Firestore SDK 批量处理多个读取请求,因此无法保证您可以一次读取所有数据。
然而,正如 Frank van Puffelen 在上面的评论中所说的,这并不意味着获取 3 个文档的速度是获取一个文档的 3 倍。 在得出结论之前,最好先进行自己的测量。
如果您正在使用颤振,您可以执行以下操作:
Firestore.instance.collection('your collection name').where(FieldPath.documentId, whereIn:[list containing multiple document IDs]).getDocuments();
这将返回一个包含List<DocumentSnapshot>
的 Future ,您可以根据需要对其进行迭代。
当然,最好的方法是在 Cloud Function 中实现 Firestore 的实际查询? 然后只有从客户端到 Firebase 的一次往返调用,这似乎正是您所要求的。
无论如何,您确实希望像此服务器端一样保留所有数据访问逻辑。
在内部,对 Firebase 本身的调用数量可能相同,但它们都将通过 Google 的超快速互连,而不是外部网络,并结合 Frank van Puffelen 解释的流水线,您应该获得出色的性能这种方法。
下面介绍如何使用 Android SDK 在 Kotlin 中执行此类操作。
可能不一定是一次往返,但它确实有效地对结果进行了分组并避免了许多嵌套的回调。
val userIds = listOf("123", "456")
val userTasks = userIds.map { firestore.document("users/${it!!}").get() }
Tasks.whenAllSuccess<DocumentSnapshot>(userTasks).addOnSuccessListener { documentList ->
//Do what you need to with the document list
}
请注意,获取特定文档比获取所有文档并过滤结果要好得多。 这是因为 Firestore 向您收取查询结果集的费用。
我希望这对你有帮助,它对我有用。
getCartGoodsData(id) {
const goodsIDs: string[] = [];
return new Promise((resolve) => {
this.fs.firestore.collection(`users/${id}/cart`).get()
.then(querySnapshot => {
querySnapshot.forEach(doc => {
goodsIDs.push(doc.id);
});
const getDocs = goodsIDs.map((id: string) => {
return this.fs.firestore.collection('goods').doc(id).get()
.then((docData) => {
return docData.data();
});
});
Promise.all(getDocs).then((goods: Goods[]) => {
resolve(goods);
});
});
});
}
对于那些想使用 Angular 做这件事的人,这里有一个例子:
首先需要一些库导入:(必须预先安装)
import * as firebase from 'firebase/app'
import { AngularFirestore, AngularFirestoreCollection } from '@angular/fire/firestore'
集合的一些配置:
yourCollection: AngularFirestoreCollection;
constructor(
private _db : AngularFirestore,
) {
// this is your firestore collection
this.yourCollection = this._db.collection('collectionName');
}
这是执行查询的方法:('products_id' 是一个 id 数组)
getProducts(products_ids) {
var queryId = firebase.firestore.FieldPath.documentId();
this.yourCollection.ref.where(queryId, 'in', products_ids).get()
.then(({ docs }) => {
console.log(docs.map(doc => doc.data()))
})
}
目前在 Firestore 中这似乎是不可能的。 我不明白为什么 Alexander 的回答被接受,他提出的解决方案只是返回“用户”集合中的所有文档。
根据您需要做什么,您应该考虑复制您需要显示的相关数据,并且仅在需要时才请求完整文档。
对的,这是可能的。 .NET SDK for Firestore 中的示例:
/*List of document references, for example:
FirestoreDb.Collection(ROOT_LEVEL_COLLECTION).Document(DOCUMENT_ID);*/
List<DocumentReference> docRefList = YOUR_DOCUMENT_REFERENCE_LIST;
// Required fields of documents, not necessary while fetching entire documents
FieldMask fieldMask = new FieldMask(FIELD-1, FIELD-2, ...);
// With field mask
List<DocumentSnapshot> documentSnapshotsMasked = await FirestoreDb.GetAllSnapshotsAsync(docRefList, fieldMask);
// Without field mask
List<DocumentSnapshot>documentSnapshots = await FirestoreDb.GetAllSnapshotsAsync(docRefList);
.NET 中的文档:
您可以使用文档 ID(最多十个)执行 IN 查询:
import {
query,
collection,
where,
getDocs,
documentId,
} from 'firebase/firestore';
export async function fetchAccounts(
ids: string[]
) {
// use lodash _.chunk, for example
const result = await Promise.all(
chunk(ids, 10).map(async (chunkIds) => {
const accounts = await getDocs(
query(
collection(firestore, 'accounts'),
where(documentId(), 'in', chunkIds)
));
return accounts.docs.filter(doc => doc.exists()).map(doc => doc.data());
})
);
return result.flat(1);
}
对于一些陷入同样问题的人来说,这里是一个示例代码:
List<String> documentsIds = {your document ids};
FirebaseFirestore.getInstance().collection("collection_name")
.whereIn(FieldPath.documentId(), documentsIds).get().addOnCompleteListener(new OnCompleteListener<QuerySnapshot>() {
@Override
public void onComplete(@NonNull Task<QuerySnapshot> task) {
if (task.isSuccessful()) {
for (DocumentSnapshot document : Objects.requireNonNull(task.getResult())) {
YourClass object = document.toObject(YourClass.class);
// add to your custom list
}
}
}
}).addOnFailureListener(new OnFailureListener() {
@Override
public void onFailure(@NonNull Exception e) {
e.printStackTrace();
}
});
您可以使用“documentId()”和“in”和“where”子句在一次往返中通过多个id获取多个文档:
import {
query,
collection,
where,
documentId,
getDocs
} from "firebase/firestore";
const q = query(
collection(db, "products"),
where(documentId(), "in",
[
"7332ec75-c010-4316-af7f-51170769dca5",
"a1e820eb-7f8f-4761-ab00-708a7c836b7d",
"acd02b1d-2756-4205-b351-fc376b10ca7f"
]
),
);
const productDocsSnap = await getDocs(q);
productDocsSnap.forEach((doc) => {
console.log(doc.data()); // "doc1", "doc2" and "doc3"
});
如果您使用的是 python firebase 管理员 sdk 这就是您使用其 uid 查询多个文档的方式
from firebase_admin import firestore
import firebase_admin
from google.cloud.firestore_v1.field_path import FieldPath
app = firebase_admin.initialize_app(cred)
client = firestore.client(app)
collection_ref = client.collection('collection_name')
query = collection_ref.where(FieldPath.document_id(), 'in', listOfIds)
docs = query.get()
for doc in docs:
print(doc.id, doc.to_dict())
除了导入FieldPath
,您还可以简单地使用字符串__name__
。 现在您的查询将是collection_ref.where('__name__', 'in', listOfIds)
你能做的就是不能用最好的Promise.all
为你的客户则必须等待.all
在继续之前读取。
迭代读取并让它们独立解析。 在客户端,这可能归结为 UI 有几个进度加载器图像独立解析为值。 但是,这比冻结整个客户端直到.all
读取解决为止要好。
因此,立即将所有同步结果转储到视图中,然后让异步结果在解析时单独进入。 这似乎是微不足道的区别,但是如果您的客户的 Internet 连接不佳(就像我目前在这家咖啡店的情况),将整个客户体验冻结几秒钟可能会导致“这个应用程序很糟糕”的体验。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.