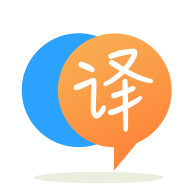
[英]Python how to sort list of objects based on their attribute according to another list
[英]How to efficiently sort a list of objects in python by attribute
可能会有一个标题相似的问题,但这无济于事。
我正在编写字典(英语,德语),现在的目标是按字母顺序对缓存(所有vocab对象的列表)进行排序。
vocab类的每个属性都是一个列表,其中列表中最重要的第一个元素/单词是列表,因此您使用该单词/单词进行排序。
这是一个工作最低版本:
class vocab:
def __init__(self, english, german, context, box=0):
""" self.eng/ger/con supposed to be lists"""
self.eng = english
self.ger = german
self.con = context
def present(self):
return "English: {}\n\nGerman: {}\n\nExample: {}\n{}\n".format(self.eng,self.ger,self.con,"-"*20)
#...
class dictionary:
def __init__(self, Cache=[]):
self.cache = Cache
def sort_cache(self, sortby="eng"):
"""sort cache alphabetically (default = english)"""
#list with sorted items
# -->item: (word used to sort, related vocObject)
sort_cache = sorted([(getattr(voc,sortby),voc) for voc in self.cache])
self.cache = [item[1] for item in sort_cache]
def show_cache(self):
""" print all vocabs from self.cache"""
out = ""
for voc in self.cache:
out += voc.present()
return out
#...
#e.g.
voc1 = vocab(["to run"],["rennen","laufen"],["Run Forest! Run!!"])
voc2 = vocab(["to hide"],["(sich) verstecken","(etw.) verbergen"],["R u hidin sth bro?"])
voc3 = vocab(["anything"],["irgendwas"],["Anything ding ding"])
voc4 = vocab(["example","instance","sample"],["Beispiel"],["sample"])
MAIN_DIC = dictionary([voc1,voc2,voc3,voc4])
print MAIN_DIC.show_cache() #-->prints vocabs in order: to run, to hide, anything, example
# (voc1), (voc2) , (voc3) , (voc4)
MAIN_DIC.sort_cache()
print MAIN_DIC.show_cache() #-->prints vocabs in wanted order: anything, example, to hide, to run
# (voc3) , (voc4) , (voc2) , (voc1)
由于我在sort_cache方法中创建了一个全新的缓存,因此我想知道哪种方法更有效。 我确定有一个。
例如。 我认为,仅对self.cache中的元素排序而不创建任何副本等会更有效(节省时间)。
这是“装饰-排序-取消装饰”模式:
sort_cache = sorted([(getattr(voc,sortby),voc) for voc in self.cache])
self.cache = [item[1] for item in sort_cache]
多年来,它一直是使用Python进行排序的首选方法。 它已由sort
和sorted
函数中的内置支持取代:
self.cache = sorted(self.cache, key=lambda item: getattr(item, sortby))
要么
self.cache.sort(key=lambda item: getattr(item, sortby))
您可能还需要考虑按已排序的顺序维护self.cache
(首先将其插入正确的位置-有关此问题,请参见bisect模块),从而分摊整个插入的排序成本(可能更多)整体费用较高,但任何单个操作的费用都较低。
另请注意:
def __init__(self, Cache=[]):
在使用此默认值的所有dictionary
实例中为您提供一个 共享的缓存列表。 可变的默认值通常不是您在Python中想要的。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.