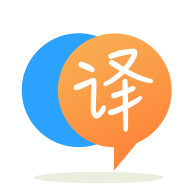
[英]How to make List in python dataclass that can accept multiple different types?
[英]How can I make my function accept different types of input?
我想创建一个接受输入(可以是int,数组或字符串形式)并返回其自恋数字的函数。
到目前为止,我创建了一个可以接受整数的函数,如果自恋则返回True,否则返回False。
def is_narcissistic(num):
total = []
number = len(str(num)) #Find the length of the value
power = int(number) #convert the length back to integer datatype
digits = [int(x) for x in str(num)] #convert value to string and loop through, convert back to int and store in digits
for i in digits:
product = i**power # iterate through the number and take each i to the power of the length of int
total.append(product) # append the result to the list total
totalsum = sum(total)
if totalsum == num:
print (True)
else:
print(False)
print(is_narcissistic(153))
但是,问题在于,它应该适用于任何类型的输入,例如字符串或列表。 例如:
输入:(0、1、2、3、4、5、6、7、8、9)---输出:True,“所有正的1位数整数都是自恋的”
输入:(0,1,2,3,4,5,6,7,22,9)---输出:False,“至少1个整数不是自恋的”
输入:(“ 4”)---输出:True,“数字可以,”
输入:(“单词”)---输出:False,“不是数字的字符串不行”)
那么我应该添加些什么,以便函数也可以接受这些输入?
自恋数字,例如153。因为当您将每个数字分开并乘以数字长度的幂(在这种情况下为3)时,您会得到数字本身。
1 ^ 3 + 5 ^ 3 + 3 ^ 3 = 153
您要做的就是检查以下特殊情况:
def is_narcissistic(num):
if isinstance(num, str): # is is a string?
if num.isdigit(): # does is consist purely out of numbers?
num = int(num)
else:
return False
if isinstance(num, (tuple,list)):
return all(is_narcissistic(v) for v in num)
# the rest of your code
另外,您还必须更改功能的结尾。 您不应该打印,但稍后返回使用该值:
if totalsum == num:
return True
else:
return False
如果希望不带元组的提取括号就可以调用它,则可以使用以下命令:
def is_narcissistic(*args):
if len(args) == 1:
num = args[0]
else:
num = args
# The code from above
现在您可以这样称呼它:
print(is_narcissistic(0, 1, 2, 3, 4, 5, 6, 7, 8, 9)) # True
print(is_narcissistic(0, 1, 2, 3, 4, 5, 6, 7, 22, 9)) # False
print(is_narcissistic(0, 1, "2", 3, "4", 5, 6, 7, "22", 9)) #False
完整的代码在这里:
def is_narcissistic(*args):
if len(args) == 1:
num = args[0]
else:
num = args
if isinstance(num, str):
if num.isdigit():
num = int(num)
else:
return False
if isinstance(num, (tuple, list)):
return all(is_narcissistic(v) for v in num)
total = []
number = len(str(num)) # Find the length of the value
power = int(number) # convert the length back to integer datatype
digits = [int(x) for x in str(num)] # convert value to string and loop through, convert back to int and store in digits
for i in digits:
product = i ** power # iterate through the number and take each i to the power of the length of int
total.append(product) # append the result to the list total
totalsum = sum(total)
if totalsum == num:
return True
else:
return False
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.