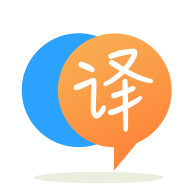
[英]Moving textfield when keyboard appears is covering up top textfields in (Swift)
[英]moving View up with textfield and Button when keyboard appear Swift
我知道有关于这个主题的一些问题,但没有一个有效。 我有一个tableview,在它下面有一个包含文本字段和按钮的UIView。 当我的键盘出现时,它覆盖了整个UIView,使我无法点击“发送”。 图像显示我的应用程序的外观:
目前,我的代码是:
override func viewDidLoad() {
super.viewDidLoad()
inputTextField.delegate = self
inputTextField.layer.cornerRadius = 5
inputTextField.clipsToBounds = true
sendButton.layer.cornerRadius = 5
sendButton.clipsToBounds = true
self.tableView.register(UINib(nibName: "TableViewCell", bundle: nil), forCellReuseIdentifier: "TableViewCell")
loadMsg()
self.hideKeyboardWhenTappedAround()
}
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
inputTextField.becomeFirstResponder()
}
当我的键盘出现时,如何将UIView与UIView包含的文本字段和按钮一起移动?
只需使用POD 将此库添加到您的项目中即可。 它会自动执行。 你不能做任何其他事情。
像这样添加pod
pod 'IQKeyboardManagerSwift'
在AppDelegate.swift中,只需导入IQKeyboardManagerSwift框架并启用IQKeyboardManager。
import IQKeyboardManagerSwift
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(application: UIApplication, didFinishLaunchingWithOptions launchOptions: [NSObject: AnyObject]?) -> Bool {
IQKeyboardManager.shared.enable = true
return true
}
}
如需参考,请查看此URL, https://github.com/hackiftekhar/IQKeyboardManager
这是解决方案,创建发送按钮视图的底部布局约束参考
@IBOutlet weak var bottomConstraint: NSLayoutConstraint!
@IBOutlet weak var sendbuttonView: UIView!
override func viewDidLoad() {
super.viewDidLoad()
NotificationCenter.default.addObserver(self, selector: #selector(handleKeyboardNotification), name: NSNotification.Name.UIKeyboardWillShow, object: nil)
NotificationCenter.default.addObserver(self, selector: #selector(handleKeyboardNotification), name: NSNotification.Name.UIKeyboardWillHide, object: nil)
}
override func viewWillDisappear(_ animated: Bool) {
NotificationCenter.default.removeObserver(self)
}
@objc func handleKeyboardNotification(_ notification: Notification) {
if let userInfo = notification.userInfo {
let keyboardFrame = (userInfo[UIKeyboardFrameEndUserInfoKey] as AnyObject).cgRectValue
let isKeyboardShowing = notification.name == NSNotification.Name.UIKeyboardWillShow
bottomConstraint?.constant = isKeyboardShowing ? -keyboardFrame!.height : 0
UIView.animate(withDuration: 0.5, animations: { () -> Void in
self.view.layoutIfNeeded()
})
}
}
请尝试以下代码
//Declare a delegate, assign your textField to the delegate and then include these methods
-(BOOL)textFieldShouldBeginEditing:(UITextField *)textField {
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(keyboardDidShow:) name:UIKeyboardDidShowNotification object:nil];
return YES;
}
- (BOOL)textFieldShouldEndEditing:(UITextField *)textField {
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(keyboardDidHide:) name:UIKeyboardDidHideNotification object:nil];
[self.view endEditing:YES];
return YES;
}
- (void)keyboardDidShow:(NSNotification *)notification
{
// Assign new frame to your view
[self.view setFrame:CGRectMake(0,-110,320,460)]; //here taken -110 for example i.e. your view will be scrolled to -110. change its value according to your requirement.
}
-(void)keyboardDidHide:(NSNotification *)notification
{
[self.view setFrame:CGRectMake(0,0,320,460)];
}
您可以通过移动向上查看constraint
来执行此操作actionBarPaddingBottomConstranit
是约束连接到您的输入视图Bottom
约束检查图像
在ViewDidload中:
override func viewDidLoad() {
super.viewDidLoad()
self.keybordControl()
}
延期 :
extension ViewController{
func keybordControl(){
NotificationCenter.default.addObserver(self, selector: #selector(self.keyboardWillShow), name: NSNotification.Name.UIKeyboardWillShow, object: nil)
NotificationCenter.default.addObserver(self, selector: #selector(self.keyboardWillHide), name: NSNotification.Name.UIKeyboardWillHide, object: nil)
}
@objc func keyboardWillShow(notification: Notification) {
self.keyboardControl(notification, isShowing: true)
}
@objc func keyboardWillHide(notification: Notification) {
self.keyboardControl(notification, isShowing: false)
}
private func keyboardControl(_ notification: Notification, isShowing: Bool) {
/* Handle the Keyboard property of Default, Text*/
var userInfo = notification.userInfo!
let keyboardRect = (userInfo[UIKeyboardFrameEndUserInfoKey]! as AnyObject).cgRectValue
let curve = (userInfo[UIKeyboardAnimationCurveUserInfoKey]! as AnyObject).uint32Value
let convertedFrame = self.view.convert(keyboardRect!, from: nil)
let heightOffset = self.view.bounds.size.height - convertedFrame.origin.y
let options = UIViewAnimationOptions(rawValue: UInt(curve!) << 16 | UIViewAnimationOptions.beginFromCurrentState.rawValue)
let duration = (userInfo[UIKeyboardAnimationDurationUserInfoKey]! as AnyObject).doubleValue
var pureheightOffset : CGFloat = -heightOffset
if isShowing {
if #available(iOS 11.0, *) {
pureheightOffset = pureheightOffset + view.safeAreaInsets.bottom
}
}
self.actionBarPaddingBottomConstranit?.update(offset:pureheightOffset)
UIView.animate(
withDuration: duration!,
delay: 0,
options: options,
animations: {
self.view.layoutIfNeeded()
},
completion: { bool in
})
}
}
Swift 4.2
在viewDidLoad()中
NotificationCenter.default.addObserver(self, selector: #selector(self.keyboard(notification:)), name: UIResponder.keyboardWillShowNotification, object: nil)
NotificationCenter.default.addObserver(self, selector: #selector(self.keyboard(notification:)), name: UIResponder.keyboardWillHideNotification, object: nil)
NotificationCenter.default.addObserver(self, selector: #selector(self.keyboard(notification:)), name:UIResponder.keyboardWillChangeFrameNotification, object: nil)
和方法
@objc func keyboard(notification:Notification) {
guard let keyboardReact = (notification.userInfo?[UIResponder.keyboardFrameEndUserInfoKey] as? NSValue)?.cgRectValue else{
return
}
if notification.name == UIResponder.keyboardWillShowNotification || notification.name == UIResponder.keyboardWillChangeFrameNotification {
self.view.frame.origin.y = -keyboardReact.height
}else{
self.view.frame.origin.y = 0
}
}
在ViewDidLoad中调用以下方法:
func keyboardSettings(){
NotificationCenter.default.addObserver(self, selector: #selector(self.keyboardNotification(notification:)), name: NSNotification.Name.UIKeyboardWillChangeFrame, object: nil)
self.view.addGestureRecognizer(UITapGestureRecognizer(target: self.view, action: #selector(UIView.endEditing(_:))))
let swipeDown = UISwipeGestureRecognizer(target: self.view , action : #selector(UIView.endEditing(_:)))
swipeDown.direction = .down
self.view.addGestureRecognizer(swipeDown)
}
然后为键盘配置添加以下方法:
@objc func keyboardNotification(notification: NSNotification) {
if let userInfo = notification.userInfo {
if let endFrame = (userInfo[UIKeyboardFrameEndUserInfoKey] as? NSValue)?.cgRectValue{
if let duration:TimeInterval = (userInfo[UIKeyboardAnimationDurationUserInfoKey] as? NSNumber)?.doubleValue{
let animationCurveRawNSN = userInfo[UIKeyboardAnimationCurveUserInfoKey] as? NSNumber
let animationCurveRaw = animationCurveRawNSN?.uintValue ?? UIViewAnimationOptions.curveEaseInOut.rawValue
let animationCurve:UIViewAnimationOptions = UIViewAnimationOptions(rawValue: animationCurveRaw)
if (endFrame.origin.y) >= UIScreen.main.bounds.size.height {
self.const_ViewMessage_Bottom?.constant = 0.0
} else {
self.const_ViewMessage_Bottom?.constant = endFrame.size.height
}
UIView.animate(withDuration: duration,
delay: TimeInterval(0),
options: animationCurve,
animations: { self.view.layoutIfNeeded() },
completion: nil)
}
}
}
}
self.const_ViewMessage_Bottom
是带文本字段的UIView的底部约束。
在一个ViewControllers中使用一个扩展名。 在文件中创建一个空的swift文件复制此代码
import UIKit
extension UIViewController {
func keyboardCheck() {
NotificationCenter.default.addObserver(self, selector: #selector(keyboardWillShow), name: UIResponder.keyboardWillShowNotification, object: nil)
NotificationCenter.default.addObserver(self, selector: #selector(keyboardWillHide), name: UIResponder.keyboardWillHideNotification, object: nil)
}
@objc func keyboardWillShow(notification: NSNotification) {
if let keyboardSize = (notification.userInfo?[UIResponder.keyboardFrameBeginUserInfoKey] as? NSValue)?.cgRectValue {
if self.view.frame.origin.y == 0 {
self.view.frame.origin.y -= keyboardSize.height
}
}
}
@objc func keyboardWillHide(notification: NSNotification) {
if self.view.frame.origin.y != 0 {
self.view.frame.origin.y = 0
}
}
}
然后调用ViewDidLoad用法中的函数:
override func viewDidLoad() {
super.viewDidLoad()
self.keyboardCheck()
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.