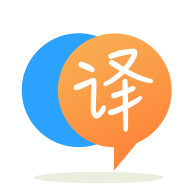
[英]Entity Framework - Code First - Ignore all properties except those specified
[英]Required rule for all properties in entity framework code first
我首先在 Entity Framework Core 中使用带有 fluent API 的代码来确定属性的行为。
我想知道是否有任何方法可以更换这部分。
modelBuilder.Entity<Person>()
.Property(b => b.Name)
.IsRequired();
modelBuilder.Entity<Person>()
.Property(b => b.LastName)
.IsRequired();
modelBuilder.Entity<Person>()
.Property(b => b.Age)
.IsRequired();
有这样的事情:
modelBuilder.Entity<Person>()
.AllProperties()
.IsRequired();
关键是有时大多数属性甚至所有属性都需要为 NOT NULL。 并且标记每个属性并不优雅。
解决方案可能是使用反射:
var properties = typeof(Class).GetProperties();
foreach (var prop in properties)
{
modelBuilder
.Entity<Class>()
.Property(prop.PropertyType, prop.Name)
.IsRequired();
}
请注意,所有属性都将根据需要进行设置。 当然,您可以根据类型(例如)过滤需要设置的属性。
更新
使用扩展方法可以使它更干净。
EntityTypeBuilderExtensions.cs
public static class EntityTypeBuilderExtensions
{
public static List<PropertyBuilder> AllProperties<T>(this EntityTypeBuilder<T> builder,
Func<PropertyInfo, bool> filter = null) where T : class
{
var properties = typeof(T)
.GetProperties()
.AsEnumerable();
if (filter != null)
{
properties = properties
.Where(filter);
}
return properties
.Select(x => builder.Property(x.PropertyType, x.Name))
.ToList();
}
}
在您的DbContext
用法:
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder
.Entity<Class>()
.AllProperties()
.ForEach(x => x.IsRequired());
}
如果您只想将IsRequired
应用于类的特定属性,您可以将过滤器函数传递给AllProperties
方法。
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
//Required is applied only for string properties
modelBuilder
.Entity<Class>()
.AllProperties(x => x.PropertyType == typeof(string))
.ForEach(x => x.IsRequired());
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.